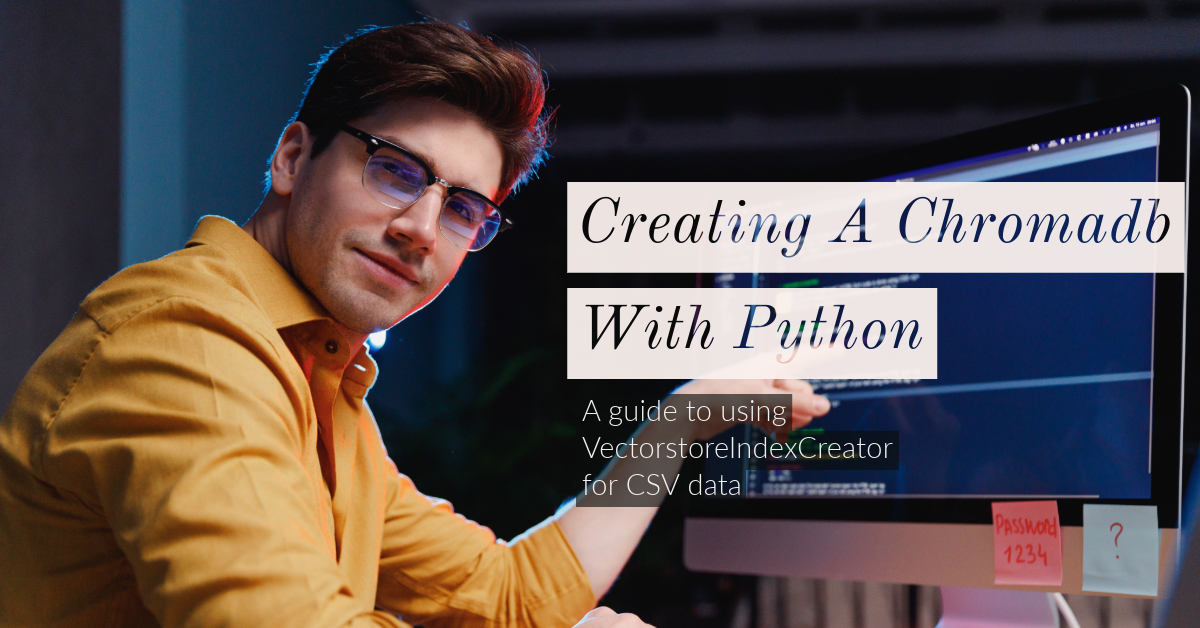
Creating a ChromaDB involves two main steps: preparing the data and building the index with VectorstoreIndexCreator
. Since you mentioned using a CSV file, I’ll guide you through a process that includes:
- Loading the CSV data.
- Preprocessing the data if necessary (depending on your CSV structure and the data you wish to index).
- Using the
VectorstoreIndexCreator
to create the ChromaDB index.
For this explanation, I’ll assume you have a CSV with vectors you want to index. These vectors might represent embeddings from images, texts, or any other data type that can be represented in a vector space. The VectorstoreIndexCreator
is not a standard library or tool in Python, so this example will be somewhat hypothetical, based on what a typical process might involve for creating a vector-based database (e.g., using FAISS, Annoy, or a similar library for creating efficient vector indices).
Step 1: Load the CSV Data
First, you need to read the CSV file into Python. You can use the pandas
library for this, which allows for easy manipulation of tabular data.
import pandas as pd
# Load the CSV file
df = pd.read_csv('your_data.csv')
# Assuming your CSV has columns 'id' and 'vector',
# where 'vector' is a string representation of a list
df['vector'] = df['vector'].apply(eval) # Converts string list to actual list
Step 2: Preprocess the Data
Depending on your needs, preprocessing might involve normalizing vectors, filtering data, or converting data into the appropriate format for indexing.
# Example preprocessing: normalization (if necessary)
# This is highly dependent on your data and use case
import numpy as np
def normalize_vector(v):
norm = np.linalg.norm(v)
return v / norm if norm > 0 else v
df['vector'] = df['vector'].apply(normalize_vector)
Step 3: Create the Index with VectorstoreIndexCreator
As VectorstoreIndexCreator
is not a standard tool, let’s assume a generic approach similar to creating a vector index in FAISS or Annoy. You would typically convert your vectors into a format the index creator expects, then build and save the index.
# Pseudo-code for VectorstoreIndexCreator usage
# Replace this with the actual API calls for your specific library or tool
index = VectorstoreIndexCreator(dimensionality_of_your_vectors)
# Add vectors to the index
for id, vector in zip(df['id'], df['vector']):
index.add(vector, id)
# Build the index
index.build()
# Save the index for later use
index.save('path_to_your_chromadb.index')
This example assumes a generic process for building a vector index. The exact implementation details, including the API calls, will depend on the specific library or tool you’re using for creating the ChromaDB. If you’re using a specific library (like FAISS, Annoy, or something similar) and need more detailed code tailored to that library, please provide more information about the library and the structure of your CSV data.
Reference Links to Include:
Python Data Handling Techniques:
- For tutorials on handling and processing CSV data in Python.
- Suggested Search: “Python CSV data processing tutorial”
Introduction to ChromaDB and VectorstoreIndexCreator:
- To provide a foundational understanding of these tools and their application in data indexing and database creation.
- Suggested Search: “ChromaDB and VectorstoreIndexCreator Python introduction”
GitHub Repositories for Related Projects:
- Examples of projects or libraries that utilize ChromaDB or similar database indexing techniques in Python.
Stack Overflow for Python Programming Questions:
- A resource for troubleshooting and advice on Python programming, especially related to data handling and database creation.
Leave a Reply