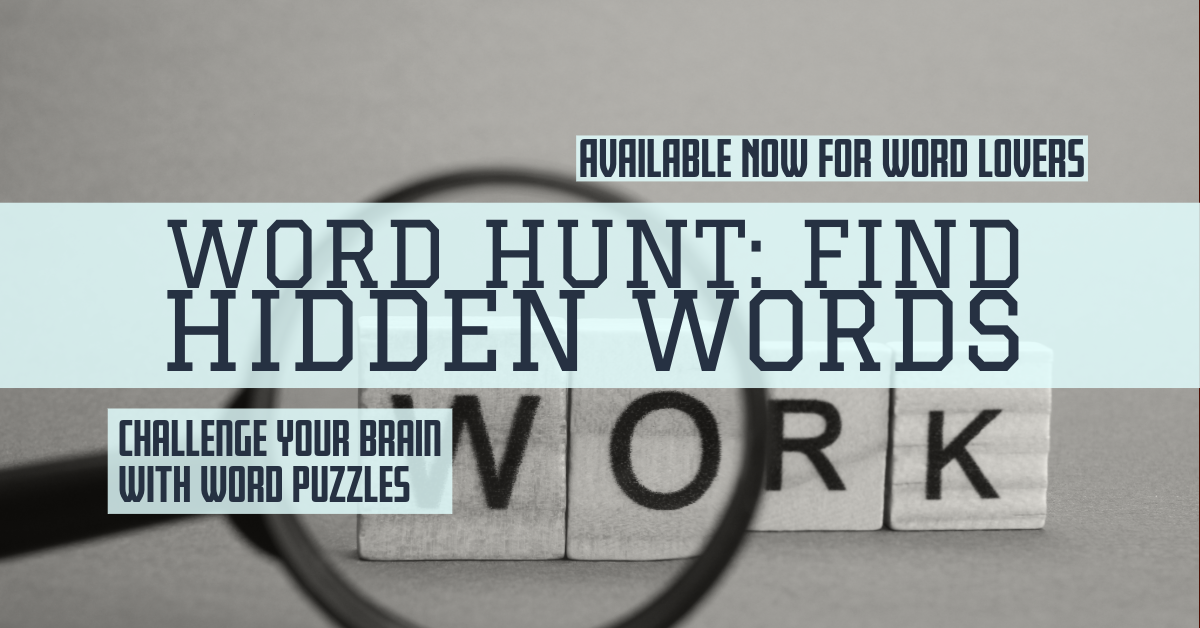
Creating a Word Search game involves creating a grid of letters with hidden words that players need to find. This explanation will guide you through setting up a simple version of the game using HTML for the structure, CSS for styling, and JavaScript for the game logic.
Step 1: HTML Structure
Define the game board and a place to list the words players need to find.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Word Search Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameContainer"></div>
<div id="wordList">Words: <span id="words"></span></div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the grid and the word list for a clear and engaging user interface.
#gameContainer {
display: grid;
grid-template-columns: repeat(10, 40px);
grid-gap: 1px;
margin: 20px auto;
user-select: none; /* Prevent text selection for a better game experience */
}
#gameContainer div {
width: 40px;
height: 40px;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
font-size: 24px;
cursor: pointer;
}
#wordList {
text-align: center;
margin-top: 20px;
}
Step 3: JavaScript Logic
Implement basic game logic, including grid generation and letter selection.
document.addEventListener('DOMContentLoaded', () => {
const gameContainer = document.getElementById('gameContainer');
const wordsElement = document.getElementById('words');
const words = ['HTML', 'CSS', 'JAVASCRIPT'];
const gridDimension = 10; // 10x10 grid
let selectedLetters = [];
wordsElement.textContent = words.join(', ');
// Function to generate the grid
function generateGrid() {
for (let i = 0; i < gridDimension * gridDimension; i++) {
const cell = document.createElement('div');
cell.textContent = String.fromCharCode(65 + Math.floor(Math.random() * 26)); // Random letter
cell.addEventListener('click', () => selectLetter(cell));
gameContainer.appendChild(cell);
}
}
function selectLetter(cell) {
cell.style.backgroundColor = 'lightgreen'; // Highlight selected letter
selectedLetters.push(cell.textContent);
// Add logic to check if the selected letters form one of the words
}
generateGrid();
// Add more functions as needed, e.g., to place the words in the grid, to check for correct selections, etc.
});
Game mechanics explained
Grid Generation: The game starts by creating a 10×10 grid of letters. Each cell in the grid contains a random letter.
Words to Find: A list of words is displayed below the grid. Players need to find these words on the grid.
Selecting Letters: Clicking on a letter selects it. You can add logic to track the player’s selections and check if they form a word from the list.
Finding Words: The objective is to discover and select all the letters of a word listed below the grid. You could highlight a word or cross it off the list when it is seen.
Enhancements
To further develop the game, consider:
Placing Words: Implement an algorithm to place the list of words in the grid, horizontally, vertically, or diagonally.
Checking Selections: Add logic to check if the selected letters match any words. Highlight or cross off words as they are found.
Scoring and Levels: Introduce scoring based on speed or selections, and increase difficulty levels with more words or larger grids.
Summary
This setup provides a foundational structure for a Word Search puzzle game, focusing on generating a grid of letters and selecting them. Enhancements include:
More sophisticated word placement.
Checking for correct word selections.
Added game levels or scoring to increase engagement.
Step 1: Setting Up the Game Board
First, we lay out the game board using HTML, creating a space where the puzzle will live (#gameContainer) and a place to list the words players need to find (#wordList).
Imagine this as drawing a big square on a piece of paper. Write down a list of secret words to hide inside this square.
Step 2: Make it Look Stylish
Next, we used CSS to make our game board and word list look lovely. We created the grid of letters to look neat and ensure each letter has its own space (div). We also styled the word list so it’s easy to read.
It is like coloring our square and ensuring each letter spot is marked and visible. We also produce our list of secret words that look pretty next to our square.
Step 3: Bringing the Game to Life
Now, we add some magic with JavaScript to make our game interactive:
Populating the Grid: We fill our square with random letters, like sprinkling confetti across the paper, but in an organized way, so every spot gets a letter.
Our basic setup does not detail the hidden words, but imagine that confetti forms the hidden words. In a real game, you’d have a clever way to hide these words in the grid.
Finding Words: When you click on a letter, it’s like clicking on a piece of confetti. The game remembers which letters you’ve circled, and you try to circle letters in a way that follows the secret words on the list.
Playing the game
You play by looking at the list of words and then finding those words hidden among the random letters in the grid. When you think you have seen a word, you click on the letters in the grid to “circle” them. If you’ve found an accurate word on the list, you’ve found a treasure!
Enhancements for a Real Game
In the full game version, you’d have:
It’s a clever way to hide the words in the grid (not just random letters everywhere).
A system to check if you’ve circled the correct letters for a word.
Even levels of difficulty, where more challenging levels have more words or are hidden in tricky ways.
Summary for students
Creating a Word Search game is like setting up a treasure hunt. You draw a grid, hide words inside it, and search for them. We use HTML and CSS to set up and style the game board. In JavaScript, we add playability and fun to a game by adding rules and actions. The combination of creativity and coding skills is ideal here.
Reference Links:
- Web Game Development
- HTML5 Canvas for interactive elements.
Leave a Reply