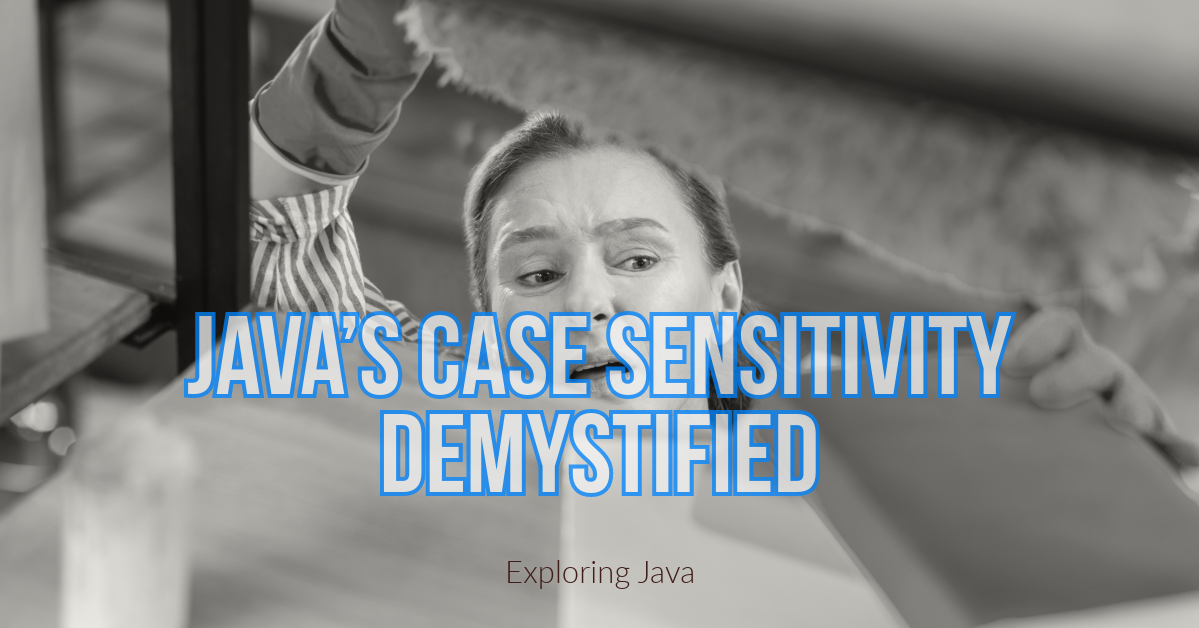
Java is a case-sensitive programming language, which distinguishes between uppercase and lowercase letters. This case sensitivity applies to all parts of a Java program, including identifiers (names of variables, methods, classes, etc.), keywords, and literals.
Example of Case Sensitivity
Consider the following code snippet.
public class CaseSensitiveExample {
public static void main(String[] args) {
int number = 10;
int Number = 20; // Different variable than 'number'
int NUMBER = 30; // Different variable than 'number' and 'Number'
System.out.println(number); // Outputs: 10
System.out.println(Number); // Outputs: 20
System.out.println(NUMBER); // Outputs: 30
}
}
In this example, three variables (number, Number, and NUMBER) are defined, each holding different values. Despite having similar names, they are treated as entirely distinct variables due to Java’s case sensitivity.
Implications of Case Sensitivity
Identifiers: Variables, methods, and classes are case-sensitive. For example, myVariable, MyVariable, and myvariable are considered different identifiers.
Keywords: Java keywords are all lowercase; changing the case would result in a syntax error or the identifier not being recognized as a keyword. For example, it is a keyword, but Int or INT would not be keywords.
Type Names: Since type names are identifiers, the case sensitivity rule applies to them. For instance, a class named MyClass is different from my class.
Method Calls: When calling methods, the case must match the method’s declaration. Calling myMethod() differs from calling the other method if declared differently.
Conclusion
Java’s case sensitivity makes casing crucial. It helps avoid confusion and errors related to identifier misuse. It also underlines the importance of adopting and adhering to a naming convention in your programming practices to ensure code readability and maintainability.
Reference Links to Include:
Oracle’s Java Documentation:
- For authoritative explanations on Java syntax rules, including case sensitivity.
Java Programming Tutorials:
- Offers a deep dive into Java programming fundamentals, highlighting the importance of case sensitivity.
- Suggested Search: “Java programming tutorials
GitHub Java Projects:
- To provide practical examples of how case sensitivity is applied in real Java projects.
Stack Overflow for Java Programming Questions:
- A valuable resource for discussions and questions related to Java’s case sensitivity and its impact on programming.
Leave a Reply