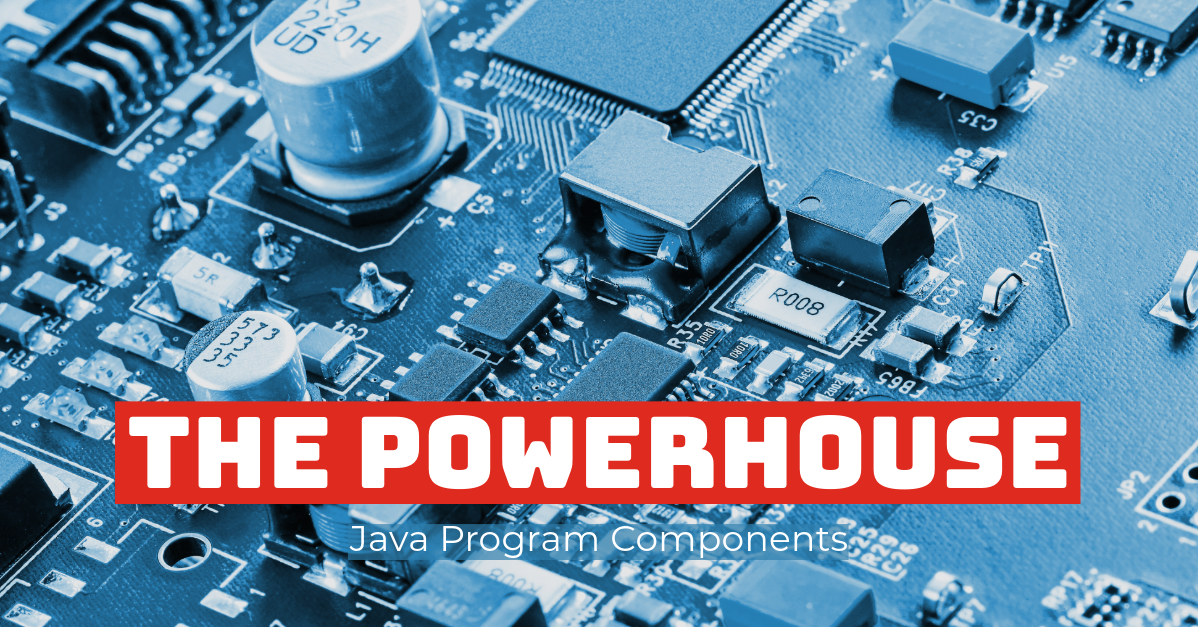
The Java Virtual Machine (JVM) runs Java programs. An integral part of the Java Runtime Environment (JRE) is the Java Virtual Machine, which runs applications written in the Java programming language using libraries, the JVM, and other components. The Java virtual machine runs Java bytecode. This enables Java applications to run on any device or operating system with a JVM based on Java’s “Write Once, Run Anywhere” (WORA) philosophy.
How the JVM works:
Compilation: First, Java source code (.java files) is compiled by the Java Compiler (javac) into bytecode (.class files). Bytecode is intermediate, platform-independent code.
Execution: The JVM reads and executes the bytecode. This process involves various stages, including loading the class files, verifying the bytecode, executing the code, and providing runtime environments such as memory management and security.
Example code:
Let’s consider a simple Java program.
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Steps to Run the Program:
Write and Save: Write the above code in a text editor and save it as HelloWorld.java.
Compile: Compile the source code into bytecode using the Java compiler.
javac HelloWorld.java
- This command generates a
HelloWorld.class
file containing the bytecode. - Run: Execute the compiled bytecode on the JVM using the Java command:
java HelloWorld
This step invokes the JVM, which loads the HelloWorld class, verifies the bytecode, allocates memory, and executes the primary method.
Components of the JVM:
Class Loader: Loads class files.
Bytecode Verifier: Checks the code for illegal code that violates access rights to objects.
Interpreter: Reads the bytecode stream and executes the instructions directly.
Just-In-Time (JIT) Compiler: Improves Java applications’ performance by compiling bytecode into native machine code at runtime.
Garbage Collector: Manages and frees up memory by collecting and discarding no longer used objects.
In conclusion:
Java’s platform independence and security model is based on the JVM. JVMs enable Java programs to run on any device or operating system. In this way, distributed software can be written with minimal consideration for hardware or operating systems.
Reference Links to Include:
Oracle’s Java Documentation:
- For official information on the Java Runtime Environment (JRE) and its components.
Understanding the Java Virtual Machine (JVM):
- Provides insights into the JVM, an integral part of the JRE, and how it executes Java programs.
- Suggested Search: “Java Virtual Machine details”
Real-world Applications of Java:
- To show how Java and its runtime environment are utilized in various industries.
Stack Overflow for Java Runtime Environment Questions:
- A platform for troubleshooting and advice related to the JRE and its functionality.
Leave a Reply