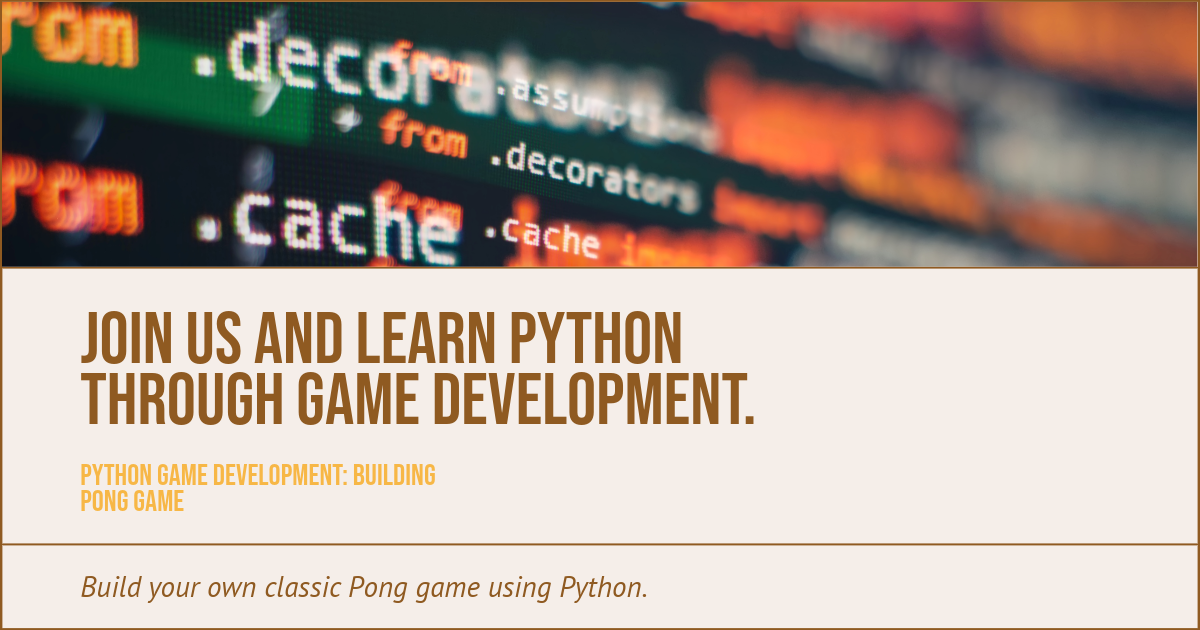
Pong Game Plan:
Language: We will use Python, a popular choice for game developers.
Library: We will use pygame, a Python module for writing video games.
Game elements:
Play is controlled by two paddles, one by the player and one by the computer.
A ball that bounces between the paddles.
Scoring system to keep track of points.
Features:
Movement of paddles using keyboard inputs for the player.
A simple AI that follows the ball using a computer paddle.
Collision detection between the ball and paddles.
Ball speed increases with each hit for added difficulty.
User interface:
It’s a game window.
The score is displayed on the screen.
Pong Game Code :
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants for the game
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
PADDLE_WIDTH, PADDLE_HEIGHT = 15, 90
BALL_RADIUS = 10
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
# Setting up the screen
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Pong Game')
# Paddle and Ball settings
player_paddle = pygame.Rect(50, SCREEN_HEIGHT // 2 - PADDLE_HEIGHT // 2, PADDLE_WIDTH, PADDLE_HEIGHT)
computer_paddle = pygame.Rect(SCREEN_WIDTH - 50 - PADDLE_WIDTH, SCREEN_HEIGHT // 2 - PADDLE_HEIGHT // 2, PADDLE_WIDTH, PADDLE_HEIGHT)
ball = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2, BALL_RADIUS, BALL_RADIUS)
# Ball movement
ball_speed_x = 7 * random.choice((1, -1))
ball_speed_y = 7 * random.choice((1, -1))
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Move the ball
ball.x += ball_speed_x
ball.y += ball_speed_y
# Ball collision with top and bottom
if ball.top <= 0 or ball.bottom >= SCREEN_HEIGHT:
ball_speed_y *= -1
# Ball collision with paddles
if ball.colliderect(player_paddle) or ball.colliderect(computer_paddle):
ball_speed_x *= -1
# Player paddle movement
keys = pygame.key.get_pressed()
if keys[pygame.K_UP] and player_paddle.top > 0:
player_paddle.y -= 10
if keys[pygame.K_DOWN] and player_paddle.bottom < SCREEN_HEIGHT:
player_paddle.y += 10
# Computer paddle AI
if computer_paddle.top < ball.y:
computer_paddle.y += 7
elif computer_paddle.bottom > ball.y:
computer_paddle.y -= 7
# Draw everything
screen.fill(BLACK)
pygame.draw.rect(screen, WHITE, player_paddle)
pygame.draw.rect(screen, WHITE, computer_paddle)
pygame.draw.ellipse(screen, WHITE, ball)
pygame.draw.aaline(screen, WHITE, (SCREEN_WIDTH // 2, 0), (SCREEN_WIDTH // 2, SCREEN_HEIGHT))
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if you haven’t already: pip install pygame.
Save this script as a .py file.
Run the script, and you will see the Pong game window.
This code provides a basic Pong game with a player paddle controlled by up and down arrow keys. A simple AI is also provided for the computer paddle in this program. The ball increases in speed with each paddle hit, adding challenge as the game progresses.
Pong game code into understandable parts:
Importing the library:
import pygame
import random
Pygame is a Python library used for games.
Random generates random values, which we use to decide the ball’s initial direction.
Initializing Pygame and Setting Up Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
PADDLE_WIDTH, PADDLE_HEIGHT = 15, 90
BALL_RADIO = 10
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
Pygame. Init () starts all Pygame modules.
SCREEN_WIDTH and SCREEN_HEIGHT set the game window size.
PADDLE_WIDTH and PADDLE_HEIGHT define the size of the paddles.
BALL_RADIUS is the ball size.
WHITE and BLACK are color definitions in RGB format.
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Pong Game’)
This sets up the game window with the specified width and height.
The caption sets the window title.
Setting Up Paddles and Ball
Player_paddle = pygame.Rect(50, SCREEN_HEIGHT // 2 – PADDLE_HEIGHT // 2, PADDLE_WIDTH, PADDLE_HEIGHT)
Computer_paddle = pygame.Rect(SCREEN_WIDTH – 50 – PADDLE_WIDTH, SCREEN_HEIGHT // 2 – PADDLE_HEIGHT // 2, PADDLE_WIDTH, PADDLE_HEIGHT)
The ball is calculated as follows: pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2, BALL_RADIUS, BALL_RADIUS)
Player_paddle and computer_paddle are rectangles representing the player’s and computer’s paddles.
A ball is a square representing a ball.
Ball movement initialization
Ball_speed_x = 7 * random.choice((1, -1))
Ball_speed_y = 7 * random.choice((1, -1))
These lines set the ball’s initial speed and direction. The direction is random (left or right, up or down).
The Game Loop
While running:
# Handle events …
# Move the ball …
# Ball collision with top and bottom …
# Ball collision with paddles …
# Player paddle movement …
# Computer paddle AI …
# Draw everything…
# Update the display…
# Ticking the clock …
The game loop is where the game logic happens. It continues as long as running is taking place.
Event handling
For Pygame events. Event.get():
If event.type == pygame.QUIT:
I am running = false.
This checks for events like closing the game window.
Moving the ball
Ball.x += ball_speed_x
Ball. y += ball
Changes the ball’s position based on its speed.
Collision detection
If ball. Top <= 0 or ball.bottom >= SCREEN_HEIGHT:
Ball_speed_y * = -1
Changes the ball’s direction when it hits the top or bottom of the screen.
Paddle Movement and Computer AI
# Player controls and Computer AI logic here
Player controls move a paddle up and down.
The computer AI moves its paddle to follow the ball.
Drawing on the screen
# Drawing the paddles, the ball, and the midline
Draw the paddles, the ball, and a line in the middle of the screen.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(60)
Pygame. Display. Flip () updates the entire screen with what we’ve drawn.
Clock. Tick (60) ensures the game runs at 60 frames per second.
It is exciting to play the game.
Pygame.quit()
Closes the playgame window and exits the game.
This code creates a simple yet functional Pong game, demonstrating basic game development concepts such as game loops, event handling, drawing graphics, and collision detection.
References:
Python Official Documentation:
- URL: https://www.python.org/doc/
- Why include it: It’s the official source for Python programming language documentation, offering comprehensive guides and tutorials for Python developers of all levels.
Pygame Official Documentation:
- URL: https://www.pygame.org/docs/
- Why include it: If your tutorial uses Pygame for developing the Pong game, this is an essential resource for readers to understand and implement Pygame functionalities.
GitHub Repository for Example Python Games:
- URL: https://github.com/topics/python-game
- Why include it: Provides examples of Python games developed by the community, including potentially Pong game projects, which can offer practical insights and inspiration.
Codecademy’s Python Course:
- URL: https://www.codecademy.com/learn/learn-python-3
- Why include it: Great for readers who are beginners in Python and might benefit from a structured course to complement your tutorial.
FreeCodeCamp’s Game Development with Python Tutorial:
- URL: https://www.freecodecamp.org/news/python-game-development-tutorial/
- Why include it: Offers a broad look at game development with Python, including tutorials that might cover Pong or similar projects, providing a different learning perspective.
Leave a Reply