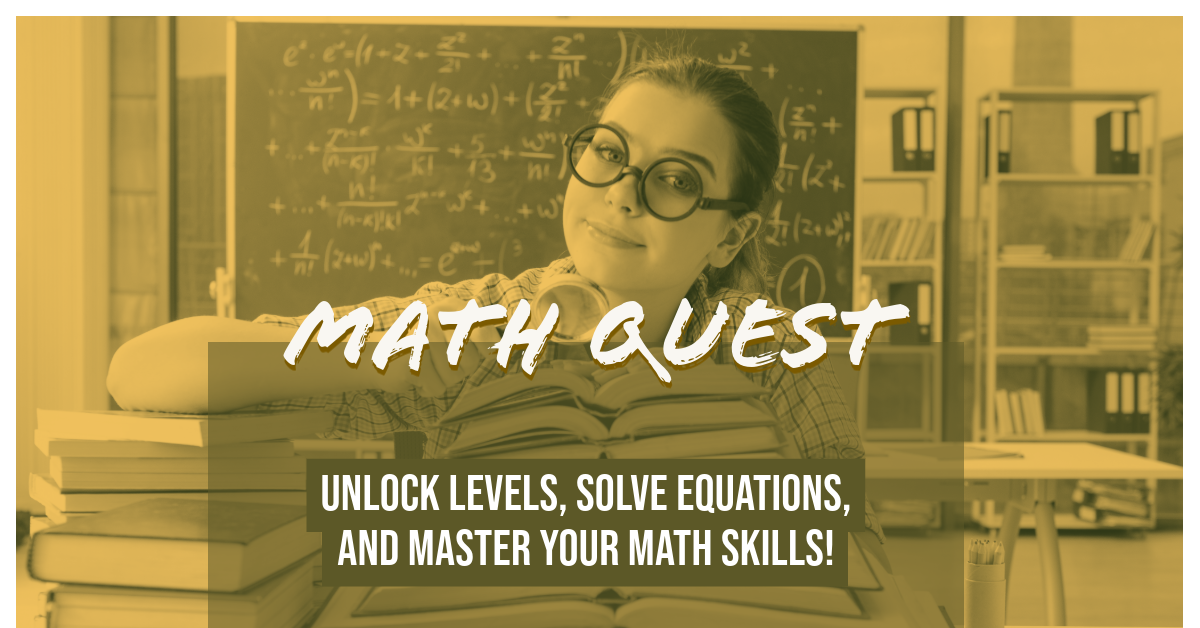
To create a Math Puzzle Game, you need to design challenges in which players solve math problems or equations. This explanation outlines how to build such a game using HTML for the structure, CSS for the styling, and JavaScript for the game’s logic and interactivity.
Step 1: HTML Structure
Define the main components: a display for the math problem, an input for the player’s answer, a submit button, and placeholders for level and score.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Math Puzzle Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameContainer">
<div id="problem">Solve: 3 + 4</div>
<input type="text" id="playerAnswer" placeholder="Enter your answer">
<button id="submitAnswer">Submit</button>
<div id="level">Level: 1</div>
<div id="score">Score: 0</div>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the game elements for a clear and engaging player experience.
CSS
#gameContainer {
text-align: center;
margin-top: 50px;
}
#problem {
font-size: 24px;
margin: 20px;
}
#playerAnswer {
font-size: 18px;
padding: 10px;
}
#submitAnswer {
display: block;
margin: 20px auto;
padding: 10px 20px;
font-size: 18px;
}
#level, #score {
font-size: 20px;
margin: 10px;
}
Step 3: JavaScript Logic
Implement the game logic, including generating math problems, checking the players’ answers, and updating the level and score.
document.addEventListener('DOMContentLoaded', () => {
const problemElement = document.getElementById('problem');
const answerInput = document.getElementById('playerAnswer');
const submitButton = document.getElementById('submitAnswer');
const levelElement = document.getElementById('level');
const scoreElement = document.getElementById('score');
let level = 1;
let score = 0;
function generateProblem() {
// Simple example: addition problems
let num1 = Math.floor(Math.random() * level * 10);
let num2 = Math.floor(Math.random() * level * 10);
problemElement.textContent = `Solve: ${num1} + ${num2}`;
return num1 + num2;
}
let currentAnswer = generateProblem();
submitButton.addEventListener('click', () => {
let playerAnswer = parseInt(answerInput.value);
if (playerAnswer === currentAnswer) {
score += 10; // Increase score for correct answer
level++; // Move to the next level
answerInput.value = ''; // Clear input for next problem
currentAnswer = generateProblem(); // Generate a new problem
} else {
alert('Wrong answer. Try again!');
answerInput.value = ''; // Clear input for retry
}
updateUI();
});
function updateUI() {
scoreElement.textContent = `Score: ${score}`;
levelElement.textContent = `Level: ${level}`;
}
});
Game mechanics explained
Math Problems: The game presents a math problem to the player, starting simple and getting more complex as the player progresses through levels.
Submitting Answers: The player types their answer into an input box and submits it. If the answer is correct, they gain points and move to the next level, which presents another problem.
Scoring and Levels: Correct answers increase the player’s score and advance them to higher levels, where issues become more challenging.
Enhancements for a Complete Game
To expand this game, consider:
Variety in Problems: Introduce different math problems (subtraction, multiplication, division) as the player advances.
Timer: Add a timer to increase the challenge, giving players limited time to solve each problem.
High Scores: Keep track of high scores, encouraging players to beat their highest scores or compete with others.
Summary
This basic Math Puzzle Game setup offers a fun and educational way to practice math skills, challenging players to solve problems and advance through levels. Expanding the game with various issues, timed challenges, and scoring systems can make it more engaging and rewarding.
Setting Up the Game
HTML Structure: Think of HTML as our game blueprint. We have:
A visual representation of the math problem you need to solve.
An input box (player answer) where you type your answer.
A submit button (submitAnswer) to check if your answer is correct.
The current level (level) and score (score) are displayed.
CSS Styling: CSS is like paint and decoration for our game, making it look excellent and user-friendly. We style:
The game container centers everything on the page.
The math problem and input box are big and easy to read and type in.
The submit button should be clear and clickable.
The level and score are displayed to keep you informed of your progress.
Making the game work
JavaScript Logic: This is where we add the game’s rules and how it reacts to your actions.
Generating Math Problems
The game starts by creating a simple addition problem with two random numbers. These numbers get bigger as you move up in levels, making the problems harder.
The correct answer is stored so the game can check if you received it correctly.
Check your answer.
When you click the “Submit” button, the game looks at what you’ve typed into the input box.
If your answer matches the correct answer, your score increases, and you move to the next level. A new problem appears.
If you get it wrong, the game tells you to try again, and you get another chance at the same problem.
Update the game display.
After each attempt, whether right or wrong, the game updates the display to show your current score and level. This lets you know how well you’re doing.
Playing the game
Objective: Solve as many math problems correctly as you can. Each correct answer increases your score and advances you to a more complex level.
Challenge: The problems get tougher as you progress, testing your math skills and speed.
What Makes the Game Fun?
A high score requires quick, accurate problem solving.
You’re constantly challenged to improve your math skills and beat your previous scores.
Summary for students
It is fun to combine coding with math practice by creating a Math Puzzle Game. Making the game environment appealing and adding interactive and challenging elements makes it a dynamic game that improves math skills and tests them.
Reference Links to Include:
Game Development Fundamentals:
- An introductory guide to basic game development principles that can be applied to creating educational games, including math puzzles.
- Suggested Search: “Game development basics”
Educational Game Design:
- For insights into designing games with educational objectives, particularly in mathematics.
- Suggested Search: “Educational game design principles”
Unity or Unreal Engine Documentation for Educational Games:
- Both Unity and Unreal Engine offer features suitable for developing math puzzle games with engaging mechanics.
Stack Overflow for Game Development Queries:
- A platform to seek advice and solutions to common game development challenges, including those related to educational game mechanics.
Leave a Reply