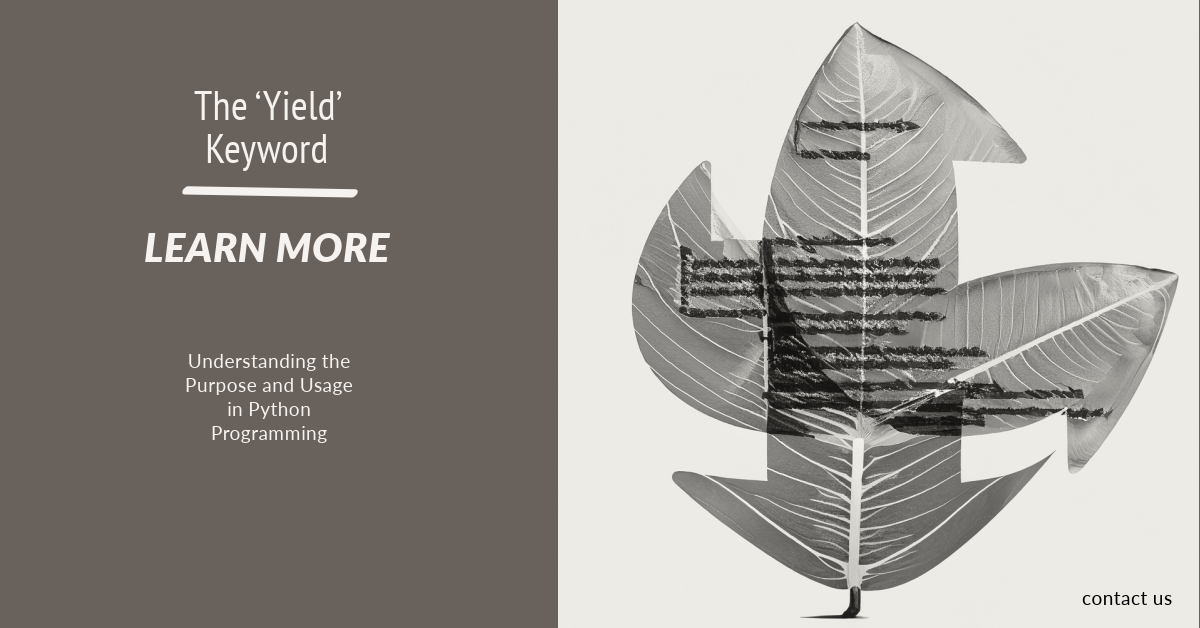
The yield
keyword in Python is used in a function to turn it into a generator. A generator is a special type of iterator that lazily produces values, meaning it generates values on the fly and maintains its state between each generation. This allows for efficient looping over large datasets or complex computations without needing to store the entire dataset in memory at once.
When a function executes a yield
statement, the state of the function is “frozen,” and a value is returned to the caller. The next time the generator’s __next__()
method is invoked, the function resumes execution right after the yield
statement. This behavior continues until the function returns, at which point a StopIteration
exception is raised to signal that the generator is exhausted.
Basic Example of yield
Here’s a simple example to demonstrate how yield
works:
def simple_generator():
yield 1
yield 2
yield 3
# Create a generator object
gen = simple_generator()
# Iterate through the generator
for value in gen:
print(value)
Output:
1
2
3
In this example, simple_generator
is a generator function because it uses the yield
keyword. Each time the loop requests the next value from gen, the generator resumes execution and yields the next value.
Example with State Preservation
Here’s a more complex example that shows how yield
can be used to maintain state and perform computations:
def fibonacci_sequence(n):
a, b = 0, 1
for _ in range(n):
yield a
a, b = b, a + b
# Generate the first 5 Fibonacci numbers
for fibonacci_number in fibonacci_sequence(5):
print(fibonacci_number)
Output:
0
1
1
2
3
This generator function, fibonacci_sequence
, yields the Fibonacci sequence up to n
numbers. The state (values of a
and b
) is preserved between yields, making it efficient for generating sequences like this without needing to store the entire sequence in memory.
Advantages of Using yield
- Memory Efficiency: Since generators produce items one at a time and only when required, they are much more memory-efficient than storing the entire dataset or sequence in memory.
- Laziness: Generators allow for lazy evaluation, computing the next value in a sequence only when needed. This is useful for working with large datasets or infinite sequences.
- Cleaner Code: Using
yield
can lead to cleaner, more readable code, especially when compared to managing iterator state manually.
Generators, facilitated by the yield
keyword, are a powerful feature in Python, enabling efficient data processing and manipulation in a variety of scenarios.
Reference Links to Include:
Python Official Documentation:
- For authoritative information on generators and the yield keyword.
Python Generators and yield:
- Tutorials or articles that explain the concept of generators in Python and how the yield keyword functions within them.
- Suggested Search: “Python generators and yield tutorial”
Effective Use of yield in Python:
- Best practices and advanced use cases for the yield keyword to improve code efficiency and readability.
- Suggested Search: “Effective use of yield in Python”
GitHub Repositories Featuring yield:
- Examples of projects that effectively use the yield keyword, providing practical insights into its application.
Stack Overflow Discussions on Python yield:
- Community advice on common questions and challenges related to the use of yield in Python programming.
Leave a Reply