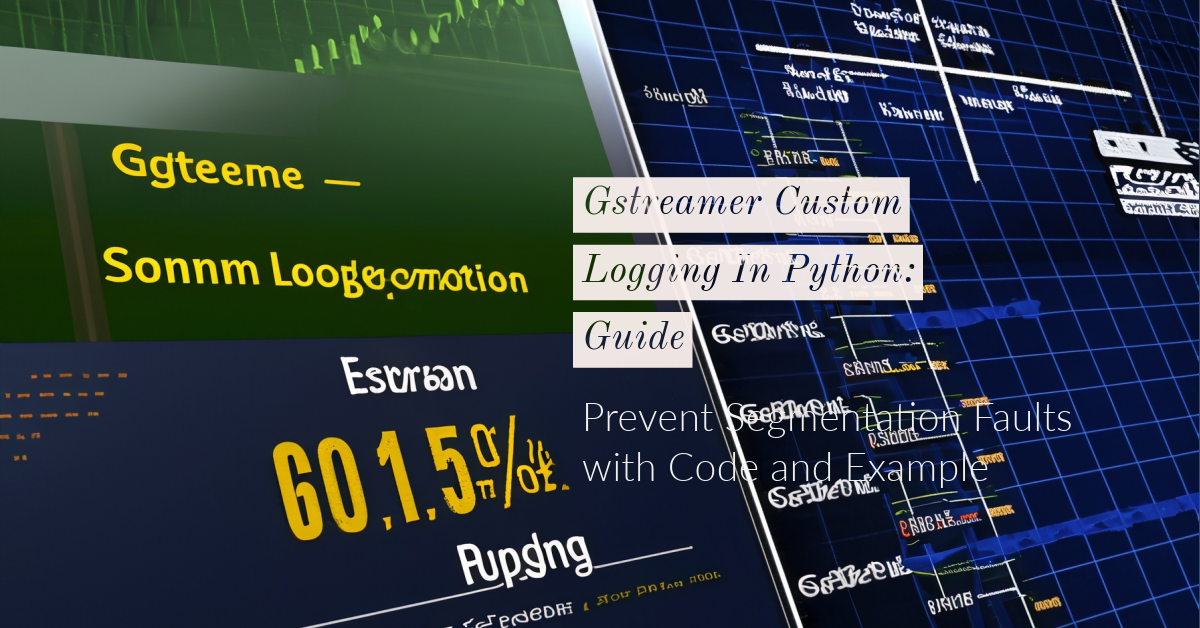
Setting up custom logging for GStreamer from Python requires careful handling, as incorrect setup can lead to issues like segmentation faults, especially when interacting with GStreamer’s C-based API. To safely set up custom logging for GStreamer from Python, follow these guidelines and ensure you’re working within the constraints of both Python’s and GStreamer’s memory management systems.
Pre-requisites:
- Make sure GStreamer is correctly installed on your system.
- You have the GStreamer Python bindings installed (
PyGObject
, along with GStreamer’s GObject introspection data).
Example: Setting Up Custom Logging
Here’s an example of how to set up custom logging in GStreamer from Python. This code assumes you’re familiar with GStreamer’s logging system and Python’s CFFI or ctypes libraries for calling C functions from Python. However, for many users, directly manipulating GStreamer’s logging system at this level might not be necessary, as GStreamer provides environment variables (e.g., GST_DEBUG
) to control logging without needing custom code.
For demonstration purposes, this example will be simplified and not directly invoke C functions to alter the logging system, to avoid the risk of segmentation faults due to incorrect memory handling.
Basic Setup for GStreamer in Python
First, ensure GStreamer is initialized in your Python script. This does not set up custom logging but is the starting point for any GStreamer application.
import gi
gi.require_version('Gst', '1.0')
from gi.repository import Gst
# Initialize GStreamer
Gst.init(None)
Using Environment Variables for Logging
Before delving into custom logging through code, consider whether your needs can be met by configuring the GST_DEBUG
environment variable, which is much simpler and less risky than modifying logging mechanisms via code.
export GST_DEBUG=2 # Sets the global debug level to WARNING
Advanced: Custom Logging with Python
For truly custom logging, you would typically need to interact with GStreamer’s logging API, potentially using ctypes
or CFFI
to call GStreamer’s C functions directly from Python. However, due to the complexities and risks of causing segmentation faults through incorrect API usage or memory management, such an approach is not provided here.
If you absolutely must implement custom logging that cannot be achieved through GStreamer’s environment variables and require direct API manipulation, it is strongly recommended to:
- Thoroughly understand GStreamer’s C API for logging.
- Have a solid grasp of Python’s foreign function interfaces (
ctypes
orCFFI
). - Ensure all memory management is correctly handled, especially for any strings or objects passed between Python and C.
Debugging Segmentation Faults
If you encounter segmentation faults while working with GStreamer and Python:
- Check all pointers and memory allocations involved in calls to C functions.
- Use tools like
gdb
(GNU Debugger) to trace the segmentation fault. - Ensure your environment (Python, PyGObject, GStreamer) is correctly set up and compatible.
Conclusion
For most applications, GStreamer’s built-in logging controlled by environment variables should be sufficient. Direct manipulation of the logging system via Python should be approached with caution and a deep understanding of both GStreamer’s C API and Python’s capabilities for interacting with C code.
Reference Links to Include:
GStreamer Official Documentation:
- For comprehensive details on using GStreamer, including custom logging features.
Python and GStreamer Integration:
- Guides or tutorials on integrating GStreamer with Python for multimedia processing and logging.
- Suggested Search: “Python GStreamer integration tutorial”
Handling Segmentation Faults in Python:
- Techniques and tips for debugging and preventing segmentation faults in Python applications.
- Suggested Search: “Preventing segmentation faults Python”
GitHub Repositories Featuring GStreamer and Python:
- Examples of projects that use GStreamer with Python, showcasing custom logging implementations.
Stack Overflow Discussions on GStreamer and Python:
- Community advice on common issues and solutions related to GStreamer logging in Python.
“>
Leave a Reply