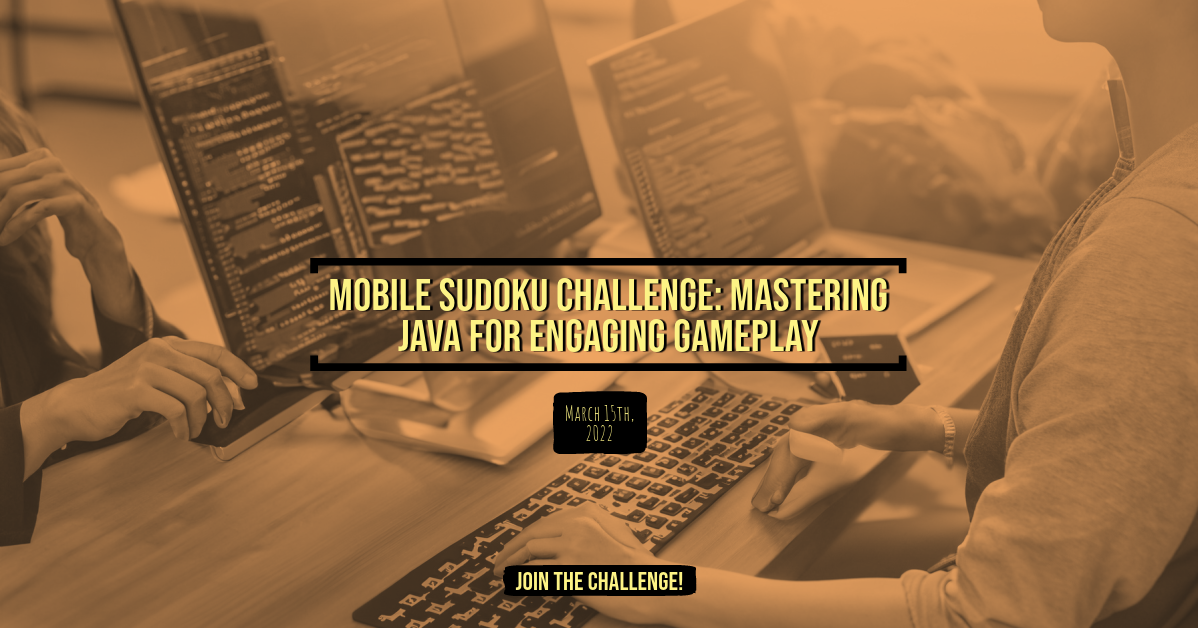
Developing a mobile Sudoku game involves:
- Creating a grid interface for players to input numbers.
- Implementing logic to check for correct answers.
- Generating puzzles of varying difficulty.
While a complete mobile app development tutorial is beyond this format, we can outline a conceptual approach using web technologies (HTML, CSS, JavaScript) that can later be adapted for mobile platforms using frameworks like React Native or web-based mobile apps.
Step 1: HTML Structure
Define the game board with a 9×9 grid and a simple interface for selecting numbers.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Sudoku Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="sudokuGrid"></div>
<div id="numberSelection">
<button>1</button>
<button>2</button>
<button>3</button>
<button>4</button>
<button>5</button>
<button>6</button>
<button>7</button>
<button>8</button>
<button>9</button>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the Sudoku grid and number selection for an intuitive and responsive design.
#sudokuGrid {
display: grid;
grid-template-columns: repeat(9, 1fr);
gap: 2px;
max-width: 90vw;
margin: 0 auto;
}
#sudokuGrid div {
width: 10vw;
height: 10vw;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
font-size: 2em;
}
#numberSelection {
display: flex;
justify-content: center;
gap: 10px;
margin-top: 20px;
}
#numberSelection button {
font-size: 1em;
padding: 10px;
}
Step 3: JavaScript Logic
Implement basic game logic, including grid generation, number selection, and a simple method for checking if the player’s solution is correct.
document.addEventListener('DOMContentLoaded', () => {
const sudokuGrid = document.getElementById('sudokuGrid');
const numberButtons = document.querySelectorAll('#numberSelection button');
let selectedNumber = 1;
// Generate the Sudoku grid
for (let i = 0; i < 81; i++) {
const cell = document.createElement('div');
cell.addEventListener('click', () => {
cell.textContent = selectedNumber; // Fill in the selected number
});
sudokuGrid.appendChild(cell);
}
// Handle number selection
numberButtons.forEach(button => {
button.addEventListener('click', () => {
selectedNumber = button.textContent;
});
});
// TODO: Implement logic for generating puzzles and checking solutions
});
Game mechanics and features
Sudoku Grid: This is a 9×9 grid where players input numbers. The row, column, and subgrid of a 3×3 must contain all numbers from 1 to 9.
Number Selection: Players select a number, then tap on the grid cell where they want to place it.
Difficulty Levels: Implement varying difficulty levels by controlling the number of pre-filled cells in the puzzle. Fewer pre-filled cells mean higher difficulty.
Solution Checking: Add functionality to check if the player’s solution is correct. It can range from simple checks for row, column, and subgrid completion to more complex validation against the puzzle’s solution.
Adapted for mobile.
To adapt this game for mobile platforms:
Responsive Design: Ensure the CSS is responsive so the game looks well on various screen sizes. Use relative units like (viewport width) for sizes.
Touch Interactions: Design the interface with touch interactions in mind. Ensure buttons and grid cells are large enough to tap.
Frameworks: Consider using mobile development frameworks like React Native, which allow you to write mobile apps using JavaScript and React, leveraging your web-based game logic.
Summary
This basic setup provides a foundation for a Sudoku game, focusing on web technologies that can be adapted for mobile. The key aspects include:
We are creating an interactive grid.
They allow number selection.
They lay the groundwork for generating puzzles and checking solutions.
Further development would involve:
I am adding puzzle-generation algorithms.
We implement varying difficulty levels.
We are enhancing the user interface for a more engaging mobile experience.
Basic Concept of Sudoku
Sudoku is a puzzle game consisting of a 9×9 grid divided into 3×3 subgrids. The goal is to fill every row, column, and subgrid with numbers from 1 to 9, ensuring no number repeats in any row, column, or subgrid.
How Game Setup Works
HTML Structure: This is like laying out a game board. We create a big square (#sudokuGrid) divided into 81 smaller squares for the numbers. There’s also a separate section (#numberSelection) with buttons for each number from 1 to 9 that you can choose to fill into the grid.
CSS Styling: Consider this decorating our game board and number buttons to make them friendly and easy to use. We ensure the 9×9 grid fits nicely on the screen and that the number buttons are high enough to tap on mobile devices.
JavaScript Logic:
Creating the Grid: We fill our big square with 81 smaller squares, preparing them to hold the numbers for the Sudoku game.
Selecting Numbers: When you tap a number button, the game remembers that number. Then, when you tap on one of the smaller squares in the grid, it puts your selected number into that square.
Game Play: The idea is to tap the numbers and then tap the grid to fill in the numbers, solving the Sudoku puzzle by following its rules.
Gameplay mechanics
Filling the Grid: You choose a number, then tap on a cell in the Sudoku grid to fill that number in. The challenge comes from figuring out where each number should go.
Checking for Correctness: Although not detailed in the basic setup, a complete game would include a way to check if your solution is correct, ensuring you’ve followed Sudoku rules.
Adapted for mobile.
When making a game like this for mobile:
Touch Friendly: The interface needs to be easy to interact with on a touchscreen, meaning buttons and grid cells should be large enough to tap accurately.
Responsive Design: The game should look appropriate on different screen sizes, adjusting the layout as needed for a clear, playable experience on phones and tablets.
Learning Takeaway
Creating a Sudoku game involves:
I am setting up a grid for the puzzle.
They allow number selection for solving the puzzle.
I am coding the logic to handle these interactions.
It’s a fun way to learn programming basics, from designing the interface to implementing game logic. The principles are similar for web or mobile, but mobile development may require additional considerations for touch interactions and screen sizes.
Leave a Reply