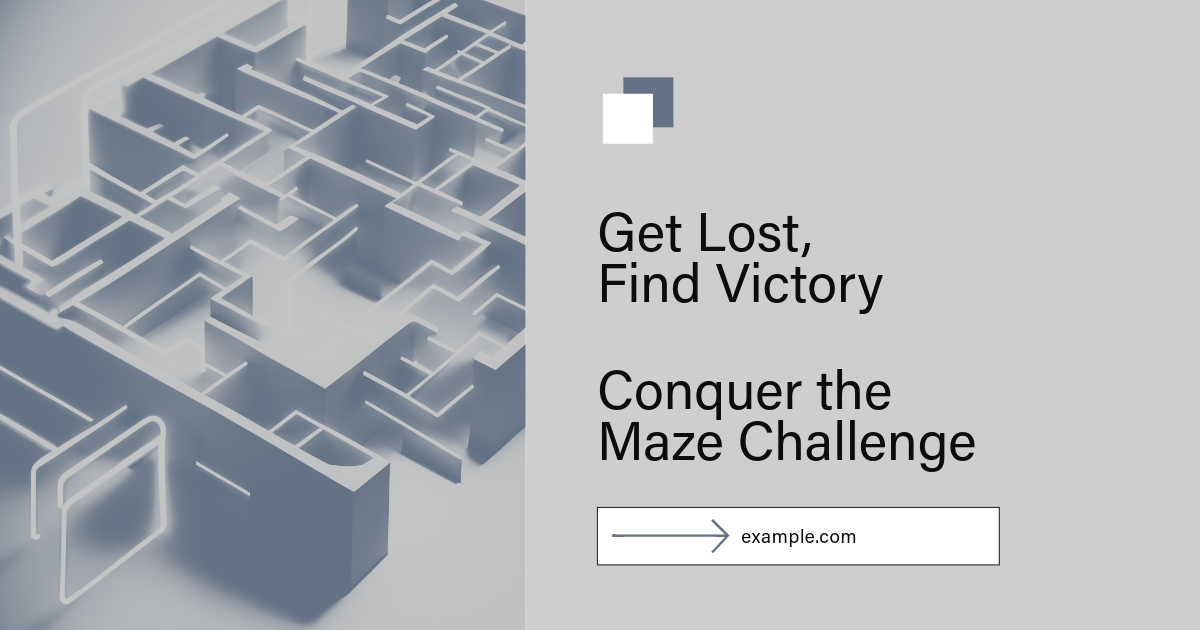
“Maze Runner” games involve creating a maze through which players navigate a character to its exit. This explanation focuses on a conceptual web version that could later be adapted for mobile devices, including swiping or tilting controls. For simplicity, we’ll outline primary navigation using keyboard arrows.
Step 1: HTML Structure
Set up the game’s HTML structure, including a container for the maze and a placeholder for the player character.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Maze Runner Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="mazeContainer">
<!-- Maze and player will be generated dynamically -->
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Maze and player styles. We’ll keep it simple with square blocks for walls and colored blocks for the players.
#mazeContainer {
width: 400px;
height: 400px;
position: relative;
background-color: #eee;
margin: auto;
}
.wall {
width: 40px;
height: 40px;
background-color: #333;
position: absolute;
}
.player {
width: 38px;
height: 38px;
background-color: blue;
position: absolute;
}
Step 3: JavaScript Logic
Using basic game logic, create a maze, place the player, and enable keyboard navigation.
document.addEventListener('DOMContentLoaded', () => {
const mazeContainer = document.getElementById('mazeContainer');
let playerPosX = 0; // Player's start position X
let playerPosY = 0; // Player's start position Y
// Example maze layout: 1 for wall, 0 for path
const maze = [
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1],
[1, 0, 0, 0, 1, 0, 0, 0, 0, 1],
[1, 0, 1, 0, 1, 0, 1, 1, 0, 1],
// More rows defining the maze...
[1, 0, 0, 0, 0, 0, 0, 0, 0, 1],
[1, 1, 1, 1, 1, 1, 1, 1, 1, 1]
];
function drawMaze() {
maze.forEach((row, y) => {
row.forEach((cell, x) => {
if (cell === 1) {
const wall = document.createElement('div');
wall.className = 'wall';
wall.style.left = `${x * 40}px`;
wall.style.top = `${y * 40}px`;
mazeContainer.appendChild(wall);
}
});
});
}
function placePlayer() {
const player = document.createElement('div');
player.className = 'player';
player.style.left = `${playerPosX}px`;
player.style.top = `${playerPosY}px`;
mazeContainer.appendChild(player);
document.addEventListener('keydown', (e) => {
// Basic movement: up, down, left, right
switch(e.key) {
case 'ArrowUp': playerPosY -= 40; break;
case 'ArrowDown': playerPosY += 40; break;
case 'ArrowLeft': playerPosX -= 40; break;
case 'ArrowRight': playerPosX += 40; break;
}
player.style.left = `${playerPosX}px`;
player.style.top = `${playerPosY}px`;
});
}
drawMaze();
placePlayer();
});
Game mechanics explained
Maze Generation: The maze is represented by a 2D array, where 1 denotes a wall, and 0 denotes a path. The drawMaze function reads this array and creates a visual maze.
Player Movement: The player starts in the top-left corner of the maze. The player can move up, down, left, or right using the arrow keys. Each press moves the player one block in the maze.
Objective: The player needs to navigate through the maze to reach an exit point. This is not included in this basic setup but represented as a particular block or area in the maze.
Enhancements for Mobile Adaptation
For a mobile version, you could replace the keyboard controls with:
Swipe Gestures: Detect swipes in different directions to move the player through the maze.
Tilting Controls: Use device orientation events to move the player based on how the device is tilted.
Summary
This setup offers a foundational structure for a Maze Runner game, focusing on maze generation and basic player movement. Expanding this foundation with mobile-friendly controls, complex mazes, obstacles, and rewards will create a more engaging and challenging game.
Setting up the game board
HTML Structure: Think of this as outlining your game on paper. A very large square (#mazeContainer) represents the maze where the game happens. Inside this square are walls, paths, and a small square (player) that you move around in.
CSS Styling: This step is like coloring and decorating your drawing. The big square (maze) gets a light background, the walls within the labyrinth are dark blocks, and the player is a blue block. The size of everything ensures that the player and walls fit nicely into the maze.
JavaScript Logic: We’re adding rules and actions to our game. It is where the game comes to life.
Drawing the Maze
Creating the Maze: We use a simple numbers map to design our maze. One represents a wall, and 0 represents a path. The maze is drawn row by row, placing a dark block (wall) where there’s a one and leaving space open where there’s a 0.
Place the player.
Starting Position: We put our player (a blue block) at the beginning of the maze. It is the starting point from where you’ll navigate through the maze.
Moving around
Listening for Key Presses: The game listens for when you press the arrow keys on your keyboard. Each key press corresponds to a direction: up, down, left, or right.
Making a Move: When you press an arrow key, the player moves one block in that direction within the maze. For example, pressing the “ArrowUp” key moves the player up one block.
Playing the game
Navigating the Maze: Your task is to move the player through the maze, from the starting point to an exit. You need to avoid walls and find the path through.
Reaching the Exit: In this basic setup, the goal is to navigate successfully, but we don’t define an exit point. In a complete game, reaching the exit is your objective.
Understanding the Code
DrawMaze Function: This part of the code looks at the maze design (our map of numbers) and creates the visual representation on the screen by placing walls according to the map.
PlacePlayer Function: This sets the player in the maze and updates his position based on the arrow keys you press.
Conclusion
In this Maze Runner game, you use code to create a maze full of walls. You place a player at the start, and then use the keyboard to navigate through the maze. It’s like a digital puzzle where you control a character trying to find its way out.
For reference links and further reading, while I can’t browse the internet for live links, I suggest looking into:
- Unity Game Development Tutorials : Unity’s official learning resources are great for beginners and advanced developers interested in game design, including maze or labyrinth games.
Leave a Reply