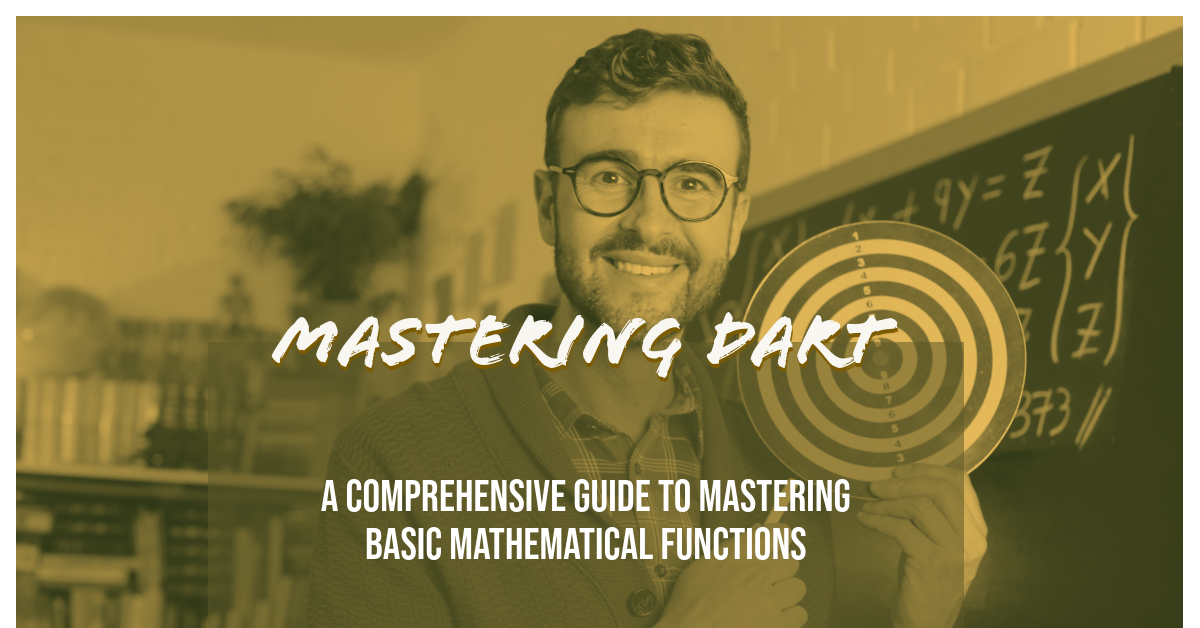
In order to write Dart source code for essential mathematical functions, we define functions that perform addition, subtraction, multiplication, and division, in addition to modulus, exponentiation, and square root. Dart is a modern language extensively used for mobile and web development, particularly with the Flutter framework. Below is an example of how to implement these essential functions in Dart:
void main() {
// Examples of using the functions
print('Addition: ${add(5, 3)}');
print('Subtraction: ${subtract(10, 4)}');
print('Multiplication: ${multiply(6, 7)}');
print('Division: ${divide(8, 2)}');
print('Modulus: ${modulus(9, 4)}');
print('Exponentiation: ${power(2, 3)}');
print('Square Root: ${squareRoot(16)}');
}
// Function to add two numbers
double add(double a, double b) {
return a + b;
}
// Function to subtract two numbers
double subtract(double a, double b) {
return a - b;
}
// Function to multiply two numbers
double multiply(double a, double b) {
return a * b;
}
// Function to divide two numbers
double divide(double a, double b) {
if (b == 0) {
throw ArgumentError('Cannot divide by zero');
}
return a / b;
}
// Function to find the modulus (remainder of a division)
double modulus(double a, double b) {
return a % b;
}
// Function for exponentiation
double power(double base, int exponent) {
return double.parse(pow(base, exponent).toString());
}
// Function to find the square root
double squareRoot(double number) {
return sqrt(number);
}
This Dart code defines basic mathematical operations. The primary functions provide examples of how to call each logical function. Dart’s math library, implicitly imported into Dart, provides more complex mathematical operations and constants (e.g., pow for exponentiation and sqrt for square roots). Still, those calls have been abstracted into our custom functions for simplicity and clarity.
Please note, for power and square-root functions, you may need to import Dart’s Dart: math library at the beginning of your code file:
import 'dart:math';
It ensures you can access the POW and SQRT functions for exponentiation and square root calculations.
Reference Links:
- Official Dart Documentation – ‘dart:math’ Library‘
Leave a Reply