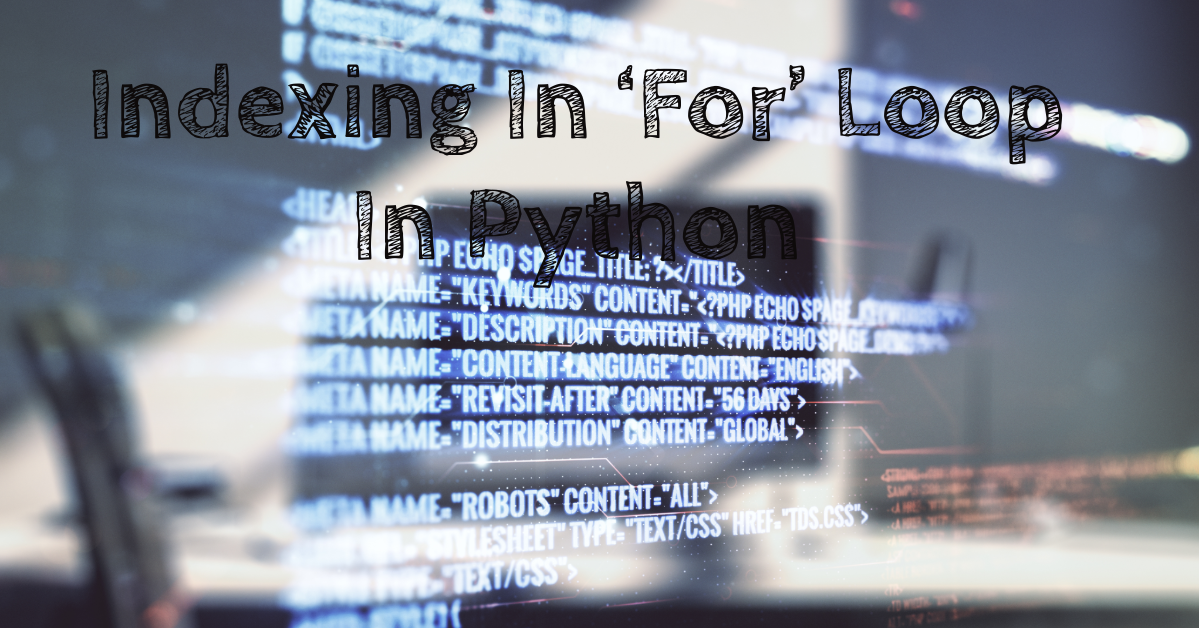
o access the index value in a Python for loop, use the enumerate() function. Enumerate () adds a counter to an iterable and returns it as an enumerated object. This can be directly used for loops to get the index and value of each item in the iterable.
Here’s a basic example.
# List of items
items = ['apple', 'banana', 'cherry']
# Using enumerate() to access index and value
for index, value in enumerate(items):
print(f"Index: {index}, Value: {value}")
Output:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
In this example, enumerate(items) returns a tuple for each item in the list, where the first element is the index and the second element is the value. The for loop unpacks each tuple into index and value variables, which you can use within the loop.
Customizing the Start Index
By default, enumerate() starts counting from 0, but you can specify a different start value by providing a second argument to enumerate(). For example, if you want the index to start at 1:
items = ['apple', 'banana', 'cherry']
for index, value in enumerate(items, start=1):
print(f"Index: {index}, Value: {value}")
Output:
Index: 1, Value: apple
Index: 2, Value: banana
Index: 3, Value: cherry
It is beneficial when dealing with situations where the index needs to match human-friendly counting (starting from 1) or when an external requirement defines the starting index.
Reference Links:
- W3Schools Python ‘for’ loop Tutorial
Leave a Reply