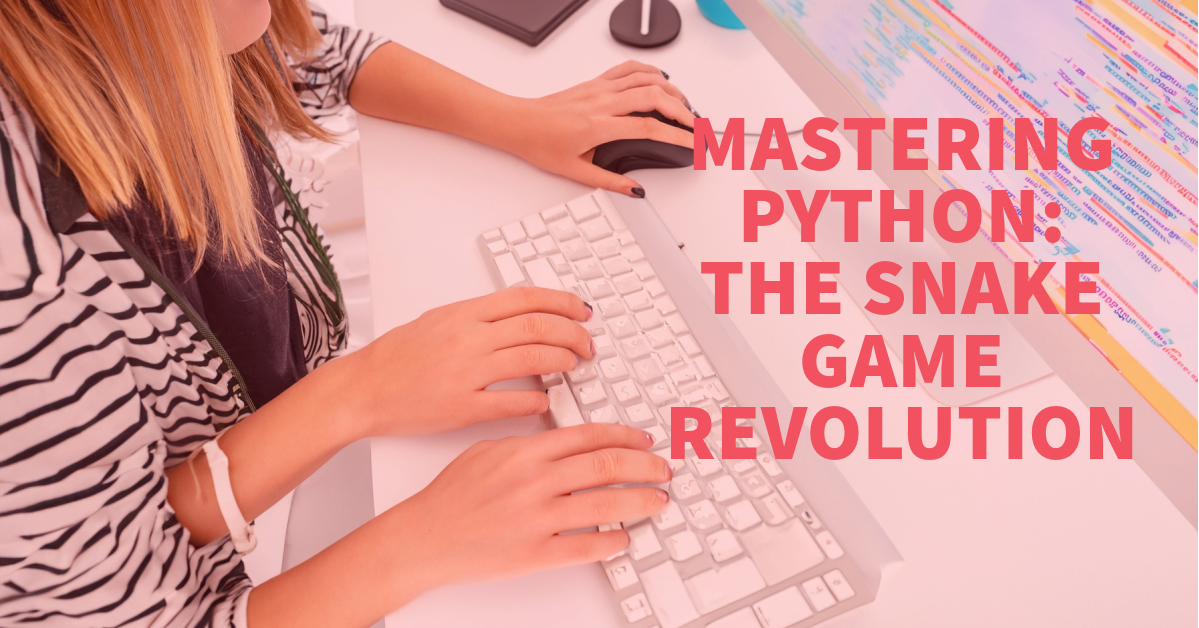
Snake Game Plan:
Language: Python, for its simplicity and effectiveness in teaching game development.
Library: Pygame handles graphics, game events, and critical inputs.
Game elements:
A snake that grows each time it eats an item.
Items for the snake to eat appear randomly on the screen.
Snake must avoid boundaries, along with its tail.
Features:
The movement arrows control the snake’s movement.
Snakes grow longer after eating something.
Random generation of items on the screen.
Game over occurs when the snake hits the wall or its tail.
User interface:
A window showing the snake game.
A scoring system based on the number of items eaten
Snake Game Code :
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants for the game
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 400
SNAKE_SIZE = 20
ITEM_SIZE = 20
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# Setting up the screen
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Snake Game')
# Snake settings
snake_position = [100, 50]
snake_body = [[100, 50], [80, 50], [60, 50]]
direction = 'RIGHT'
change_to = direction
# Item placement
item_position = [random.randrange(1, (SCREEN_WIDTH//ITEM_SIZE)) * ITEM_SIZE,
random.randrange(1, (SCREEN_HEIGHT//ITEM_SIZE)) * ITEM_SIZE]
item_spawn = True
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Handle events
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_UP:
change_to = 'UP'
if event.key == pygame.K_DOWN:
change_to = 'DOWN'
if event.key == pygame.K_LEFT:
change_to = 'LEFT'
if event.key == pygame.K_RIGHT:
change_to = 'RIGHT'
# Validate direction
if change_to == 'UP' and direction != 'DOWN':
direction = 'UP'
if change_to == 'DOWN' and direction != 'UP':
direction = 'DOWN'
if change_to == 'LEFT' and direction != 'RIGHT':
direction = 'LEFT'
if change_to == 'RIGHT' and direction != 'LEFT':
direction = 'RIGHT'
# Move the snake
if direction == 'UP':
snake_position[1] -= SNAKE_SIZE
if direction == 'DOWN':
snake_position[1] += SNAKE_SIZE
if direction == 'LEFT':
snake_position[0] -= SNAKE_SIZE
if direction == 'RIGHT':
snake_position[0] += SNAKE_SIZE
# Snake body growing mechanism
snake_body.insert(0, list(snake_position))
if snake_position == item_position:
item_spawn = False
else:
snake_body.pop()
# Item placement
if not item_spawn:
item_position = [random.randrange(1, (SCREEN_WIDTH//ITEM_SIZE)) * ITEM_SIZE,
random.randrange(1, (SCREEN_HEIGHT//ITEM_SIZE)) * ITEM_SIZE]
item_spawn = True
# Game Over conditions
for block in snake_body[1:]:
if snake_position == block:
running = False
# Draw everything
screen.fill(BLACK)
for pos in snake_body:
pygame.draw.rect(screen, GREEN, pygame.Rect(pos[0], pos[1], SNAKE_SIZE, SNAKE_SIZE))
pygame.draw.rect(screen, RED, pygame.Rect(item_position[0], item_position[1], ITEM_SIZE, ITEM_SIZE))
# Update the display
pygame.display.flip()
clock.tick(20)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script to start the Snake game.
The Snake game code is divided into understandable parts:
Importing libraries
Import a pygame
Import random
Pygame creates the game window, handles events, and draws graphics.
Random places items randomly on the screen.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 400
SNAKE_SIZE = 20
ITEM_SIZE = 20
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
Initialize the PyGame module.
Set screen size, snake segment size, and item size.
Define the game colors.
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Snake Game’)
Creates the game window and sets its title.
Snake and Item Initialization
Snake_position = [100, 50]
Snake_body = [[100, 50], [80, 50], [60, 50]]
Direction = ‘RIGHT’
Change_to = direction
Item_position = [random.randrange(1, (SCREEN_WIDTH//ITEM_SIZE)) * ITEM_SIZE,
Random.randrange(1, (SCREEN_HEIGHT//ITEM_SIZE)) * [ITEM_SIZE]
Item_spawn = True
Initializes the snake’s starting position and body segments.
Sets the initial movement direction.
Randomly place the first item on the screen.
The Game Loop
While running:
# Handle events …
# Change direction …
# Move the snake …
# Snake eating mechanism …
# Items respawn …
# Game Over conditions …
# Draw the snake and the item …
# Update display …
The main loop is where game logic occurs.
Event Handling and Direction Change
For the events in the program. Event.get():
# Event handling code
Handles keyboard inputs to change the snake’s direction.
Moving the snake
If direction == ‘UP’:
Snake_position[1] = SNAKE_SIZE
# Similar code for other directions
Updates the snake’s position based on the direction.
Growing up, the snake.
Snake_body.insert(0, list(snake_position))
If snake_position == item_position:
Item_spawn = False
Else:
Snake_body.pop()
Add another segment to the snake’s front.
If the snake eats an item, it grows; otherwise, the last segment is removed to simulate movement.
Spawning items
If not item_spawn:
# Randomly place an item
Places another item randomly if the previous one was eaten.
Game Over Conditions
For blocks in snake_body[1:]:
If snake_position == block:
Running = false.
It ends the game if the snake collides with itself.
Drawing on the screen
Screen.fill(BLACK)
# Drawing code for the snake and item
Fills the screen with black.
Draw the snake and the item.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(20)
Update the screen with drawn graphics.
It runs at 20 frames per second.
It is exciting to play the game.
Pygame.quit()
It closes the game window and ends the game.
Reference:
To enrich your guide on developing the Snake Game with Python and provide your readers with additional resources, consider adding the following outbound links. These links will offer further learning materials, tools, and examples relevant to Python game development:
Python Official Documentation:
- Purpose: Essential for readers to reference the official Python documentation for any syntax or standard library questions.
- Purpose: If your tutorial uses Pygame, this documentation is invaluable for readers to delve deeper into game-specific functions and modules.
Real Python Tutorial on Game Development:
- Purpose: Offers a primer on game development with Pygame, which can complement your guide by providing additional examples and best practices.
- Purpose: Showcases community-developed Python snake games, allowing readers to see various coding styles and approaches.
- Purpose: A place for readers to find answers to specific Python programming questions or issues they might encounter while following your tutorial.
Leave a Reply