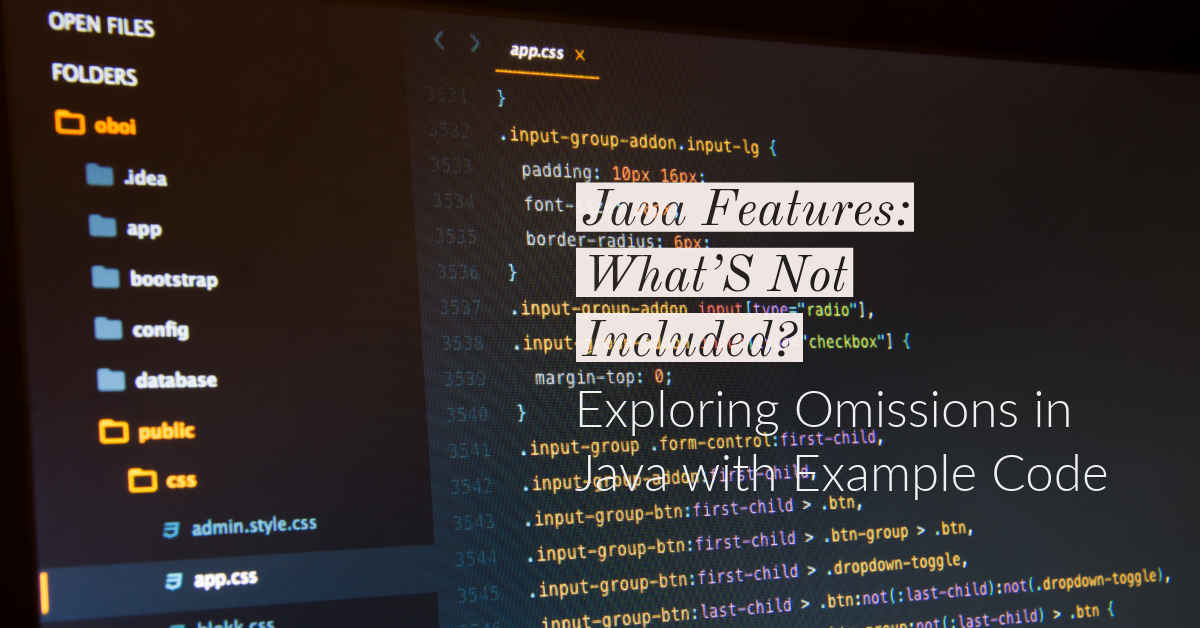
Many features make Java a popular choice among developers worldwide. In addition to object-oriented programming and multithreading, this programming language offers robustness and security. Among the features that aren’t inherent to Java, multiple-class inheritance is notable for lack of direct support.
Multiple Inheritance
Multiple inheritance allows a class to inherit behavior and attributes from more than one parent class. This feature is available in some programming languages but not directly supported in Java due to the complexity and potential for ambiguity it introduces (such as the Diamond Problem).
Example Code Demonstrating the Lack of Multiple Inheritance:
Java does not allow classes to extend to more than one class. For instance, consider two parent classes:
class Parent1 {
void show() {
System.out.println("This is Parent1");
}
}
class Parent2 {
void display() {
System.out.println("This is Parent2");
}
}
Attempting to create a child class that inherits from both Parent1 and Parent2 directly would not be possible.
// This is not possible in Java - it will result in a compile-time error
class Child extends Parent1, Parent2 {
// Attempting to inherit from multiple classes
}
How Java Addresses Multiple Inheritance Lack
Instead of multiple inheritance, Java uses interfaces to achieve similar results without complexity. Interfaces define methods that various classes can implement, allowing objects to inherit behavior from multiple sources through interfaces.
Example of Interfaces:
interface Parent1 {
void show(); // Interface method
}
interface Parent2 {
void display(); // Interface method
}
// A class can implement multiple interfaces
class Child implements Parent1, Parent2 {
public void show() {
System.out.println("This is Parent1's show method");
}
public void display() {
System.out.println("This is Parent2's display method");
}
}
public class Test {
public static void main(String[] args) {
Child c = new Child();
c.show();
c.display();
}
}
In this example, the Child class implements both the Parent1 and Parent2 interfaces, thereby incorporating behavior from both interfaces without directly inheriting from the two classes.
As a conclusion
Multiple inheritance is not a supported feature of Java, despite the fact that the language is rich in features that support robust and secure object-oriented programming. The Java programming language provides an alternative due to its flexible and powerful interfaces. It is possible to create courses without relying on multiple inheritance when using this type.
Reference Links to Include:
Oracle’s Java Documentation:
- For official information on Java’s capabilities and limitations.
Java Language and Environment Comparisons:
- Offers insights into Java’s features compared to other programming languages, highlighting what Java might lack.
- Suggested Search: “Comparing Java with other programming languages”
GitHub Java Projects:
- For real-world examples of how developers work around Java’s limitations or highlight missing features.
Stack Overflow for Java Programming:
- A resource for discussions related to Java’s features, including questions about what Java may not support.
Leave a Reply