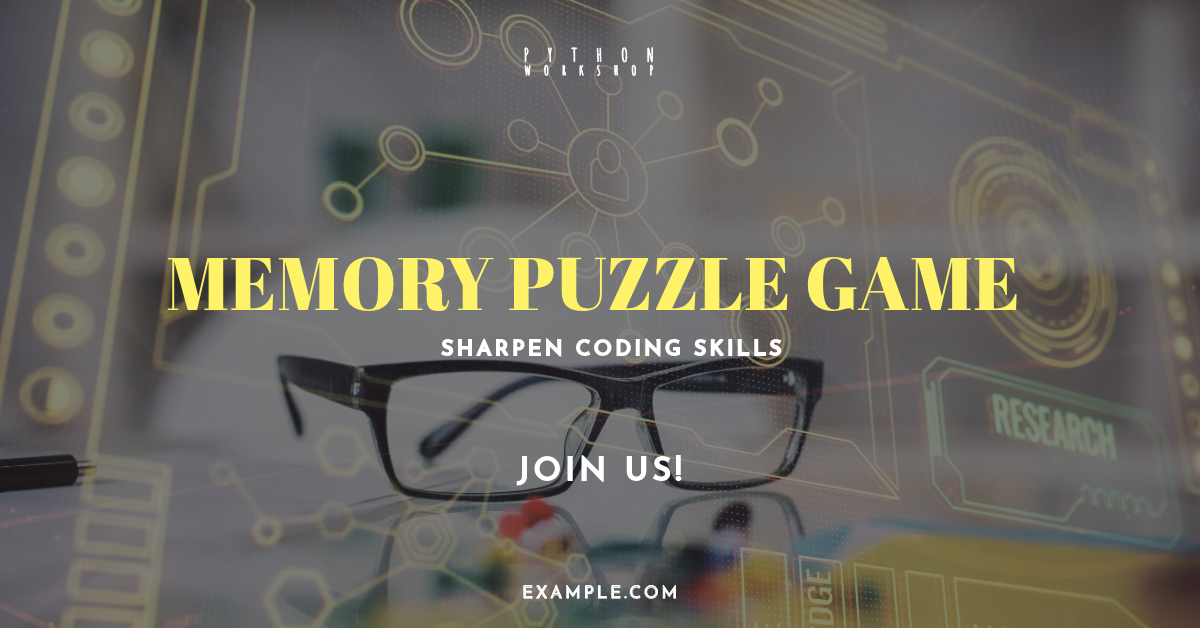
Memory Puzzle Game Plan:
Language: Python is ideal for creating simple games and learning basic programming concepts.
Library: pygame, used to handle graphics, events, and game states.
Game elements:
A grid of flippable tiles.
Pairs of identical images are hidden under these tiles.
Features:
Players can click and flip tiles.
Checking for matches when two tiles are flipped.
Keeping track of attempts and matches.
Resetting unmatched tiles after a short delay.
User interface:
A tile grid window.
Optional: Display of attempts or scores.
Memory Puzzle Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 600
TILE_SIZE = 100
GRID_SIZE = 4
MARGIN = 10
WHITE = (255, 255, 255)
GRAY = (200, 200, 200)
BLUE = (0, 0, 255)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Memory Puzzle Game')
# Generate pairs of numbers
numbers = list(range(1, GRID_SIZE * GRID_SIZE // 2 + 1)) * 2
random.shuffle(numbers)
# Setup tiles
tiles = [pygame.Rect(x * (TILE_SIZE + MARGIN) + MARGIN, y * (TILE_SIZE + MARGIN) + MARGIN, TILE_SIZE, TILE_SIZE)
for x in range(GRID_SIZE) for y in range(GRID_SIZE)]
flipped_tiles = []
matched_tiles = []
waiting = False
clock = pygame.time.Clock()
# Game loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEBUTTONDOWN and not waiting:
mouse_pos = event.pos
for i, tile in enumerate(tiles):
if tile.collidepoint(mouse_pos) and i not in matched_tiles:
flipped_tiles.append(i)
if len(flipped_tiles) == 2:
waiting = True
# Check for matches
if waiting and not pygame.time.get_ticks() % 1000:
index1, index2 = flipped_tiles
if numbers[index1] == numbers[index2]:
matched_tiles.extend(flipped_tiles)
flipped_tiles = []
waiting = False
# Draw everything
screen.fill(WHITE)
for i, tile in enumerate(tiles):
if i in matched_tiles or i in flipped_tiles:
pygame.draw.rect(screen, BLUE, tile)
font = pygame.font.Font(None, 40)
text = font.render(str(numbers[i]), True, WHITE)
text_rect = text.get_rect(center=tile.center)
screen.blit(text, text_rect)
else:
pygame.draw.rect(screen, GRAY, tile)
pygame.display.flip()
clock.tick(30)
pygame.quit()
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut elit tellus, lu
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Memory Puzzle game will start.
This code provides a basic memory puzzle game where players flip tiles to find matching pairs of numbers. The game includes a simple delay mechanism to allow players to view the numbers before the tiles flip back if they don’t match.
Importing libraries
Import a pygame
Import random
Pygame creates to create, handles events, handles raw graphics drawn, and shuffles the numbers for the tiles.
Initializing Pygame and Setting Constants
pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 600
# Other constants for tile size, grid size, margin, and colors
Initialize the Pygame module.
Set up constants for the game’s screen size, tile size, grid size, margins, and colors.
Creating the game window
Screen = pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame. Display.set_caption(‘Memory Puzzle Game’)
Creates a game window with the specified dimensions.
Sets the window title.
Generating Pairs of Numbers and Setting Up Tiles
Numbers = list(range(1, GRID_SIZE GRID_SIZE // 2 + 1)) 2
Random.shuffle(numbers)
Tiles = […]
Creates a list of numbers for the tiles, with each number appearing twice.
Shuffle the numbers to randomize the tile arrangement.
Creates a list of pygame—rect objects representing each tile’s position and size.
Game variables
Flipped_tiles = []
Matched_tiles = []
Waiting = false.
Clock = pygame. Time.Clock()
Flipped_tiles keeps track of the indices of tiles currently flipped.
Matched_tiles stores the indices of tiles that have been successfully matched.
Waiting introduces a delay after flipping two tiles.
The clock controls the game’s frame rate.
The Game Loop
While running:
# Event handling, flipping tiles, checking for matches…
The main game loop handles events (like mouse clicks), updates the game state, and redraws the screen.
Handling mouse clicks and Flipping Tiles
For the event in pygame. Event.get():
If event.type == pygame.MOUSEBUTTONDOWN and not waiting:
# Code to flip tiles
Listens for mouse clicks and flips the corresponding tile if it’s not matched or flipped.
I checked for matches.
If waiting and not pygame.time.get_ticks() % 1000:
# Code to check if two flipped tiles match
Introduces a delay after flipping two tiles, then checks if they match. If they match, they remain reversed; otherwise, they flip back.
Drawing of tiles and numbers
For i, tile in enumerate(tiles):
# Code to draw tiles and numbers
Draw each tile on the screen.
If a tile is flipped or matched, its number is displayed.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(30)
Updates the entire screen with what has been drawn.
Set the frame rate to 30.
It is exciting to play the game.
Pygame.quit()
It closes the game window and ends the game.
Learning Points for Students
Event Handling: How to respond to user inputs like mouse clicks.
List Manipulation: Managing lists of tiles, numbers, and matches.
Conditional Logic: Using conditions to determine game actions, like flipping tiles or checking for matches.
Rendering Graphics: Drawing shapes and text on the screen to create a game interface.
Reference Links to Include:
Python Official Documentation:
- Purpose: Provides essential Python syntax and library information for beginners and advanced developers.
Pygame Documentation (for game development):
- Purpose: Vital for readers looking to add interactive elements and graphics to their memory puzzle game.
GitHub Projects for Memory Games in Python:
- Purpose: Offers inspiration and practical coding examples from existing Python projects focused on memory games.
Stack Overflow for Python Game Development:
- Purpose: A great resource for solving common programming challenges and gaining insights from the Python game development community.
Leave a Reply