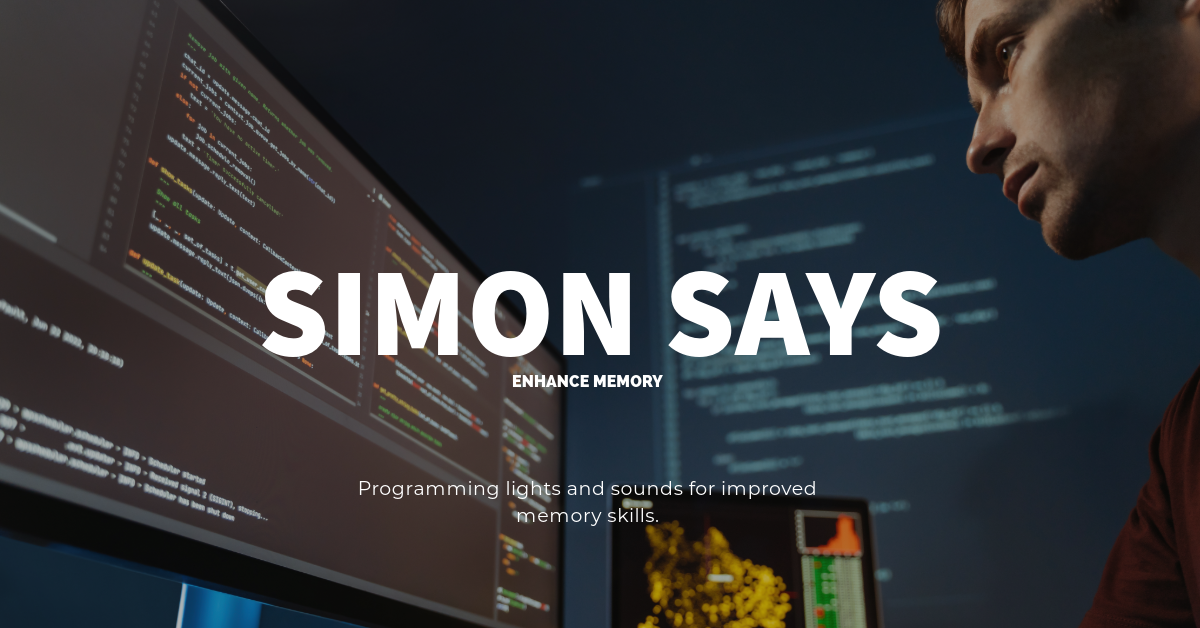
Simon Says Game Plan:
Language: Python, suitable for handling sequences and user interaction.
Library: pygame to handle the graphical interface and user inputs.
Game elements:
A series of colored lights (or buttons) on the screen.
Sounds correspond to each light.
Features:
The game displays a sequence of lights and sounds.
The player must repeat the sequence by clicking on the lights in the correct order.
Each round, the sequence gets longer, increasing the difficulty.
A scoring system based on the number of correctly repeated sequences.
User interface:
Colored lights are displayed in a window.
Optional: Text displayed for the score or level.
Simon Says Game Code:
import pygame
import random
import time
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 600
COLORS = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0)] # Red, Green, Blue, Yellow
BUTTON_SIZE = 200
MARGIN = 50
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Simon Says Game')
# Create buttons
buttons = [pygame.Rect((i % 2) * (BUTTON_SIZE + MARGIN) + MARGIN, (i // 2) * (BUTTON_SIZE + MARGIN) + MARGIN, BUTTON_SIZE, BUTTON_SIZE) for i in range(4)]
# Game variables
sequence = []
player_sequence = []
game_over = False
playing_sequence = True
# Function to display the sequence
def display_sequence(seq):
for color_index in seq:
pygame.draw.rect(screen, COLORS[color_index], buttons[color_index])
pygame.display.flip()
time.sleep(1)
screen.fill((0, 0, 0)) # Clear screen
pygame.display.flip()
time.sleep(0.5)
# Game loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEBUTTONDOWN and not playing_sequence:
for i, button in enumerate(buttons):
if button.collidepoint(event.pos):
player_sequence.append(i)
if player_sequence[-1] != sequence[len(player_sequence) - 1]:
game_over = True
if len(player_sequence) == len(sequence):
playing_sequence = True
player_sequence = []
# Sequence display
if playing_sequence and not game_over:
sequence.append(random.randint(0, 3))
display_sequence(sequence)
playing_sequence = False
# Game over check
if game_over:
print("Game Over! Your score: ", len(sequence) - 1)
break
# Draw buttons
for i, button in enumerate(buttons):
pygame.draw.rect(screen, COLORS[i], button)
pygame.display.flip()
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Simon Says game will start.
This code creates a simple Simon Says game. The game displays a sequence of colored lights (represented by buttons), and the player must click the buttons in the same order. The sequence length increases with each round, making the game more challenging.
Importing libraries
Import a pygame
Import random
Import time
pygame creates to create, handles eventhandleraws graphics to generate random sequences.
Time is used to create delays (pauses) in the game.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 600, 600
COLORS = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0)] # Red, Green, Blue, Yellow
Initialize the Pygame module.
Sets screen dimensions and game button colors.
Creating the game window
Screen = pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame. Display.set_caption(‘Simon Says Game’)
Sets up the game window with the specified width and height.
Set the window title to ‘Simon Says Go.’
Setting Up Game Buttons
Buttons = […]
Creates a list of pygame.Rect objects, each representing a button on the screen.
Game variables
Sequence = []
Player_sequence = []
Game_over = False
Play_sequence = True
The sequence contains a game-generated color sequence.
Player_sequence stores the sequence of colors chosen by the player.
Game_over is a flag to indicate if the game has ended.
Play_sequence indicates whether the game displays a sequence.
Function to display the sequence.
Def display_sequence(seq):
# Code to display each color in the sequence
This function shows each color in the sequence, pausing between each.
Game loop
While running:
# Event handling and game logic
The game’s main loop runs continuously, handling events and updating the game state.
Handling Events (Mouse Clicks)
For events in pygame. Event.get():
# Check for quit and mouse button clicks
Listens for the quit event to close the game and mouse clicks for player input.
Sequence Generation and Player Input
# Code for generating sequence elements
# Code for handling player input and comparing it to the sequence
Add another random element to the sequence at the start of each round.
Checks the player’s input against the game sequence.
The game is over. Check
If game_over:
# Print the score and break out of the loop
If game_over is True, the game prints the player’s score and ends.
Draw the buttons
For i, the button in enumerate(buttons):
pygame. Draw.rect(screen, COLORS[i], button)
Draw each button on the screen with the corresponding color.
Update the display.
pygame. Display.flip()
The screen was updated to show the latest drawings.
It is exciting to play the game.
Pygame.quit()
It closes the game window and ends the game.
Learning Points for Students
Loops and Conditionals: The game uses loops for the primary cycle and conditionals to handle logic.
Event Handling: Responding to user inputs like mouse clicks.
Lists: Use lists to manage sequences and buttons.
Functions: Define and use functions for specific tasks, like displaying a sequence.
Timing: Using time delays to control game pace.
Reference Links to Include:
Python Official Documentation:
- Purpose: To provide essential Python syntax and library information.
Pygame Documentation (for creating game graphics and sounds):
- Purpose: Crucial for those incorporating graphical elements and sounds in their Simon Says game.
GitHub Python Projects for Simon Says:
- Purpose: To offer examples and inspiration from existing Simon Says games developed in Python.
Stack Overflow for Python Game Development:
- Purpose: A great resource for solving common issues and getting advice on game development with Python.
Leave a Reply