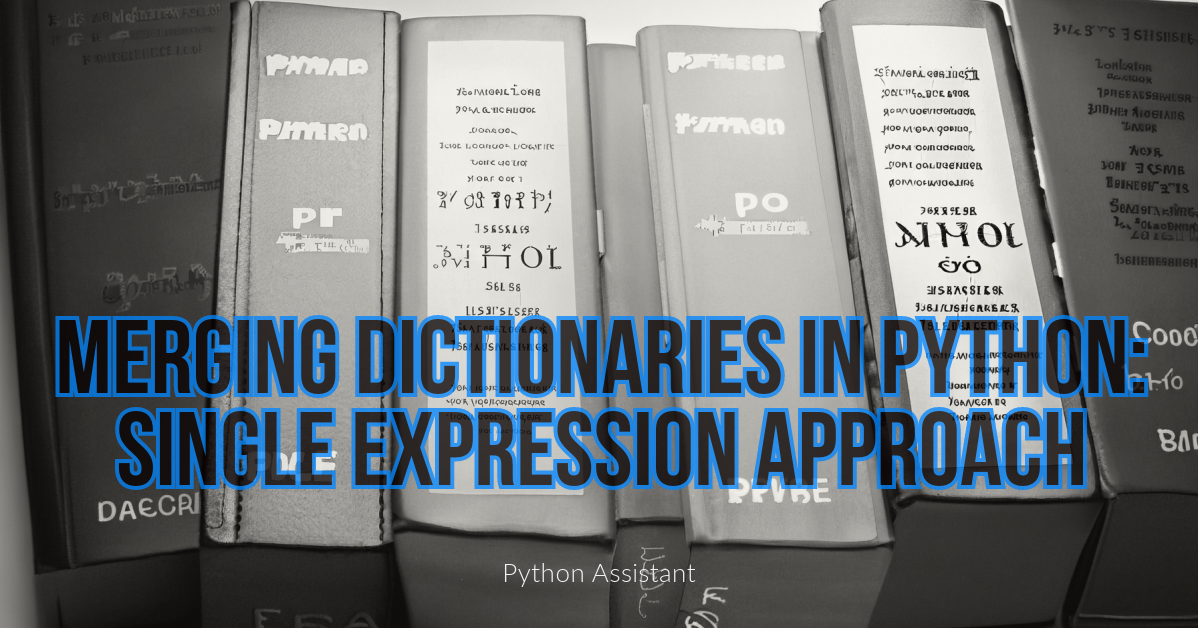
In Python, you can merge two dictionaries into a single dictionary using several methods, depending on your Python version.
For Python 3.5+:
You can use the {**d1, **d2} syntax, where d1 and d2 are the dictionaries you want to merge. This method creates a newly created dictionary containing the keys and values from D1 and D2. If there are overlapping keys, d2 values will overwrite d1 values.
d1 = {'a': 1, 'b': 2}
d2 = {'b': 3, 'c': 4}
merged_dict = {**d1, **d2}
print(merged_dict)
Output:
{'a': 1, 'b': 3, 'c': 4}
For Python 3.9+:
Python 3.9 introduced a more intuitive way to merge dictionaries using the | operator. Similar to the method above, this merges d1 and d2, with values from d2 overwriting those from d1 for matching keys.
d1 = {'a': 1, 'b': 2}
d2 = {'b': 3, 'c': 4}
merged_dict = d1 | d2
print(merged_dict)
Output:
{'a': 1, 'b': 3, 'c': 4}
Updating an Existing Dictionary (All Python Versions):
If you want to update d1 instead of d2 contents (modifying d1), you can use the .update() method:
d1 = {'a': 1, 'b': 2}
d2 = {'b': 3, 'c': 4}
d1.update(d2)
print(d1)
Output:
{'a': 1, 'b': 3, 'c': 4}
This method modifies d1 to include items from d2, overwriting existing keys in d1 with those from d2.
Each of these methods has its use cases, depending on whether you need a newly merged dictionary or want to update an existing dictionary. In addition, it depends on which Python version you use.
Reference Links:
- Stack Overflow Discussion (Merging Dictionaries)
Leave a Reply