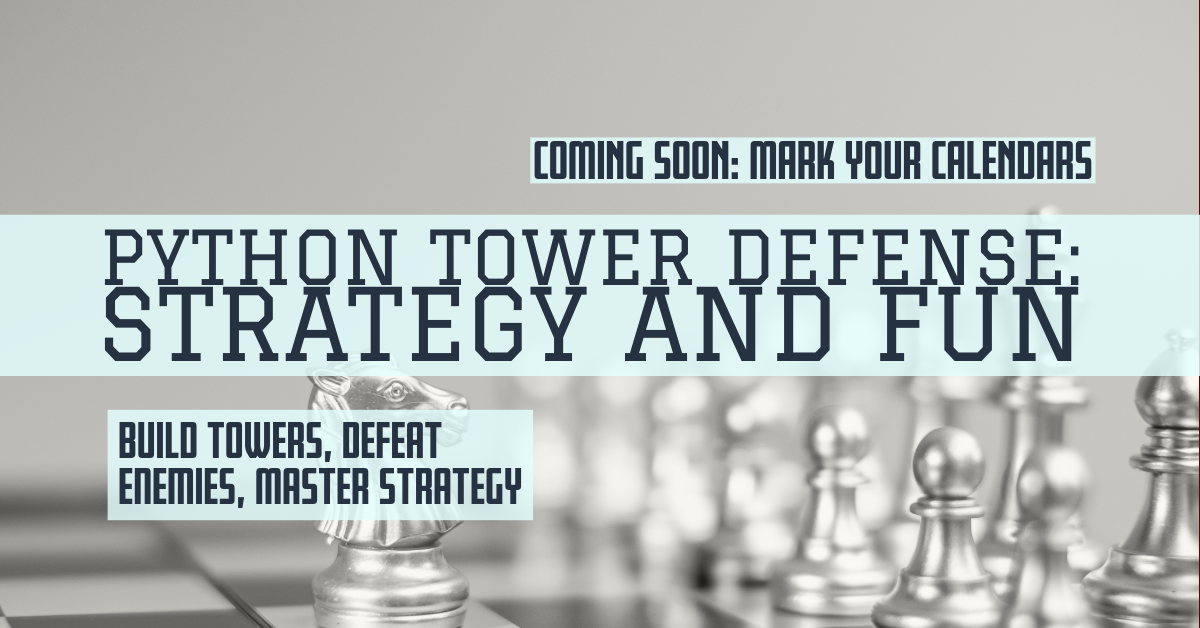
Tower Defense Game Plan:
Language: Python is a versatile language ideal for creating simple games.
Library: pygame for handling graphics, events, and game logic.
Game elements:
A path along which enemies will travel.
Towers that the player can place along the path.
Waves of enemies trying to pass through the path.
Features:
Players can place towers at strategic points.
Towers automatically shoot at enemies within range.
Different types of enemies and towers, each with unique attributes.
A system to manage enemy waves.
Scoring is based on how well the player defends against the waves.
User interface:
A window displaying the game with the path, towers, and enemies.
Display the player’s score, resources, or tower information.
Tower Defense Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
TOWER_SIZE = 40
ENEMY_SIZE = 30
WHITE = (255, 255, 255)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Tower Defense Game')
# Tower setup
towers = []
# Enemy setup
enemies = []
enemy_frequency = 2000 # milliseconds
last_enemy = pygame.time.get_ticks()
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.MOUSEBUTTONDOWN:
tower_x, tower_y = event.pos
towers.append(pygame.Rect(tower_x - TOWER_SIZE // 2, tower_y - TOWER_SIZE // 2, TOWER_SIZE, TOWER_SIZE))
# Generate enemies
current_time = pygame.time.get_ticks()
if current_time - last_enemy > enemy_frequency:
enemy_x = random.randint(0, SCREEN_WIDTH - ENEMY_SIZE)
enemies.append(pygame.Rect(enemy_x, -ENEMY_SIZE, ENEMY_SIZE, ENEMY_SIZE))
last_enemy = current_time
# Move enemies
for enemy in enemies[:]:
enemy.y += 2
if enemy.y > SCREEN_HEIGHT:
enemies.remove(enemy)
# Drawing everything
screen.fill(WHITE)
for tower in towers:
pygame.draw.rect(screen, GREEN, tower)
for enemy in enemies:
pygame.draw.rect(screen, RED, enemy)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Tower Defense game will start.
This code provides a basic tower defense game where players can click to place towers on the screen. Enemies spawn randomly and move down the screen. The game ends if the player closes the window. Note that this version is simplified without actual tower shooting mechanics or wave management, but it lays the foundation for a tower defense game.
Importing libraries
Import a pygame
Import random
pygame is a Python library used for game development.
Random generates random positions for enemies.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Other constants for tower and enemy size, colors
Initializes Pygame modules, which are necessary for game development.
Set constants for screen dimensions, tower size, enemy size, and colors.
Creating the game window
Screen = pygame. Display.set_mode(SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Tower Defense Game’)
Sets up the game window with the specified width and height.
The window’s title is ‘Tower Defense Game.’
Setting up towers and enemies
Towers = []
Enemies = []
Towers will store the positions of towers placed by the player.
Enemies store enemies’ positions and movements.
Game loop
While running:
# Event handling, generating enemies, updating the game state…
The main loop continuously updates the game state and redraws the screen.
Handling Events (Placing Towers and Quitting)
For events in pygame. Event.get():
# Code for event handling (placing towers and quitting the game)
Listen for mouse clicks to place towers and quit events to end the game.
Generating and Moving Enemies
# Code to generate new enemies at intervals
# Code to move each enemy down the screen
Adds new enemies at random horizontal positions at set intervals.
Move each enemy down the screen to simulate an advancing enemy.
Drawing Towers and Enemies
Screen.fill(WHITE)
# Drawing code for towers and enemies
He clears the screen before drawing towers and enemies.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(60)
Updates the entire screen with what has been drawn.
It keeps the game running at 60 frames per second for smooth gameplay.
Playing the game is leaving.
Pygame.quit()
It closes the game window and ends the game.
Learning Points for Students
Game Loop: Understanding the central loop that handles events, updates game state, and renders graphics.
Event Handling: How to respond to user inputs like mouse clicks and system events.
Lists and Loops: Using lists to manage game objects (towers and enemies) and loops to update/render them.
Basic Game Mechanics: Introducing concepts like enemy spawning, player interaction (placing towers), and simple movement mechanics.
Reference Links to Include:
Python Official Documentation:
- Purpose: Provides essential Python syntax and library references.
Pygame Documentation:
- Purpose: Crucial for game development, especially if you’re using Pygame for the game’s graphical interface.
GitHub Projects for Tower Defense in Python:
- Purpose: Offers examples and inspiration from existing Tower Defense games developed in Python.
Stack Overflow Python Game Development:
- Purpose: A great resource for solving common issues and getting advice on game development with Python.
Leave a Reply