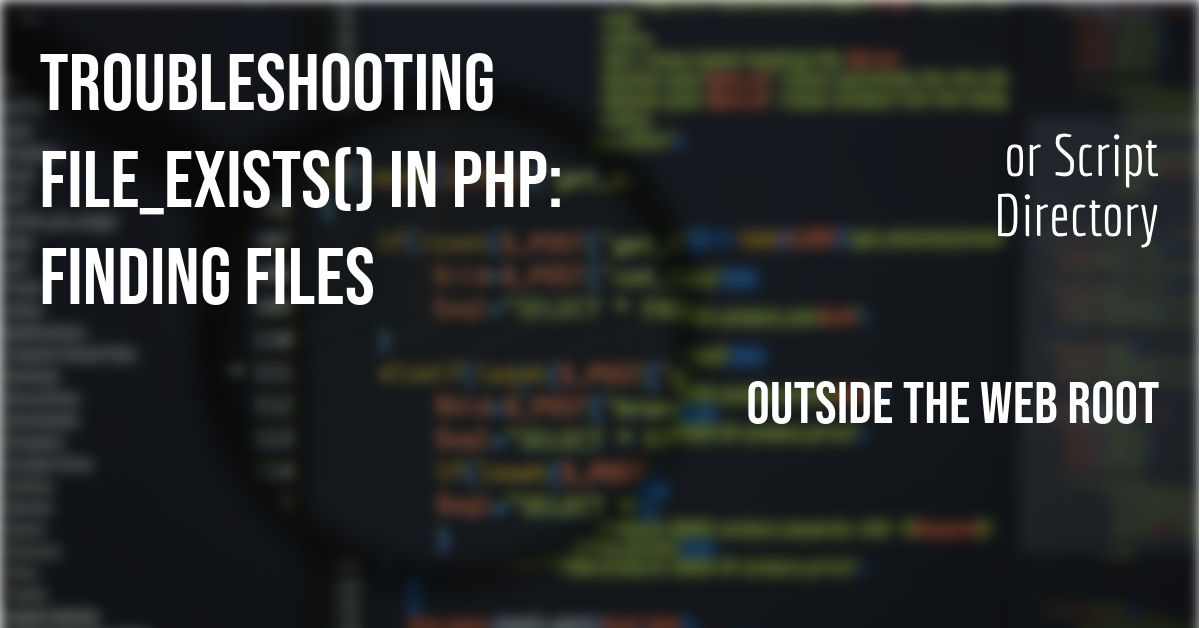
When you encounter an issue where the file_exists() function in PHP does not find files outside of the web root or script directory, it’s typically due to one of several reasons. These include file path issues, server configuration, or permission restrictions. Here are some troubleshooting steps and solutions to consider.
Absolute vs. Relative paths
Issue: Using relative paths can lead to inconsistent results depending on where the script is executed.
Solution: Always use absolute paths to reference files. You can dynamically generate absolute paths using DIR, dirname(__FILE__), or the getcwd() function for the current working directory.
// Example using __DIR__
$filePath = __DIR__ . '/../outside/webroot/myfile.txt';
if (file_exists($filePath)) {
echo "File exists.";
} else {
echo "File does not exist.";
}
2.Permissions
Issue: The server process (e.g., Apache or PHP-FPM) might not have permission to read the file or directory.
Solution: Ensure the file and its parent directories have the correct permissions. Typically, the server should have read access to the file.
chmod 644 /path/to/your/file.txt
And for directories:
chmod 755 /path/to/your/directory
Open_basedIR Restriction
Issue: PHP’s open_based_dir configuration directive limits PHP’s files to the specified directory and its subdirectories. Accessing files outside of these directories will fail.
Solution: Check your php.ini file or server configuration for open_basedir restrictions. You can modify the open_basedir setting to include the directory where your file is located but do so cautiously as it affects security.
; Example php.ini configuration
open_basedir = "/var/www/html:/path/to/your/directory"
SELinux context
Issue: If SELinux (Security-Enhanced Linux) is enforced, web servers may not access files outside typical web directories.
Solution: Adjust SELinux policies to allow access or change the file context to be accessible by the web server. This is more advanced and should be done carefully to avoid security risks.
# Example: Changing SELinux context
chcon -t httpd_sys_content_t /path/to/your/file.txt
Symbolic links
Issue: If you’re trying to access a file via a symbolic link and it’s not working, it might be due to server configuration.
Solution: Ensure that your server configuration allows the following symbolic links. The FollowSymLinks option should be enabled in the .htaccess or server configuration file for Apache.
Final Notes
Always validate the file path and ensure it’s correctly formatted.
Consider security implications when allowing access to files outside the web root. Serving files through a PHP script can help implement access controls.
If troubleshooting doesn’t resolve the issue, check server error logs for specific messages that can guide further actions.
The PHP Manual on the official PHP website.
Leave a Reply