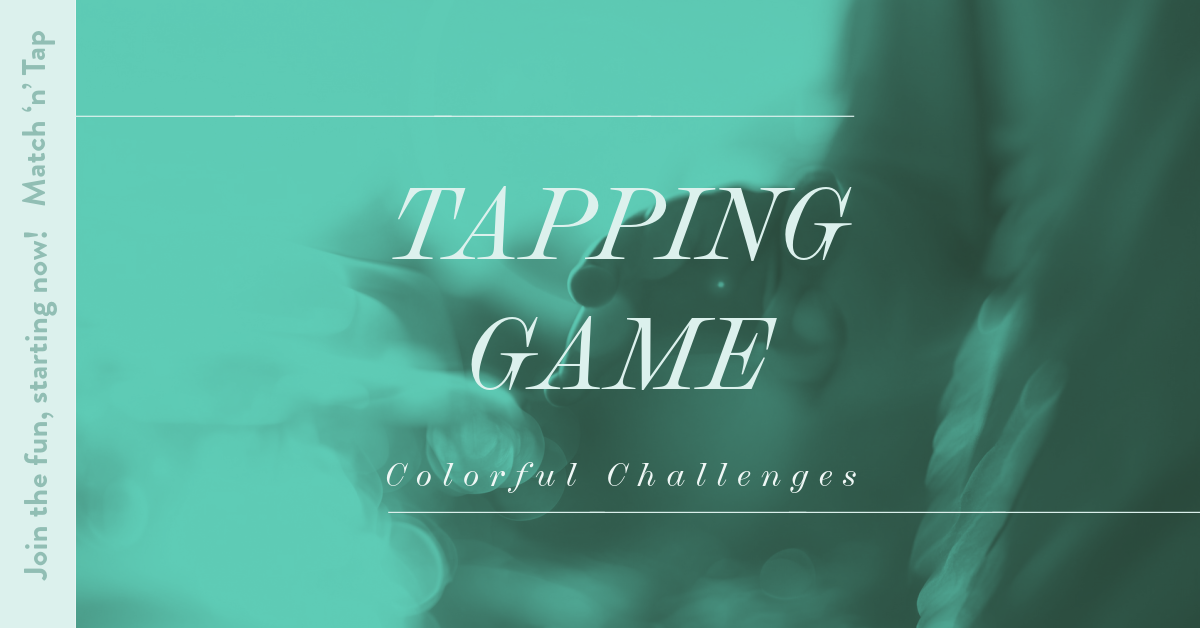
Color Switch clones involve game mechanics where a ball changes colors while the player controls it. They must pass through obstacles that match the ball’s color. This explanation outlines a conceptual approach using web technologies (HTML, CSS, JavaScript) that could be adapted for a mobile game using frameworks like React Native or by creating a web-based mobile game.
Step 1: HTML Structure
Set up a game area and a ball. For simplicity, we’ll not change the ball’s color dynamically in this outline, but you’ll get the concept.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Color Switch Clone</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<div id="ball"></div>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the game area and the ball. Based on game logic, adjust the ball’s background color in JavaScript.
#gameArea {
width: 100vw;
height: 100vh;
background-color: black;
display: flex;
justify-content: center;
align-items: center;
position: relative;
}
#ball {
width: 30px;
height: 30px;
border-radius: 50%;
position: absolute;
bottom: 50px; /* Start position */
background-color: blue; /* Initial color */
}
Step 3: JavaScript Logic
Implement basic color changing mechanics. This example won’t include moving obstacles or comprehensive collision detection but shows how to adjust the ball’s color.
document.addEventListener('DOMContentLoaded', () => {
const ball = document.getElementById('ball');
const colors = ['blue', 'red', 'green', 'yellow']; // Possible ball colors
let currentColorIndex = 0; // Start with the first color
document.addEventListener('click', () => {
// Change the ball's color on click
currentColorIndex = (currentColorIndex + 1) % colors.length; // Cycle through colors
ball.style.backgroundColor = colors[currentColorIndex];
});
// Implement obstacle generation and collision detection based on ball's color
});
Game mechanics explained
Ball and Obstacles: The player controls a ball that must pass through matching-colored obstacles. Each tap changes the ball’s color in a predefined sequence.
Color Changing: The ball changes color by tapping the screen (or clicking on the web version). This mechanic is crucial for matching the ball’s color to upcoming obstacles.
Obstacles: In a complete game, obstacles with different colored sections move toward the ball, and the player must change the ball’s color accordingly to pass through matching parts.
Enhancements for a Complete Game
Dynamic Obstacles: Generate moving obstacles with different colored sections. The obstacles could move vertically toward the player or rotate, requiring timely color changes to pass.
Collision Detection: Implement logic to detect when the ball’s color matches the obstacle section it passes through. If there’s a mismatch, the game ends or restarts.
Scoring and Levels: Increase the game’s difficulty as the player progresses through faster-moving obstacles or more complex patterns. Track the player’s score based on how many obstacles they’ve navigated.
Adapted for mobile.
For a mobile-friendly game,
Responsive Design: Ensure the game looks well and is playable on various screen sizes. Adjust #gameArea and #ball sizes as needed.
Touch Inputs: The game already uses a simple tap to change the ball’s color, which translates well to touch screens. Ensure touch events are responsive and accurate.
Frameworks: Consider using a mobile app development framework like React Native for a native app experience or adapting the web game for mobile browsers with touch support.
Starting with the basics.
The game area and ball
HTML Structure: Think of HTML as a playground. We have a game area (#gameArea) where everything happens and a ball (#ball) that the player controls.
Designing CSS is like choosing paint and decorations for a playground. The game area is the whole screen; we’ve made it black, like outer space. The ball is a colorful circle at the bottom of this area.
Make the ball change color
JavaScript Magic: JavaScript lets us add magic to the playground. We’ve taught the ball to change color whenever the player taps or clicks on the screen. It knows only a few colors (blue, red, green, yellow) and changes between them in order.
How to play?
Tap or Click: Each time you tap the screen, the ball changes to the next color in its small list. Imagine it’s like a chameleon changing its colors to match the leaves it’s sitting on.
The Goal: In the game’s full version, obstacles come towards the ball. Each obstacle has different parts. The player needs to tap the screen and change the ball’s color to match the part of the obstacle the ball needs to pass through.
Building the Full Game
To make this a complete game, you would add:
Moving Obstacles: These are obstacles that move towards the ball. Each has different colored sections, and the ball must pass through the section that matches its color.
Winning and Losing: You keep playing, passing through obstacles, until you miss matching the colors. You get a score based on how many obstacles you pass.
Making It Mobile-Friendly
For a game you can play on phones and tablets:
Touches Instead of Clicks: The game already works with taps, which is appropriate for mobile devices. We make it easy to tap the screen to change the ball’s color.
Looks Well on All Screens: The playground (game area) should look good, whether it’s a small phone or a big tablet screen. There should be no obstacles to seeing and touching everything.
Learning Through Games
Creating a game like Color Switch is a fun way to learn coding. You work with HTML and CSS to set up and style the game, then use JavaScript to make it interactive. Also, you make sure the game is fun, challenging, but not too complicated for players.
I recommend focusing on several types of resources:
Game Development Platforms Documentation:
- Unity Unity’s official documentation and tutorials are invaluable for learning game development basics, including physics, animations, and color matching mechanics.
- Unreal Engine Explore Unreal Engine’s documentation for advanced visual effects and game mechanics that could enhance your tapping game.
Coding Tutorials and Courses:
- Codecademy Offers interactive programming courses, including game development with JavaScript, which could be useful for creating browser-based tapping games.
Game Development Communities and Forums:
- Stack Overflow A great place to ask specific questions about game development challenges and search for advice on color matching algorithms or game design.
- Reddit – r/gamedev A community where you can share your game development progress, get feedback, and find inspiration from other game developers.
Online Articles and Blogs:
- Gamasutra Features postmortems and articles by game developers, offering insights into the game development process, including design and programming challenges.
- Medium Search for game development topics where indie developers and professionals share their experiences and tips on creating engaging games.
Leave a Reply