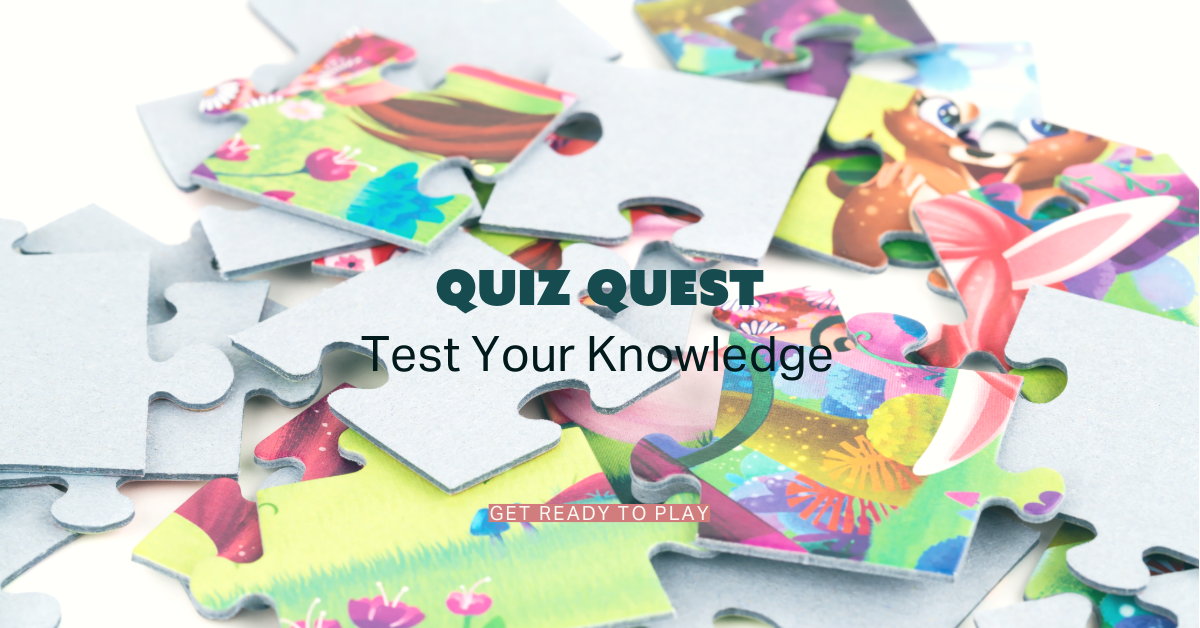
A quiz game is created by setting up a series of questions, presenting them to players, and scoring their answers. Below is a basic framework for a web-based quiz game using HTML for structure, CSS for styling, and JavaScript for quiz logic.
Step 1: HTML Structure
Define the main elements: a container for the quiz, a place to show the questions, options for answers, and a button to submit an answer.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Quiz Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="quizContainer">
<div id="question"></div>
<ul id="answerOptions"></ul>
<button id="submitAnswer">Submit Answer</button>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Style the quiz elements for a clean and engaging interface
#quizContainer {
width: 600px;
margin: auto;
text-align: center;
}
#question {
margin-bottom: 20px;
font-size: 24px;
}
#answerOptions li {
list-style-type: none;
margin: 10px;
padding: 10px;
background-color: #f0f0f0;
cursor: pointer;
}
#submitAnswer {
margin-top: 20px;
padding: 10px 20px;
font-size: 18px;
}
Step 3: JavaScript Logic
The quiz questions must be set up, answers must be selected, and scoring must be implemented.
document.addEventListener('DOMContentLoaded', () => {
const questions = [
{
question: "What is 2+2?",
answers: ["3", "4", "5", "6"],
correctAnswer: "4"
},
{
question: "Who is the current president of the United States?",
answers: ["Barack Obama", "Donald Trump", "Joe Biden", "George Bush"],
correctAnswer: "Joe Biden"
}
// Add more questions as needed
];
let currentQuestionIndex = 0;
let score = 0;
const questionElement = document.getElementById('question');
const answerOptionsElement = document.getElementById('answerOptions');
const submitAnswerButton = document.getElementById('submitAnswer');
function displayQuestion() {
const currentQuestion = questions[currentQuestionIndex];
questionElement.textContent = currentQuestion.question;
answerOptionsElement.innerHTML = ''; // Clear previous options
currentQuestion.answers.forEach(answer => {
const li = document.createElement('li');
li.textContent = answer;
li.addEventListener('click', () => selectAnswer(answer));
answerOptionsElement.appendChild(li);
});
}
function selectAnswer(answer) {
const correctAnswer = questions[currentQuestionIndex].correctAnswer;
if (answer === correctAnswer) {
score++;
alert("Correct!");
} else {
alert("Wrong answer.");
}
currentQuestionIndex++;
if (currentQuestionIndex < questions.length) {
displayQuestion();
} else {
alert(`Quiz finished! Your score: ${score}`);
// Optionally, restart the quiz or navigate to a results page
}
}
submitAnswerButton.addEventListener('click', displayQuestion);
displayQuestion(); // Start with the first question
});
Game mechanics explained
Displaying Questions: The first question and answer options are shown when the game loads. Players select their answer by clicking on one of the options.
Selecting Answers: When a player chooses an answer, the game checks its correctness. Players are immediately informed, and the score is updated accordingly.
Progressing Through Questions: After answering a question, the game moves to the next one until all questions have been answered.
Scoring and Feedback: The game keeps track of the player’s score, providing a final tally.
Enhancements
To further develop the game, consider adding:
Timer: Implement a countdown for each question to add urgency.
Categories: Offer questions in different categories for players to choose from.
Difficulty Levels: Adjust the difficulty of the questions based on the player’s progress or choice.
High Score Tracking: Keep track of high scores using local storage or a backend database.
Step 1: Setting Up the Stage
Imagine setting up a game board:
Structure of HTML: It’s like drawing a board with questions, options, and a submit button. The game board (#quizContainer) shows one question at a time, several answer options, and a “Submit Answer” button.
Step 2: Make it Look Stylish
Now, let’s paint and decorate our board:
CSS Styling: This step is like choosing colors and styles for our game board, making sure the questions, answer options, and buttons are visible and appealing. We create the questions to stand out, give the answer options an excellent background, and make the submit button bold and easy to click.
Step 3: Add Magic (Game Logic).
Here’s where we bring our game to life:
JavaScript Logic: Think of this as programming the game rules. We start with a list of questions, each having a correct answer. The game shows these questions one by one. When you pick an answer and hit “Submit,” the game tells you if you’re right or wrong and then moves on to the next question. It is your goal to answer as many questions as possible correctly.
How to play?
View the Question: A question appears on the game board with several answer options.
Choose Your Answer: Click on your favorite answer. It’s like choosing a card with the correct answer written on it.
Submit Your Answer: Press the “Submit Answer” button to see if you’re correct.
Score Points: You get a point if you pick the correct answer. The game then shows you the next question.
Finished the Game: The game displays your total score after answering all questions, like how many questions you answered correctly.
Make it fun
To make the game more interesting, consider adding a timer for each question, creating different categories (like science, history, or pop culture), or setting up difficulty levels. You could also keep track of high scores to add competition.
For reference links and additional resources, while I can’t provide current live internet content, I recommend exploring:
- Unity Learning Materials for tutorials on game development, including quiz and educational game creation.
- Game Development on Stack Overflow for community-driven advice and troubleshooting.
- Educational Technology Journals or websites for insights into the pedagogical aspects of quiz games, which can provide a deeper understanding of engaging players in learning.
Leave a Reply