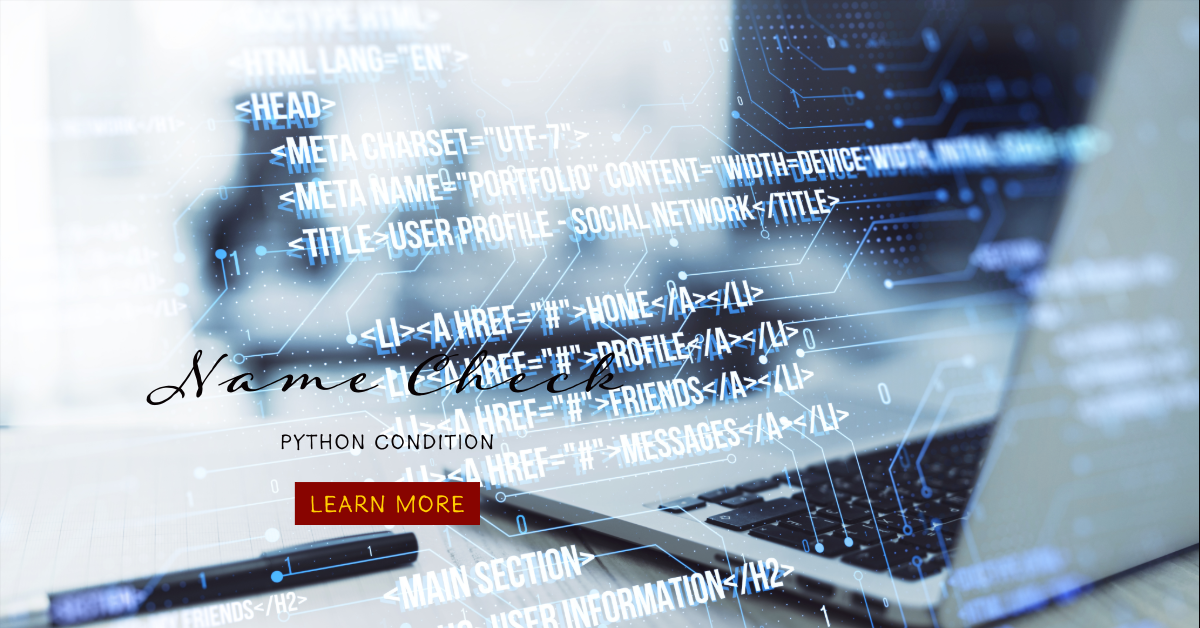
In Python, the special if name == “__main__”: statement checks whether a Python script is being executed directly or imported as a module from another script. This condition becomes true if the script is executed directly. It’s a conversational way to run code that should only execute when run as a standalone file, not when imported elsewhere.
How it works?
Name is a Python variable that represents the current module’s name.
When you execute a Python script directly, Python sets the name variable to “__main__” in that script.
However, if the script is imported as a module in another script, the name is set to the script/module name.
Example
Consider two Python files: main_script.py and imported_module.py.
imported_module.py:
def function_from_module():
print("This function is in imported_module.py")
if __name__ == "__main__":
print("imported_module.py is being run directly")
else:
print("imported_module.py has been imported into another module")
main_script.py
:
import imported_module
def main_function():
print("This function is in main_script.py")
if __name__ == "__main__":
main_function()
Execution flow
When you run imported_module.py directly, the name variable equals “__main__, ” so it prints “imported_module.py is being run directly. “
When you run main_script.py, it imports imported_module.py. In this case, the name in imported_module.py is set to “imported_module,” not “__main__,” so it prints “imported_module.py has been imported into another module.” Then, main_script.py checks its own name variable, finds it set to “__main__”, and calls main_function(), printing “This function is in main_script.py“.
Benefits
Modularity: This feature allows a script to be used as a reusable module and standalone program.
Control Flow provides control over which code blocks are executed, facilitating a clean separation between module definitions (functions, classes) and script execution (test code, standalone functionality).
Testing: It’s beneficial for running tests in the same file as a module’s functions.
The if name == “__main__”: statement is a standard Python idiom for both readability and functionality, ensuring scripts can act as importable modules and standalone programs with specific execution paths.
For reference links and further exploration, while I can’t provide live internet links, consider exploring:
- Python Official Documentation : This section explains how Python treats modules and scripts, including the use of
if __name__ == '__main__':
. - Real Python : Offers in-depth tutorials and articles on Python programming, likely covering the
if __name__ == '__main__':
usage and best practices. - Stack Overflow : A great resource for community-driven explanations and examples of using
if __name__ == '__main__':
in various scenarios.
Leave a Reply