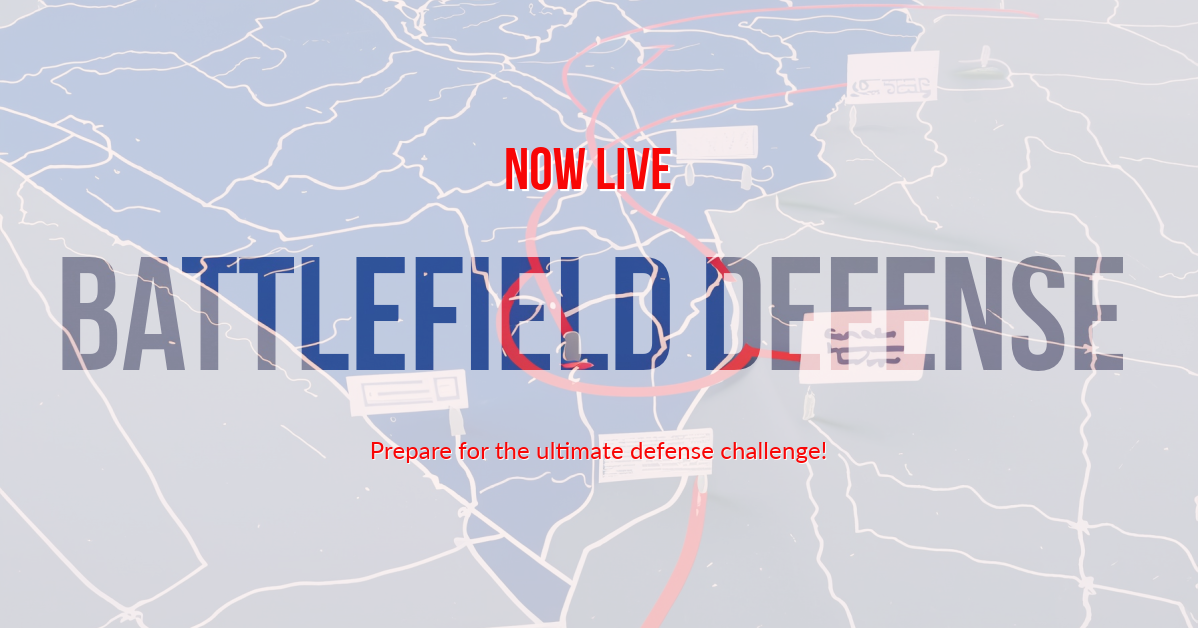
Creating a simple tower defense game involves players placing defense towers on the map to stop waves of enemies from reaching an endpoint. This basic outline will help you understand how to start building such a game using HTML, CSS, and JavaScript. The game we’ll outline will include placing towers, generating enemies, and a primary attack mechanism.
Step 1: HTML Structure
Define the game area, a place for the player’s score and currency, and buttons for selecting different towers.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Tower Defense Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="score">Score: 0</div>
<div id="currency">Currency: 100</div>
<div id="gameArea"></div>
<button id="basicTower">Place Basic Tower ($10)</button>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the game area and other elements for clarity and visual appeal.
#gameArea {
width: 600px;
height: 400px;
position: relative;
background-color: #ddd;
margin: auto;
}
#score, #currency {
text-align: center;
}
button {
display: block;
margin: 10px auto;
}
Step 3: JavaScript Logic
Implement tower placement, enemy generation, and basic gameplay.
document.addEventListener('DOMContentLoaded', () => {
const gameArea = document.getElementById('gameArea');
const scoreElement = document.getElementById('score');
const currencyElement = document.getElementById('currency');
let score = 0;
let currency = 100;
document.getElementById('basicTower').addEventListener('click', () => {
if (currency >= 10) {
// Allow the player to place a tower if they have enough currency
placeTower();
currency -= 10;
updateUI();
}
});
function placeTower() {
// Simplified: Place a tower at a fixed position
const tower = document.createElement('div');
tower.classList.add('tower');
tower.style.position = 'absolute';
tower.style.width = '40px';
tower.style.height = '40px';
tower.style.backgroundColor = 'black';
tower.style.top = '180px'; // Example fixed position
tower.style.left = '100px';
gameArea.appendChild(tower);
// Add attack functionality or event listeners as needed
}
function updateUI() {
scoreElement.textContent = `Score: ${score}`;
currencyElement.textContent = `Currency: ${currency}`;
}
// Add functions for enemy generation and game logic
});
Game mechanics explained
Placing Towers: Players can place towers in the game area to defend themselves against enemies. Towers cost currency, which players earn by defeating enemies.
Enemy Waves: Enemies should spawn at intervals and move across the game area toward a goal. If an enemy reaches the endpoint, the player loses health or points.
Combat: Towers automatically attack enemies within range. Implementing a primary attack mechanism can start with towers “hitting” any enemy that passes within a certain distance.
Scoring and Currency: Players earn points and currency by defeating enemies. These can be used to place more towers or upgrade existing ones.
Enhancements
To further develop the game, consider adding:
Different Tower Types: Offer a variety of towers with unique abilities or attack types.
Enemy Varieties: Introduce different enemy types with unique strengths and weaknesses.
Level Progression: Design levels with increasing difficulty, including stronger enemies and more complex maps.
Tower Upgrades: Allow players to upgrade towers for improved defense capabilities.
Summary
As a tower defense game, this setup focuses on placing towers, creating enemies, and generating a simple scoring system. A number of advanced mechanics will be introduced in the game over time, such as varying types of towers and enemies, level progression, and upgrades.
HTML: Setting Up the Game Space
Game Area: We have a “game area,” like our playground, where all the action happens. It’s a big box on the web page.
Score and Currency: Two small boxes at the top show the player’s score (“Score: 0”) and how much money they have (“Currency: 100”) to spend on building towers.
Place Tower Button: A button labeled “Place Basic Tower (\$10)” that players can click to place an additional tower in the game area, costing them \$10 from their currency.
CSS: Make It Look Nice
Game Area Styling: The “game area” is styled to be a transparent, visible rectangle on the screen where players can see everything happening.
Score and Currency Styling: The score and currency are displayed clearly so players can always see their progress and how much money they have.
Button Styling: The button to place another tower stands out so players know where to click when adding a tower.
JavaScript: The Game’s Brain
This is where we define what happens in the game, how players interact with it, and how it responds.
Starting the Game: The game takes place when the webpage loads (DOMContentLoaded).
Placing towers:
When the player clicks the “Place Basic Tower” button, the game checks if the player has enough currency (\$10).
If they do, another tower (a black box) is placed in the game area at a fixed position.
The player’s currency is reduced by $10 because they’ve spent that on the tower.
Updating the Score and Currency:
Whenever something affects the score or currency, these displays are updated to show the updated values.
For now, the currency changes only when the first tower is placed.
What’s missing? More game mechanics
This basic setup covers placing towers and updating scores, but a full tower defense game also needs:
Generating Enemies: Code to create enemies that move across the game area, trying to get from one side to another.
Towers Attacking: Mechanisms for towers to automatically attack enemies when they come close enough.
Winning and Losing Conditions: Rules for how the player wins (e.g., surviving a certain number of waves of enemies) or loses (e.g., too many enemies cross the game area).
Simplified explanation for students.
Imagine you’re playing a game where you must stop cartoon monsters from crossing your backyard. You can buy toy soldiers and place them in the yard with your allowance money. Every time you click a button, you use some of your allowances to purchase another toy soldier that you think will help combat the monsters. The game keeps track of how many monsters you’ve stopped and how much allowance money you have left to spend on more soldiers.
This code is like instructions for setting up and playing that game on a computer or phone. It tells it how to make the yard, how much allowance money you start with, and what happens when you put down your first toy soldier.
For reference links and further exploration, while I can’t provide current live internet content, consider the following types of resources:
- Unity Learn : Unity’s official learning platform offers tutorials and projects specifically for game development, including tower defense games.
Leave a Reply