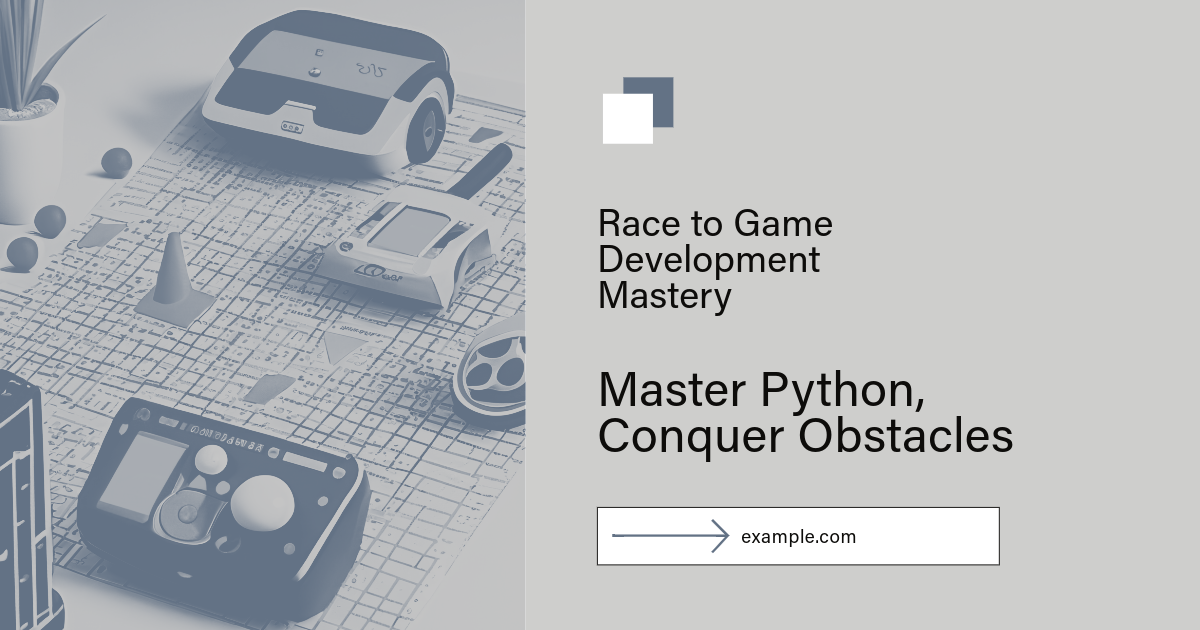
Racing Game Plan:
Language: Python, widely used for its simplicity and readability, is suitable for game development beginners.
Library: pygame for handling graphics and events.
Game elements:
A vehicle (car) that the player controls.
A straight track with lane boundaries.
Obstacles to avoid.
Features:
Basic controls to move the vehicle left and right.
The car moves forward.
Randomly spawn barriers that the player must avoid.
Scoring is based on distance traveled or obstacles avoided.
User interface:
A window showing the car, the track, and obstacles.
Display the player’s score or distance.
Racing Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
CAR_WIDTH, CAR_HEIGHT = 50, 100
OBSTACLE_WIDTH, OBSTACLE_HEIGHT = 50, 50
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Racing Game')
# Car setup
car = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT - 120, CAR_WIDTH, CAR_HEIGHT)
# Obstacles setup
obstacles = []
obstacle_frequency = 1500 # milliseconds
last_obstacle = pygame.time.get_ticks()
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Car movement
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and car.left > 0:
car.move_ip(-5, 0)
if keys[pygame.K_RIGHT] and car.right < SCREEN_WIDTH:
car.move_ip(5, 0)
# Generate obstacles
current_time = pygame.time.get_ticks()
if current_time - last_obstacle > obstacle_frequency:
obstacle_x = random.randint(0, SCREEN_WIDTH - OBSTACLE_WIDTH)
obstacles.append(pygame.Rect(obstacle_x, -OBSTACLE_HEIGHT, OBSTACLE_WIDTH, OBSTACLE_HEIGHT))
last_obstacle = current_time
# Move obstacles
for obstacle in obstacles[:]:
obstacle.move_ip(0, 5)
if obstacle.top > SCREEN_HEIGHT:
obstacles.remove(obstacle)
# Collision detection
for obstacle in obstacles:
if car.colliderect(obstacle):
running = False
# Drawing everything
screen.fill(WHITE)
pygame.draw.rect(screen, BLUE, car)
for obstacle in obstacles:
pygame.draw.rect(screen, RED, obstacle)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the racing game will start.
In this code, the player controls a car, moving left and right to avoid random obstacles on the screen. The game ends if the car collides with an obstacle.
Importing libraries
Import a pygame
Import random
pygame creates the game, manages graphics, and handles user inputs.
Randomly generates random obstacle positions.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Other constants for car size, obstacle size, and colors
Initializes Pygame, which is necessary for game development.
Set constants for the screen size, car size, obstacle size, and colors used in the game.
Creating the game window
Screen = pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame. Display.set_caption(‘Racing Game’)
Sets up the game window with the specified width and height.
Set the window title to ‘Racing Game.’
Setting Up the Car and Obstacles
Car = Pygame.Rect(…)
Obstacles = []
The car is a rectangle representing the player’s car.
The obstacle is a list that stores rectangles for each obstacle.
Game loop
While running:
# Event handling, car movement, obstacle generation, and collision detection
The main game loop continues as long as running is True.
It handles critical events, updates the game state, and redraws the graphics for each iteration.
Handling Player Input and Car Movement
Key = pygame. Key.get_pressed()
# Code to move the car left or right
Check if the left or right arrow key is pressed and move the car accordingly.
Generating and Moving Obstacles
# Code to generate upcoming obstacles periodically
# Code to move each obstacle down the screen
Creates new obstacles randomly.
Move each obstacle down the screen to simulate the car moving forward.
Collision detection
For obstacles in obstacles:
If car.collider(obstacle):
Running = false.
Check if the car collides with any obstacle and end the game if a collision occurs.
Drawing of the car and obstacles.
Screen.fill(WHITE)
Code drawing of the car and obstacles
He clears the screen before drawing the car and obstacles.
Update the display and frame rate.
pygame. Display.flip()
Clock.tick(60)
Updates the entire screen with what has been drawn.
Run the game at 60 frames per second to ensure smooth movement.
It is exciting to play the game.
pygame.quit()
It closes the game window and ends the game.
Learning Points for Students
Game Loop: Understanding the main loop that handles events, updates game state, and renders graphics.
Player Input: How to respond to key presses to control the game character (car).
Collision Detection: Checking for interactions between the vehicle and obstacles.
Lists and Loops: Manage multiple game objects (obstacles) using lists and loops.
Reference Links to Include:
Python Official Documentation:
- Purpose: To provide foundational Python syntax and library information.
Pygame Documentation (for game graphics and interaction):
- Purpose: Essential for developing the game’s graphical interface and handling user input.
GitHub Projects for Racing Games in Python:
- Purpose: To offer coding inspiration and insights from existing racing games developed in Python.
Stack Overflow for Python Game Development Advice:
- Purpose: A valuable resource for troubleshooting and getting advice on game development challenges with Python.
Leave a Reply