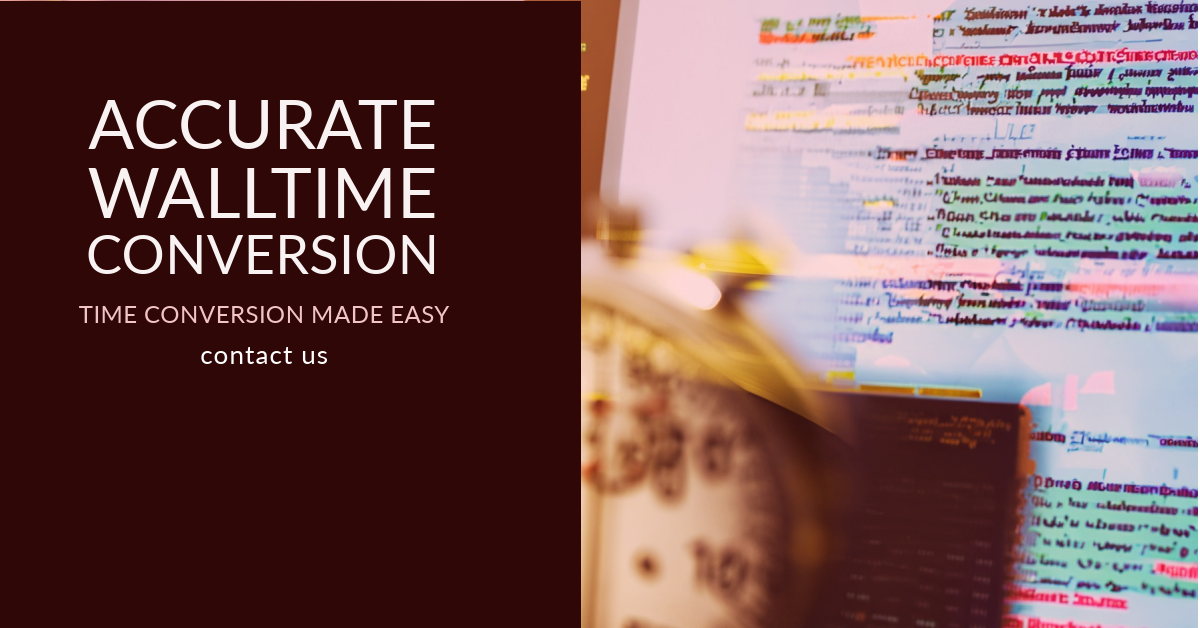
Converting a time value from time.monotonic()
to wall time (the actual date and time) in Python requires a reference point because time.monotonic()
measures time elapsed since an unspecified point (which is not affected by system clock updates). The monotonic clock is useful for measuring durations with high accuracy without being affected by changes in the system clock.
To achieve an accurate conversion from monotonic time to wall time, you need to record both a time.monotonic()
timestamp and the corresponding wall time at a reference moment. You can then calculate the wall time of future time.monotonic()
timestamps by adding the elapsed monotonic time to the reference wall time.
Here’s how to implement this:
Step 1: Capture Reference Points
First, capture both the monotonic time and the corresponding wall time as your reference points.
import time
from datetime import datetime, timedelta
# Capture reference points
monotonic_ref = time.monotonic()
walltime_ref = datetime.now()
Step 2: Convert Monotonic Time to Wall Time
To convert a later time.monotonic()
timestamp to wall time, calculate the difference from the reference monotonic time, and add this difference to the reference wall time.
def monotonic_to_walltime(monotonic_timestamp):
elapsed_since_ref = monotonic_timestamp - monotonic_ref
return walltime_ref + timedelta(seconds=elapsed_since_ref)
Example Usage
This example demonstrates capturing a reference point and converting a future monotonic time back to wall time.
# Assuming the reference points are already captured
time.sleep(5) # Simulate some time passing
# Get a future monotonic time
future_monotonic = time.monotonic()
# Convert to wall time
future_walltime = monotonic_to_walltime(future_monotonic)
print("Future wall time corresponding to the monotonic timestamp:", future_walltime)
Considerations
- This method depends on the accuracy of the initial reference points. Any significant delay between capturing
monotonic_ref
andwalltime_ref
reduces accuracy. - The system’s clock should not be significantly adjusted between capturing the reference wall time and converting monotonic times to wall time. While
time.monotonic()
is immune to changes in the system clock, the reference wall time (and thus the conversion) could be affected. - This approach provides a practical method to relate monotonic timestamps to real-world time, but it’s best suited for applications where the duration between capturing the reference points and using them is relatively short and controlled.
This method is useful in scenarios where you need the benefits of both monotonic time (for its steadiness and immunity to clock adjustments) and wall time (to relate time measurements to real-world dates and times).
Reference Links to Include:
Python Official Documentation on time.monotonic:
- For authoritative information on the time.monotonic function.
Understanding Walltime:
- An introduction to wall clock time and its significance in programming.
- Suggested Search: “Understanding Walltime in programming”
Time Conversion Techniques in Python:
- Tutorials or guides on performing various time conversions, including monotonic time to real (wall) time.
- Suggested Search: “Python time conversion techniques”
GitHub Repositories Featuring time.monotonic Usage:
- Examples of projects utilizing time.monotonic for timing or synchronization purposes.
Stack Overflow for Python Time Handling Questions:
- A platform for troubleshooting and advice on handling time in Python, including conversions.
Leave a Reply