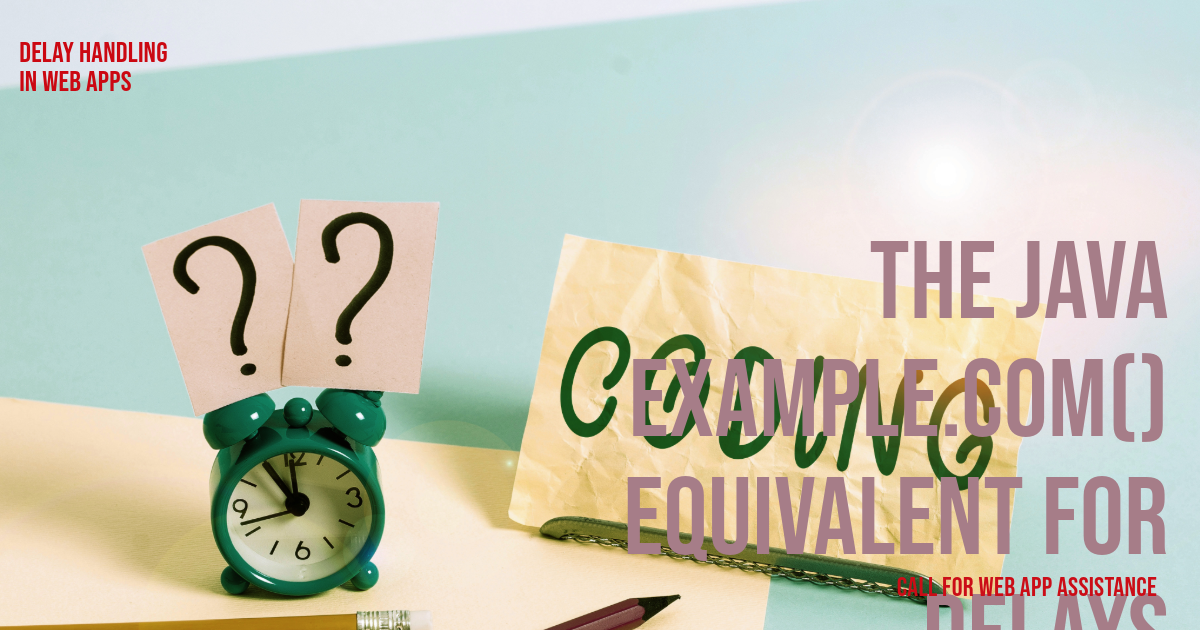
The JavaScript equivalent of Java’s Thread. Sleep () for introducing a delay can be achieved using non-synchronous programming constructs since JavaScript is single-threaded and relies on an event loop for executing asynchronous code. The most common ways to introduce a delay simultaneously in JavaScript are through the setTimeout() function for callback-based delays and Promise combined with async/await syntax for more modern, cleaner asynchronous code.
SetTimeout ()
Using the setTimeout() function, you can delay executing a function by a specified amount of time. However, setTimeout() does not pause code execution. Instead, it schedules a function to run after the delay, allowing the rest of the code to continue executing.
function simulateThreadSleep(milliseconds) {
setTimeout(() => {
console.log("Delay finished");
}, milliseconds);
}
simulateThreadSleep(2000); // Delays the message for 2000 milliseconds (2 seconds)
Using async/await with Promises
For scenarios where you want to write asynchronous code that looks more like synchronous code (similar to how you might use Thread). Sleep() in Java), you can use async/await with Promises. I prefer this approach because it is cleaner and easier to read, especially when chaining together asynchronous operations.
Here’s how to create a sleep-like function in JavaScript:
function sleep(milliseconds) {
return new Promise(resolve => setTimeout(resolve, milliseconds));
}
async function run() {
console.log('Before delay');
await sleep(2000); // Waits for 2 seconds
console.log('After delay');
}
run();
In this example, the sleep function returns a Promise that resolves after a given time. This effectively pausing execution in the run function when awaited without blocking the main thread.
Important considerations
SetTimeout() and the sleep function using Promises are both non-blocking. They do not pause the script’s execution; instead, they allow other operations to run in the background.
The use of async/await allows you to write asynchronous code that resembles synchronous blocking operations, but JavaScript remains single-threaded and non-blocking.
In web environments, these mechanisms are particularly useful since blocking operations would negatively impact the user experience, since application responsiveness is vital.
For reference links, while I can’t generate live internet content, I recommend checking out:
- MDN Web Docs for comprehensive documentation on JavaScript, including best practices for implementing delays and asynchronous functions.
- Stack Overflow for community-driven solutions and discussions on JavaScript timing functions and handling delays effectively.
- Java Script for tutorials and articles that cover JavaScript from the basics to advanced topics, including timing and delay handling techniques.
Leave a Reply