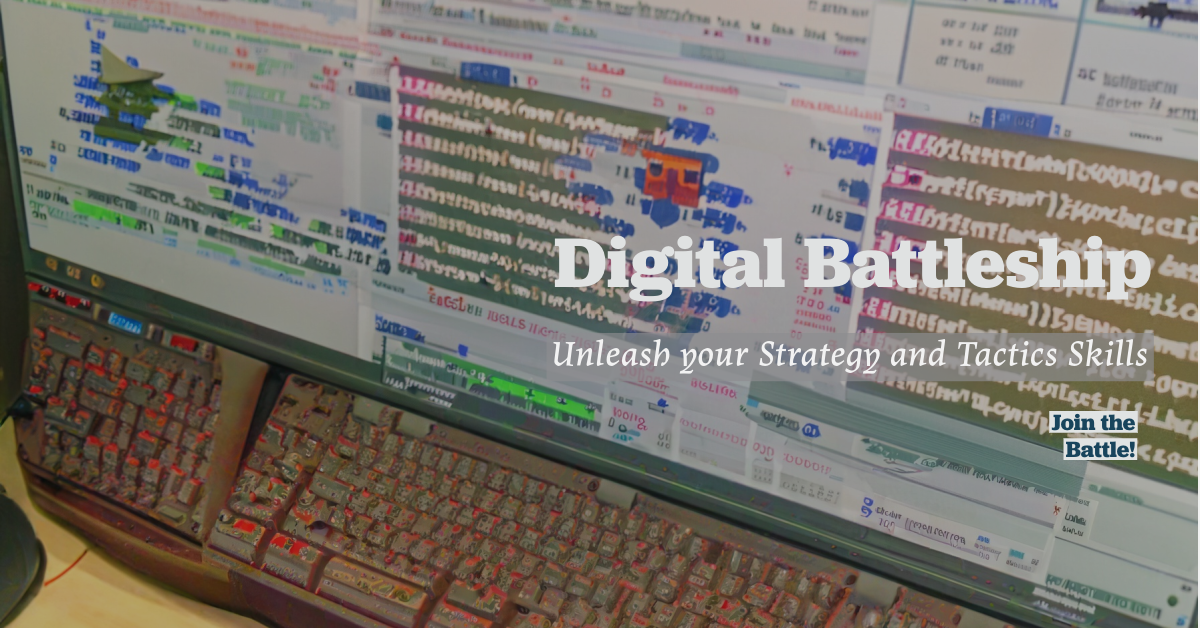
Battleship Game Plan:
Language: Python, ideal for grid-based logic and user interaction.
Game elements:
Two grids (one for each player): one to place their ships and one to record the opponent’s shots.
Different types of vessels of varying sizes.
Features:
Players place their boats on their grids at the start of the game.
Players guess the location of opponents’ ships on the grid.
Marking hits and misses on the grid.
Players continue to play until one of their ships is destroyed.
User interaction:
Text-based input for placing ships and making guesses.
Text output showing grid status and game progress.
Battleship Game Code:
def print_grid(grid):
for row in grid:
print(" ".join(row))
def initialize_grid():
return [["O" for _ in range(8)] for _ in range(8)]
def place_ship(grid, ship_size):
placed = False
while not placed:
row = int(input("Enter row to place {}-size ship: ".format(ship_size)))
col = int(input("Enter column to place {}-size ship: ".format(ship_size)))
direction = input("Enter direction (h for horizontal, v for vertical): ")
if direction == 'h' and col + ship_size <= 8:
for i in range(ship_size):
grid[row][col + i] = "S"
placed = True
elif direction == 'v' and row + ship_size <= 8:
for i in range(ship_size):
grid[row + i][col] = "S"
placed = True
else:
print("Invalid placement. Try again.")
def player_turn(grid, opponent_grid):
hit = False
while not hit:
row = int(input("Enter row to hit: "))
col = int(input("Enter column to hit: "))
if opponent_grid[row][col] == "S":
print("Hit!")
grid[row][col] = "X"
opponent_grid[row][col] = "X"
hit = True
elif opponent_grid[row][col] == "O":
print("Miss.")
grid[row][col] = "-"
opponent_grid[row][col] = "-"
hit = True
else:
print("You already tried this spot. Try again.")
def check_win(grid):
for row in grid:
if "S" in row:
return False
return True
# Game setup
player1_grid = initialize_grid()
player2_grid = initialize_grid()
player1_shots = initialize_grid()
player2_shots = initialize_grid()
# Place ships
for size in [2, 3]: # Example with two ships of size 2 and 3
print("Player 1, place your ships.")
place_ship(player1_grid, size)
print("Player 2, place your ships.")
place_ship(player2_grid, size)
# Game loop
game_over = False
while not game_over:
print("Player 1's turn")
player_turn(player1_shots, player2_grid)
if check_win(player2_grid):
print("Player 1 wins!")
game_over = True
break
print("Player 2's turn")
player_turn(player2_shots, player1_grid)
if check_win(player1_grid):
print("Player 2 wins!")
game_over = True
How to run:
Save this script as a .py file.
Run the script using Python, and the Battleship game will start.
Follow the on-screen prompts to place ships and hit opponents’ ships.
This code allows two players to play Battleship against each other. Each player places his or her ships on a grid and guesses the location of the other players’ ships. The game ends when one player’s ship sinks. The code includes functions to set up the grid, place ships, take turns, and check for a win.
Defining Helper Functions
Print_grid Function:
Def print_grid(grid):
For each row in the grid:
Print(” “.join(row)”)
This function prints the game grid. Grid rows are joined into strings and printed.
Initialize_grid Function:
Def initialize_grid():
Return [[“O” for in range(8)] for in range(8)]
Initializes an 8×8 grid filled with “O” (representing water).
Place_ship Function:
Def place_ship(grid, ship_size):
# Code to place a ship of a given size on the grid
Asks the player to enter the starting position and direction (horizontal or vertical) to place a ship.
Check if the ship placement is valid (within the grid bounds).
Player_turn Function:
Def player_turn(grid, opponent_grid):
# Code to handle a player’s turn to guess the opponent’s ship location
Allows a player to guess a location on the opponent’s grid.
Marks hits (“X”) and misses (“-“) on their tracking grid.
Check_win Function:
Def check_win(grid):
# Code to check if all ships on a grid have been sunk
Check if any ships (“S”) are left on a grid. If not, the opponent wins.
Setting Up the Game
Initialized four grids: two for each player’s ship and two to track their shots.
Players place ships of specified sizes on their grids.
The Game Loop
While not game_over:
Player 1 and Player 2 alternate turns
The game alternates turns between Player 1 and Player 2.
After each turn, it checks if the opponent’s ships have all been sunk.
Learning Points for Students
Multidimensional Lists: The game uses 2D lists to represent grids.
Nested Loops: The code uses loops within loops to interact with the grid.
Function Calls: Demonstrates how to define and use functions for specific tasks.
User Input and Validation: Handling and validating user input against game rules.
Conditional Logic: Uses if-else statements to implement game logic, like checking for hits, misses, and win conditions.
Reference Links to Include:
Python Official Documentation:
- Purpose: To provide a solid foundation in Python for your readers.
Pygame Documentation (for game graphics):
- Purpose: If your Battleship game includes graphical elements, Pygame’s documentation is invaluable for readers.
GitHub Python Projects for Battleship:
- Purpose: To offer examples of Battleship games coded in Python, providing inspiration and coding perspectives.
Stack Overflow Python Game Development:
- Purpose: A great resource for solving common issues encountered during game development in Python.
Leave a Reply