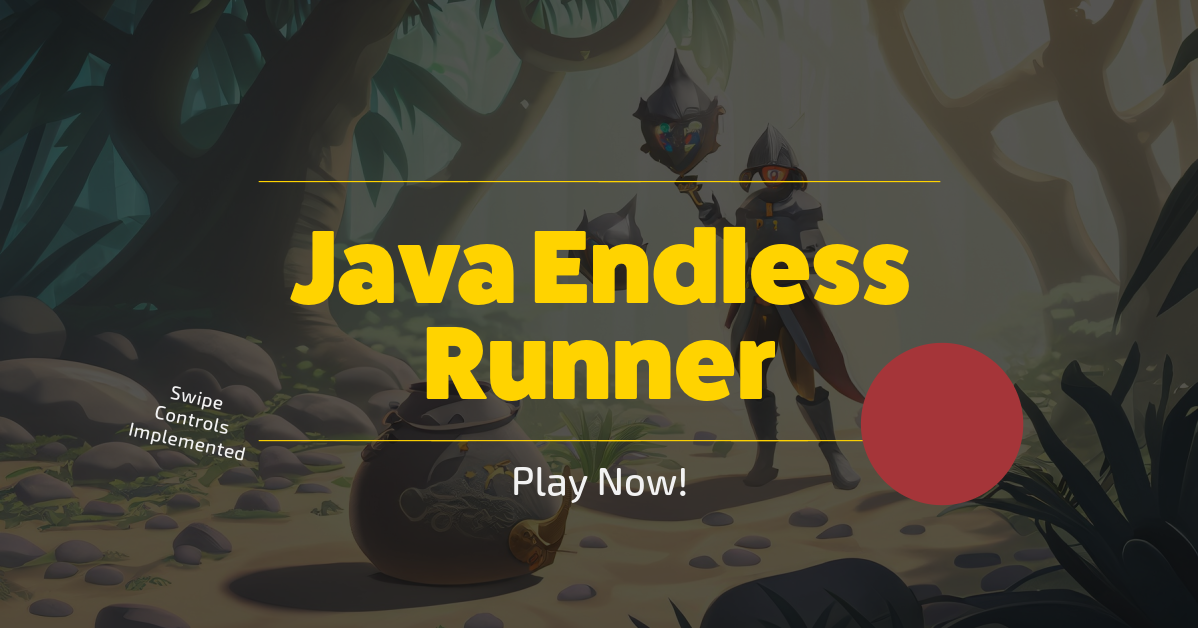
Creating an “Endless Runner” game involves a bit more complexity, especially when considering controls like swiping for movement. Since we’re focusing on web technologies, we’ll implement basic keyboard controls (left, right, and up for movement) to simplify the concept. Here’s how you can get started with HTML, CSS, and JavaScript.
Step 1: HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Endless Runner Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<div id="player"></div>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
#gameArea {
width: 800px;
height: 400px;
background-color: #f0f0f0;
overflow: hidden;
position: relative;
}
#player {
width: 50px;
height: 50px;
background-color: #000;
position: absolute;
bottom: 50px; /* Initial position */
left: 50px; /* Initial position */
}
Step 3: JavaScript Logic
For the JavaScript part, let’s set up the player movement and automatic running. We’ll focus on moving left and right and jumping (up). We won’t implement swiping since it’s more complex and requires touch event handling, but keyboard controls are a great starting point.
const player = document.getElementById('player');
let playerX = 50; // Initial X position
let playerY = 50; // Initial Y position (from bottom)
let isJumping = false;
document.addEventListener('keydown', (e) => {
switch (e.key) {
case 'ArrowLeft': // Move left
playerX -= 10;
break;
case 'ArrowRight': // Move right
playerX += 10;
break;
case 'ArrowUp': // Jump
if (!isJumping) {
isJumping = true;
jump();
}
break;
}
updatePlayerPosition();
});
function jump() {
let jumpHeight = 0;
let upInterval = setInterval(() => {
if (jumpHeight >= 100) {
clearInterval(upInterval);
let downInterval = setInterval(() => {
if (jumpHeight <= 0) {
clearInterval(downInterval);
isJumping = false;
} else {
jumpHeight -= 5;
playerY = 50 + jumpHeight;
updatePlayerPosition();
}
}, 20);
} else {
jumpHeight += 5;
playerY = 50 + jumpHeight;
updatePlayerPosition();
}
}, 20);
}
function updatePlayerPosition() {
player.style.left = playerX + 'px';
player.style.bottom = playerY + 'px';
}
Summary
- The player’s character runs automatically, but since our simple version focuses on basic controls, you might consider implementing an automatic forward movement or obstacles coming towards the player.
- The
keydown
event listener checks for arrow key presses to move the player left, right, or make them jump. - The
jump
function simulates a jumping motion with intervals, similar to the previous example. updatePlayerPosition
updates the CSS position of the player based on their X and Y coordinates.
Enhancements
- Automatic Running: You could simulate automatic running by moving the background or obstacles towards the player.
- Obstacles and Power-ups: Dynamically generate obstacles and power-ups that the player must avoid or collect.
- Score and Difficulty: Implement a scoring system based on distance run and gradually increase the difficulty by speeding up the game or adding more obstacles.
This setup provides a basic framework. Expanding it into a full-fledged endless runner game would involve adding more complex mechanics, such as collision detection, scoring, and increasingly difficult obstacles.
Key Variables
const player = document.getElementById('player');
let playerX = 50; // Initial X position
let playerY = 50; // Initial Y position (from bottom)
let isJumping = false;
player
: Represents the player’s character in the game, allowing us to modify its position on the screen.playerX
andplayerY
: Hold the current position of the player.playerX
is the horizontal position, andplayerY
is the vertical position from the bottom of the game area.isJumping
: A boolean flag used to indicate whether the player is currently jumping. This prevents the player from initiating a new jump mid-air.
Responding to Key Presses
document.addEventListener('keydown', (e) => {
switch (e.key) {
case 'ArrowLeft': // Move left
playerX -= 10;
break;
case 'ArrowRight': // Move right
playerX += 10;
break;
case 'ArrowUp': // Jump
if (!isJumping) {
isJumping = true;
jump();
}
break;
}
updatePlayerPosition();
});
- An event listener is attached to the entire document, listening for when a key is pressed down.
- When an arrow key is pressed, the code checks which key (
ArrowLeft
,ArrowRight
,ArrowUp
) and performs an action:- Left/Right: Decreases/increases the
playerX
value, moving the player horizontally. - Up: Initiates a jump if the player is not already jumping.
- Left/Right: Decreases/increases the
- After any key press,
updatePlayerPosition()
is called to apply the new position changes to the player’s character.
The Jump Mechanism
function jump() {
let jumpHeight = 0;
let upInterval = setInterval(() => {
...
}, 20);
}
- The
jump
function controls the character’s jumping motion using two intervals: one for moving up and another for coming down. jumpHeight
tracks how high the player has jumped from the initial position.
Moving Up
- The character moves up by increasing
jumpHeight
and thusplayerY
, simulating the jump. This continues until a certain height is reached (jumpHeight >= 100
).
Coming Down
- After reaching the peak of the jump, another interval decreases
jumpHeight
, simulating gravity pulling the player back down.
Updating Player Position
function updatePlayerPosition() {
player.style.left = playerX + 'px';
player.style.bottom = playerY + 'px';
}
- This function updates the CSS properties of the player element to reflect the new position (
playerX
andplayerY
) on the screen. - It’s called after every movement (left/right/jump) to visually move the player in the game area.
Summary for Students
This code allows a character in an endless runner game to move left, right, and jump using the keyboard’s arrow keys. The movement is simulated by changing the position of the player character on the web page, using CSS properties manipulated through JavaScript. The jumping action is created by a timed sequence of movements that simulate an arc, with gravity bringing the player back down after reaching a peak height. This example provides a basic foundation for understanding how player input can be captured and translated into character movement in web-based games.
Reference Links to Include:
Java Game Development Frameworks:
- For insights on using Java frameworks like LibGDX for game development.
- Suggested Search: “LibGDX Java game development tutorial”
Game Design Principles:
- To provide foundational knowledge on crafting engaging game mechanics, focusing on endless runner games.
- Suggested Search: “Game design principles for endless runners”
Implementing Swipe Controls in Java:
- For tutorials on creating intuitive swipe controls for mobile games developed in Java.
- Suggested Search: “Java swipe control implementation for games”
Stack Overflow for Game Development Queries:
- A resource for troubleshooting and advice on developing endless runner games in Java.
Leave a Reply