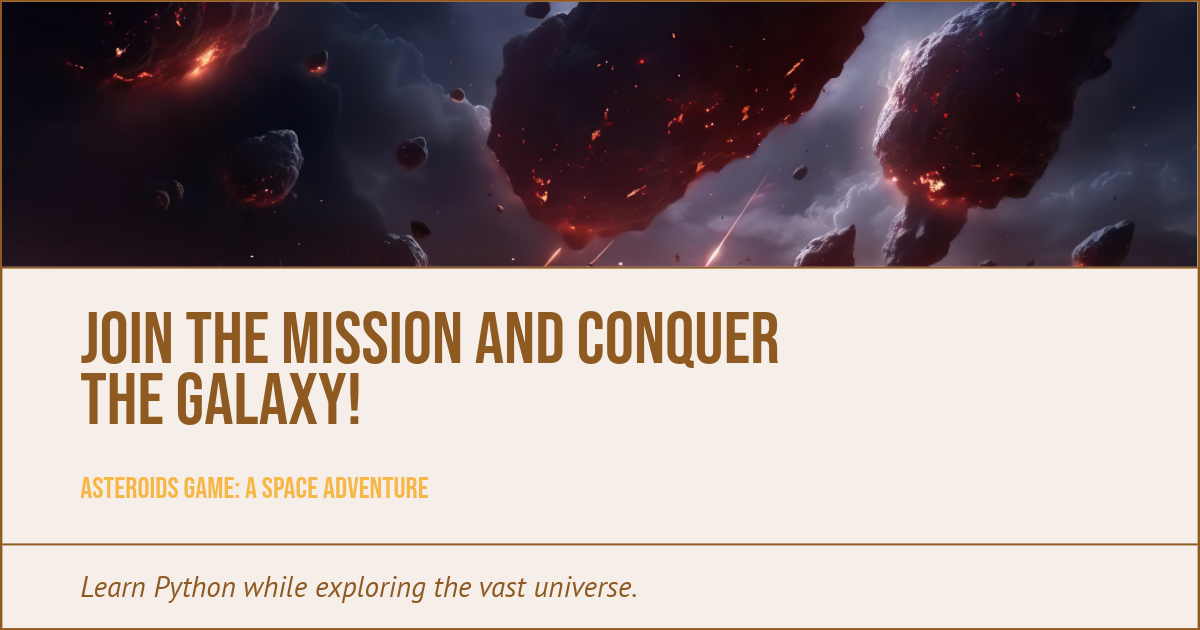
Asteroids Clone Game Plan:
Language: Python is an excellent choice for simple game development.
Library: Pygame for handling graphics, events, and game physics.
Game elements:
A spaceship controlled by the player.
Asteroids of various sizes float around.
Flying saucers are also enemies.
Features:
Ability to move the spaceship around the screen.
The goal is to destroy asteroids and saucers by shooting mechanics.
Asteroids split into smaller pieces when shot.
Score system based on the number of asteroids and saucers destroyed.
User interface:
A window displaying spaceships, asteroids, and saucers.
Display the player’s score.
Asteroids Clone Game Code:
import pygame
import random
import math
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
SHIP_SIZE = 40
ASTEROID_SIZE = 50
BULLET_SIZE = 5
WHITE = (255, 255, 255)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Asteroids Clone')
# Ship setup
ship = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2, SHIP_SIZE, SHIP_SIZE)
ship_speed = 0
ship_angle = 0
# Asteroids setup
asteroids = [pygame.Rect(random.randint(0, SCREEN_WIDTH), random.randint(0, SCREEN_HEIGHT), ASTEROID_SIZE, ASTEROID_SIZE) for _ in range(5)]
# Bullets setup
bullets = []
# Function to rotate the ship
def rotate_ship(angle, speed):
rad_angle = math.radians(angle)
return speed * math.cos(rad_angle), speed * math.sin(rad_angle)
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullet_velocity = rotate_ship(ship_angle, 10)
bullets.append([ship.center, bullet_velocity])
# Ship movement
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT]:
ship_angle += 5
if keys[pygame.K_RIGHT]:
ship_angle -= 5
if keys[pygame.K_UP]:
ship_speed += 0.1
ship_speed *= 0.99 # Ship deceleration
dx, dy = rotate_ship(ship_angle, ship_speed)
ship.move_ip(dx, dy)
# Bullet movement
for bullet in bullets:
bullet[0][0] += bullet[1][0]
bullet[0][1] += bullet[1][1]
# Drawing everything
screen.fill((0, 0, 0)) # Black background
pygame.draw.rect(screen, WHITE, ship) # Draw ship
for asteroid in asteroids:
pygame.draw.rect(screen, WHITE, asteroid) # Draw asteroids
for bullet in bullets:
pygame.draw.circle(screen, WHITE, (int(bullet[0][0]), int(bullet[0][1])), BULLET_SIZE) # Draw bullets
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Asteroids Clone game will start.
This code provides a basic Asteroids Clone game in which the player controls a spaceship that rotates and moves forward. Asteroids are randomly placed on the screen, and the player can shoot bullets to hit them. The game continues until the player closes the window. Collision detection and asteroid splitting are not implemented in this basic version.
Importing libraries
Import a pygame
Import random
Import math
Pygame creates games, manages graphics, and detects user input.
Random generates random asteroids positions.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Constants for ship size, asteroid size, bullet size, and color
Initializes Pygame, which is necessary for game development.
Set constants for screen size, ships, asteroids, bullets, and color (white).
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Asteroids Clone’)
Sets up the game window with the specified width and height.
The window’s title is ‘Asteroids for Everyone.’
Setting Up the Ship, Asteroids, and Bullets
Ship = Pygame.Rect(…)
Asteroids = [… for _ in range(5)]
Bullets = []
The ship is a rectangle that represents the player’s spaceship.
An asteroid is a list of rectangles, each representing an asteroid.
Bullets store the positions and velocities of ship bullets fired.
Function to Rotate the Ship
Def rotate_ship(angle, speed):
# Code to calculate an updated position based on rotation
This function calculates the ship’s updated direction based on its rotation angle.
Game loop
While running:
# Event handling, ship movement, bullet movement…
The game’s main loop continuously runs to update the game state and redraw the screen.
Handling events
For Pygame events. Event.get():
# Check for quit and key presses
Listen for the quit event to close the game and key presses for controlling the ship and shooting bullets.
Ship movement and rotation
# Code for rotating and moving the ship
Allows the player to rotate the ship left or right and move forward.
Bullet movement
For bullets in bullets:
# Update each bullet’s position
Move each bullet according to its velocity.
Drawing the ship, Asteroids, and Bullets
The drawing code for the ship, asteroids, and bullets
He clears the screen and draws the ship, asteroids, and bullets on the screen.
Update the display.
Pygame. Display.flip()
Updates the entire screen with what has been drawn.
Frame Rate Control
Clock.tick(60)
Ensure smooth gameplay at 60 frames per second.
Playing the game is leaving.
Pygame.quit()
It closes the game window.
Learning Points for Students
Game Loop: Understanding the core loop that handles events, updates game state, and renders graphics.
Event Handling: Responding to user inputs like critical presses.
Lists and Loops: Manage and update multiple objects (asteroids and bullets) using lists and loops.
Math and Physics: Basic concepts like rotation, direction, and velocity for game movements.
Drawing Graphics: How to draw shapes (rectangles, circles) to represent game objects.
Reference Links to Include:
Python Official Documentation:
- Purpose: For foundational Python syntax and library information.
- Purpose: Essential for game development, especially if you’re using Pygame for creating the game interface and graphics.
GitHub Python Projects for Asteroids:
- Purpose: To provide examples of Asteroids games developed in Python, offering inspiration and coding insights.
Stack Overflow for Game Development in Python:
- Purpose: A valuable resource for troubleshooting and community advice on Python game development challenges.
Leave a Reply