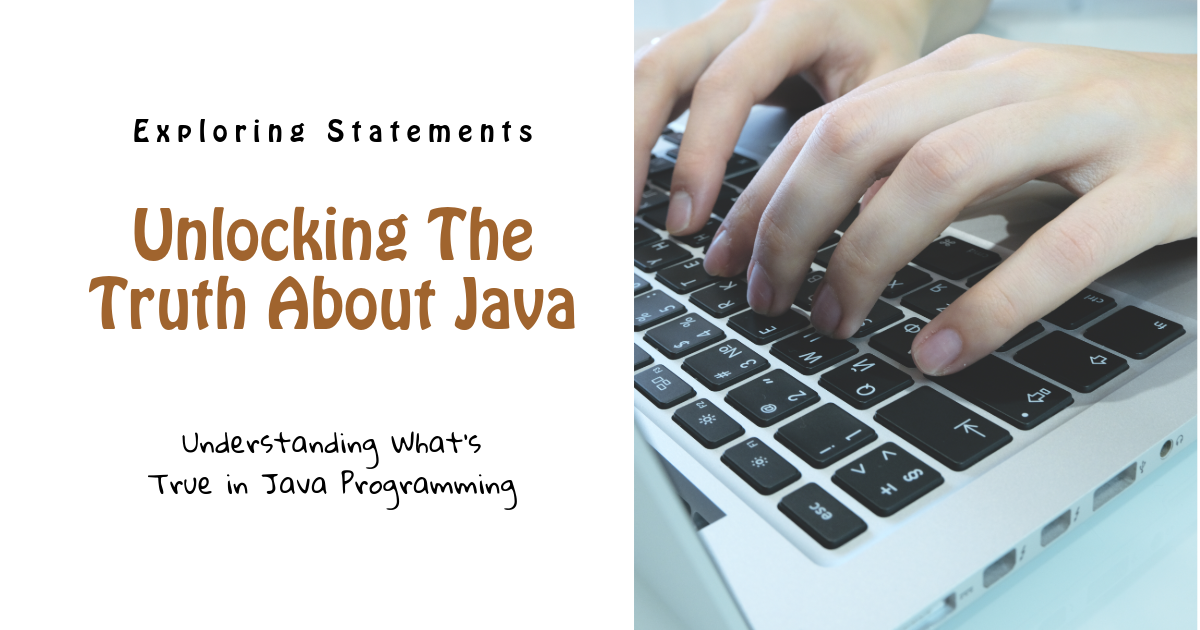
Java is an object-oriented programming (OOP) language. Java uses objects and classes, enabling programmers to create modular, reusable code. In Java, object-oriented programming is based on several fundamental principles, including encapsulation, inheritance, polymorphism, and abstraction.
Encapsulation
Encapsulation is bundling the data (attributes) and methods (functions) that operate on the data into a class unit and restricting access to some of the object’s components. This is typically achieved through access modifiers.
Example Code for Encapsulation:
public class Person {
private String name; // Private variable, encapsulated within the class
public Person(String name) { // Constructor
this.name = name;
}
public String getName() { // Public getter method to access the private name variable
return name;
}
public void setName(String name) { // Public setter method to modify the private name variable
this.name = name;
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("John");
System.out.println(person.getName()); // Accessing name through getter method
person.setName("Doe");
System.out.println(person.getName()); // Accessing the modified name
}
}
Inheritance
A class can inherit properties and methods from another class through inheritance. In Java, inheritance is achieved using the extend keyword.
Example Code for Inheritance:
class Animal { // Base class (parent)
public void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal { // Derived class (child)
public void bark() {
System.out.println("The dog barks.");
}
}
public class TestInheritance {
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // Method from parent class
myDog.bark(); // Method from child class
}
}
Polymorphism
In Java, polymorphism allows objects to be treated as instances of their parent classes rather than their own classes. Java polymorphism types are compile-time (method overloading) and runtime (method overriding).
Example Code for Polymorphism (Method Overriding):
class Bird {
public void sing() {
System.out.println("The bird sings.");
}
}
class Sparrow extends Bird {
@Override
public void sing() {
System.out.println("The sparrow sings melodiously.");
}
}
public class TestPolymorphism {
public static void main(String[] args) {
Bird myBird = new Sparrow(); // Polymorphism
myBird.sing(); // Calls the overridden method in Sparrow
}
}
The abstraction
An abstraction is the process of hiding implementation details from the user and showing only functionality. Abstract classes and interfaces enable Java abstraction.
Example code for abstraction:
abstract class Vehicle { // Abstract class
abstract void start(); // Abstract method
}
class Car extends Vehicle {
void start() {
System.out.println("Car starts with a key.");
}
}
public class TestAbstraction {
public static void main(String[] args) {
Vehicle myCar = new Car(); // Cannot instantiate the abstract class directly
myCar.start(); // Calls the implementation in the Car class
}
}
Encapsulation, inheritance, polymorphism, and abstraction are supported in Java.
Reference Links to Include:
Oracle’s Java Documentation:
- For official documentation on Java programming language constructs and best practices.
Java Programming Tutorials:
- To offer comprehensive guides and examples on Java programming fundamentals.
- Suggested Search: “Java programming tutorials for beginners”
GitHub Java Projects:
- For practical examples of Java coding practices and applications.
Stack Overflow for Java Questions:
- A platform for community discussions, advice, and solutions on Java programming challenges.
Leave a Reply