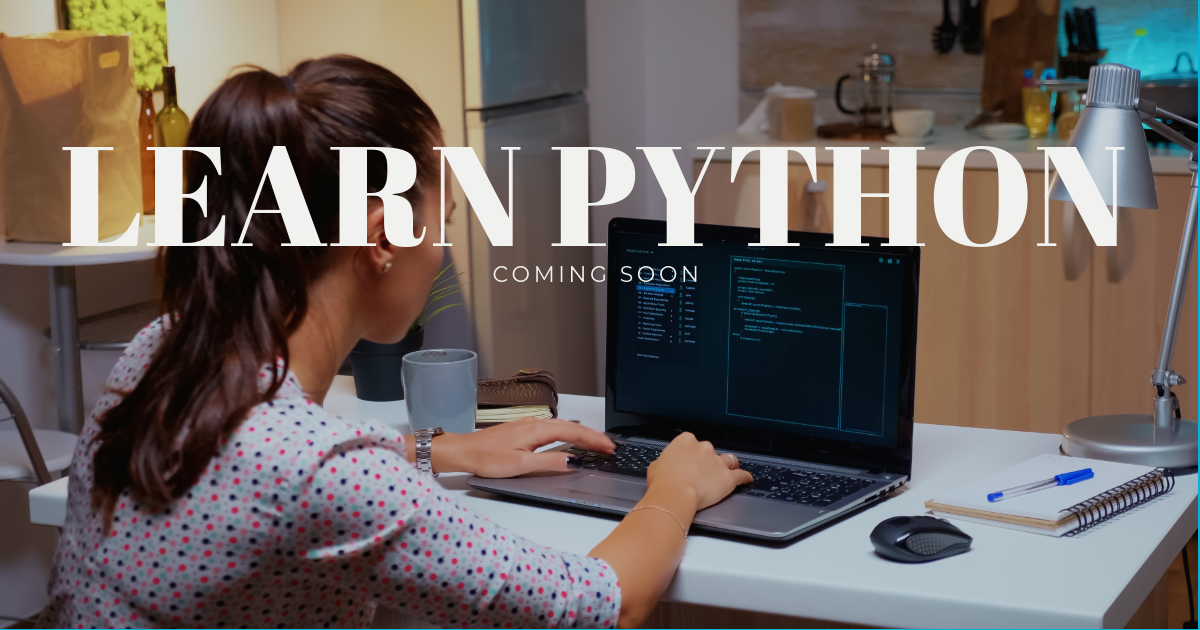
Tic-Tac-Toe Game Plan:
Language: Python, which is simple and widely used in education.
Library: No specific library is needed; we’ll use Python’s built-in functions.
Game elements:
A 3×3 grid for the game board.
Two players mark the grid with an X or an O.
Features:
Function to display the game board.
Function to take player input and update the board.
Check for win or draw conditions after each turn.
A simple loop to alternate turns between players.
User interface:
Console-based text output displays the game board and instructions.
Tic-Tac-Toe Game Code:
def print_board(board):
for row in board:
print(" | ".join(row))
print("-" * 9)
def check_win(board, player):
win_conditions = [
[board[0][0], board[0][1], board[0][2]],
[board[1][0], board[1][1], board[1][2]],
[board[2][0], board[2][1], board[2][2]],
[board[0][0], board[1][0], board[2][0]],
[board[0][1], board[1][1], board[2][1]],
[board[0][2], board[1][2], board[2][2]],
[board[0][0], board[1][1], board[2][2]],
[board[0][2], board[1][1], board[2][0]],
]
return [player, player, player] in win_conditions
def get_move(board):
while True:
move = input("Enter your move (row col): ")
try:
row, col = map(int, move.split())
if board[row][col] == ' ':
return row, col
else:
print("This cell is already occupied. Try again.")
except (IndexError, ValueError):
print("Invalid input. Please enter row and column numbers (e.g., 1 2).")
def tic_tac_toe():
board = [[' ' for _ in range(3)] for _ in range(3)]
current_player = 'X'
for _ in range(9):
print_board(board)
print(f"Player {current_player}'s turn.")
row, col = get_move(board)
board[row][col] = current_player
if check_win(board, current_player):
print_board(board)
print(f"Player {current_player} wins!")
return
current_player = 'O' if current_player == 'X' else 'X'
print_board(board)
print("It's a draw!")
if __name__ == "__main__":
tic_tac_toe()
How to run:
Save this script as a .py file.
Run the script using Python, and the game will start on the console.
Players enter their moves as row and column numbers (e.g., entering 1 or 2 marks the middle cell of the top row).
This code provides a simple console-based Tic-Tac-Toe game. Two players mark Xs and Os on a 3×3 grid, and the game alternates turns. Every move is checked to see if it has won or drawn.
Understanding the Code
Function to Print the Game Board:
Def print_board(board):
For row on board:
Print(” | “.join(
Print(“-” * 9)
Print_board takes the game board as an argument.
It prints each row of the board, separating cells with | and rows with -.
Functions to Check for a Win:
Def check_win(board, player):
# code to check win conditions…
Check_win checks if the current player has won.
It looks at all possible win conditions (rows, columns, diagonals) to see if any are filled with the player’s mark (X or O).
Function to Get Player’s Move:
Def get_move(board):
# code to get and validate the player’s move…
Ask players to enter their moves as row and column numbers.
Check if the chosen cell is empty (‘ ‘) and the input is valid.
The Main Game Function: TIC_TAC_TOE:
Def tic_tac_toe():
# code to initialize the game and handle turns…
Initialize the game board as a 3×3 grid of empty spaces.
Alternates turns between players.
It uses get_move to get the player’s move and updates the board.
Check for a win after each move with check_win.
Announce the result (win or draw).
Running the game:
If name == “__main__”:
Tic_tac_toe()
This line runs the tic_tac_toe function when the script is executed.
Gameplay
The board is a 3×3 grid, and players alternate turns marking Xs and Os.
Players input their moves as row and column numbers (e.g., 0 0 for the top-left corner).
A winner is determined after each move in the game.
A draw occurs if all the cells have been filled without a winner.
Learning Points for Students
Loops: The game uses loops to alternate turns and runs.
Functions: Functions like print_board, check_win, and get_move modularize the code, making it organized and reusable.
Conditionals: The code uses if-statements to check game conditions like wins or valid moves.
Lists: The game board is a list of lists, a simple way to create a 2D grid in Python.
Reference Links to Include:
Python Official Documentation:
- Purpose: For understanding Python syntax and exploring its comprehensive library.
Pygame Documentation (if using Pygame for the game GUI):
- Purpose: Essential for creating the game interface if your guide includes a graphical user interface component.
GitHub Repositories for Tic-Tac-Toe:
- Purpose: To provide examples of other Tic-Tac-Toe projects in Python for inspiration and learning different approaches.
Stack Overflow Python Game Development Questions:
- Purpose: A resource for troubleshooting and learning from the challenges faced by other Python game developers.
Leave a Reply