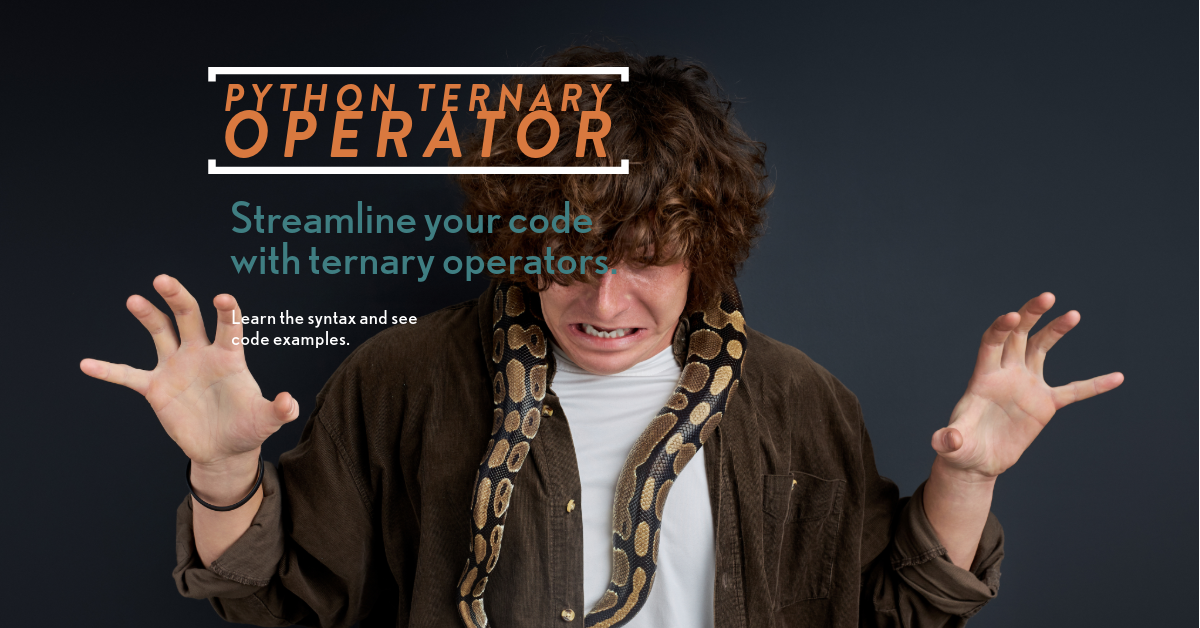
Python has a ternary conditional operator, a one-liner that allows quick decision-making within a single line of code. The Python ternary operator syntax is:
[on_true] if [condition] else [on_false]
This operator evaluates the condition ([condition]). If the condition is True, it returns the value of [on_true]; if the condition is False, it returns the value of [on_false].
Example of Usage
Here’s a simple example that uses the ternary conditional operator to check if a number is odd or even:
num = 5
result = "Odd" if num % 2 != 0 else "Even"
print(result)
In this example, num % 2 != 0 checks if num is odd. If the condition is True, the result is “Odd.” If the condition is False, the result is “Even.” When you run this code with num = 5, it prints “Odd” because 5 is indeed an odd number.
Another example:
Let’s consider another example where we want to assign a minimum of two variables to a third variable:
a = 10
b = 20
min_value = a if a < b else b
print(min_value)
This code will print ten because a is less than b, so a is assigned to min_value.
Use in Functions
The ternary operator can also be used within functions to return values based on conditions:
def is_adult(age):
return "Adult" if age >= 18 else "Minor"
print(is_adult(20)) # Output: Adult
print(is_adult(16)) # Output: Minor
This function checks if the age passed to it is 18 or older. If so, it returns “Adult”; otherwise, it returns “Minor”.
Nested Ternary Operators
You can nest ternary operators for multiple conditions, though this might impact readability:
age = 15
category = "Child" if age < 13 else ("Teen" if age < 18 else "Adult")
print(category)
This code prints “Teen” because the age is between 13 and 18. It checks if the age is less than 13; if not, it checks if the age is less than 18.
While Python’s ternary conditional operator is powerful and concise, it’s essential to use it judiciously to maintain code clarity. This is especially true when dealing with complex conditions or multiple nested ternaries.
Reference Links:
- W3Schools Python Tutorial (Ternary Operator)
Leave a Reply