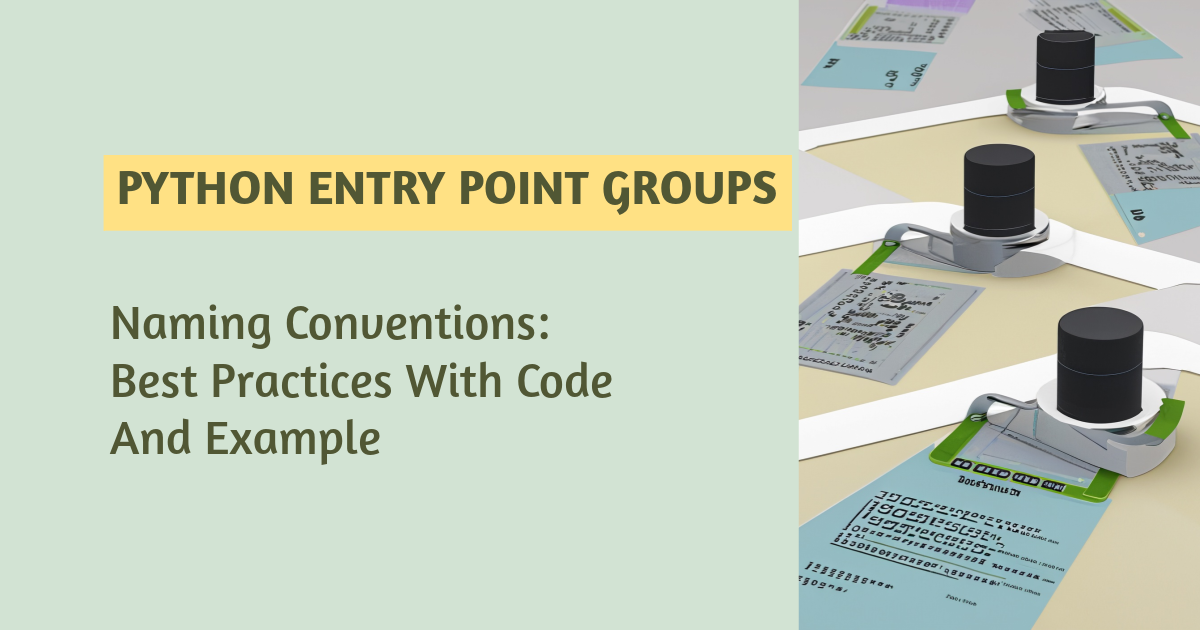
In Python, entry point groups are used in the context of package distribution to specify a group of entry points that a package provides. These entry points can be used for various purposes, such as creating plugins for applications or specifying command-line tools provided by a package. The entry points are defined in the setup.py
file (for setuptools) or pyproject.toml
file (for more modern packaging tools that follow PEP 517/518) of a Python package.
Conventions Around Naming Entry Point Groups
There are no strict conventions enforced by the packaging tools themselves regarding the naming of entry point groups. However, there are some best practices and common patterns observed in the Python community:
Use Namespaces: It’s common to use a namespace-like structure for entry point group names, especially for plugins. For example,
myapp.plugins
for a group of plugins formyapp
. This helps to avoid conflicts between entry point group names from different packages.Be Descriptive: The name of the entry point group should clearly describe its purpose or the type of entry points it contains. For example,
console_scripts
for command-line executables.Follow Existing Patterns: If you are creating entry points for use with a well-known application or framework, follow the naming conventions that it uses. For example, many packages providing plugins for
pytest
use entry point groups named according topytest
‘s expectations, such aspytest11
.
Example
Here’s an example demonstrating how to define entry points in a setup.py
file, using the setuptools
approach. This example defines a command-line tool entry point (console_scripts
) and a hypothetical plugin entry point group for an application named myapp
.
from setuptools import setup, find_packages
setup(
name="mypackage",
version="0.1",
packages=find_packages(),
entry_points={
# Command-line executable scripts
'console_scripts': [
'mycli=mypackage.cli:main',
],
# Plugins for 'myapp'
'myapp.plugins': [
'myplugin=mypackage.plugins.myplugin:PluginClass',
],
},
)
And here’s an example for pyproject.toml
, which is becoming more common with the adoption of PEP 517 and 518 for Python packaging:
[project]
name = "mypackage"
version = "0.1"
[project.entry-points.console-scripts]
mycli = "mypackage.cli:main"
[project.entry-points.myapp.plugins]
myplugin = "mypackage.plugins.myplugin:PluginClass"
Summary
While there’s flexibility in naming entry point groups, adhering to conventions around namespaces and descriptiveness can improve the clarity and maintainability of your package. Additionally, aligning with the naming patterns of the applications or frameworks your package integrates with will ensure compatibility and ease of use for end-users.
Reference Links to Include:
Python Packaging Authority (PyPA) Documentation:
- For official guidelines on Python package development, including entry point naming conventions.
Python Entry Points Explanation:
- Detailed articles or tutorials on how entry points work in Python and their importance in package distribution.
- Suggested Search: “Understanding Python entry points”
Examples of Well-Named Entry Point Groups:
- Practical examples or GitHub repositories showcasing effective use of entry point groups naming in Python projects.
Stack Overflow for Python Packaging Questions:
- A platform for troubleshooting and advice on Python packaging, including entry points and naming conventions.
Leave a Reply