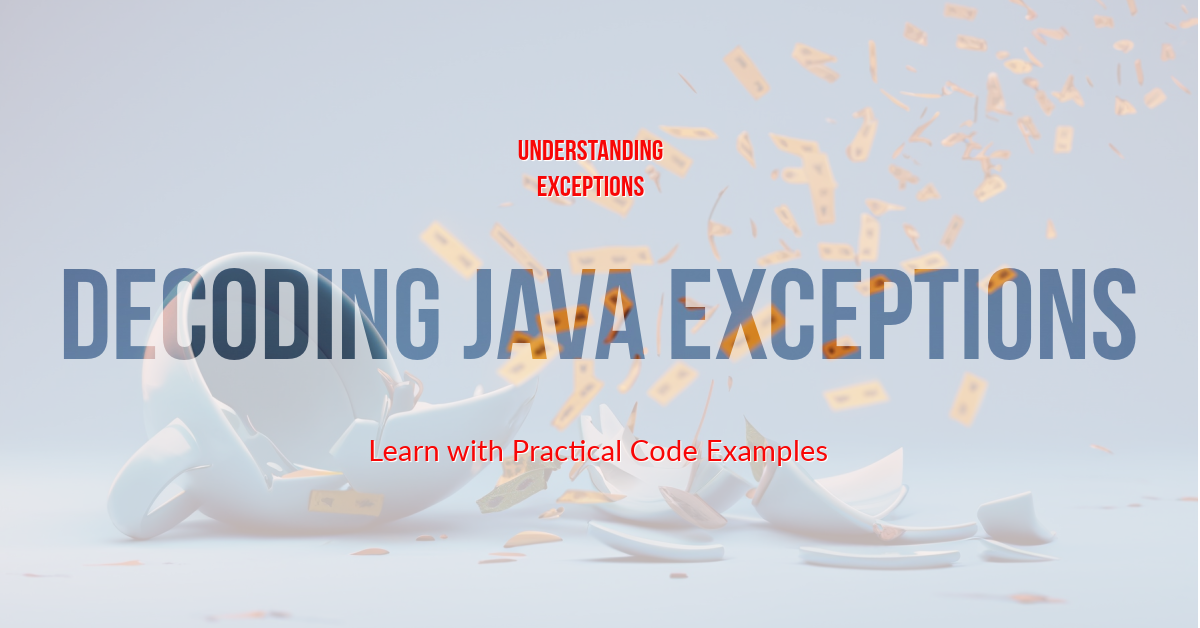
A Java exception occurs when an error occurs during the execution of a program, interrupting the normal flow of instructions. Exceptions in Java are objects that describe errors or unexpected events during program execution. It provides a robust mechanism to catch and handle these exceptions using try-catch blocks or by declaring them using the throws keyword in Java. Exceptions can be caught and handled via try-catch blocks and throws rules, respectively.
An example of a common Java exception
An exception might occur if we try to access an array index that does not exist. This results in an ArrayIndexOutOfBoundsException.
public class ArrayExceptionExample {
public static void main(String[] args) {
try {
int[] numbers = {1, 2, 3};
// Attempting to access the 4th element of the array, which does not exist
System.out.println(numbers[3]);
} catch (ArrayIndexOutOfBoundsException e) {
System.out.println("An exception has occurred: " + e.getMessage());
}
}
}
In this example,
The array of numbers has three elements.
The code attempts to access the element at index 3, which does not exist (arrays are zero-indexed, so valid indices are 0, 1, and 2).
This access attempt throws an ArrayIndexOutOfBoundsException.
The try-catch block catches this exception, and the catch block executes, printing a message that an exception has occurred.
Exception handling in Java
Java provides two primary ways to handle exceptions:
Try-Catch Block: Allows you to catch exceptions and manage them. The try block contains code that might throw an exception, and the catch block contains code to execute if an exception occurs.
Throw Keyword: Used in a method signature to declare that the method might throw exceptions. The responsibility for handling these exceptions is passed to the method caller.
Example of Declaring an Exception
public class ThrowsExample {
public static void main(String[] args) {
try {
riskyMethod();
} catch (Exception e) {
System.out.println("Exception handled: " + e.getMessage());
}
}
public static void riskyMethod() throws Exception {
throw new Exception("A deliberate exception");
}
}
In this example,
riskyMethod is declared with the throws keyword, indicating it might throw an Exception.
Inside riskyMethod, an Exception is explicitly thrown with a custom message.
The primary method calls riskyMethod within a try-catch block, thus handling the exception that riskyMethod might throw.
A fundamental part of Java is exceptions. They make your program more resilient to unexpected situations by managing runtime errors.
Leave a Reply