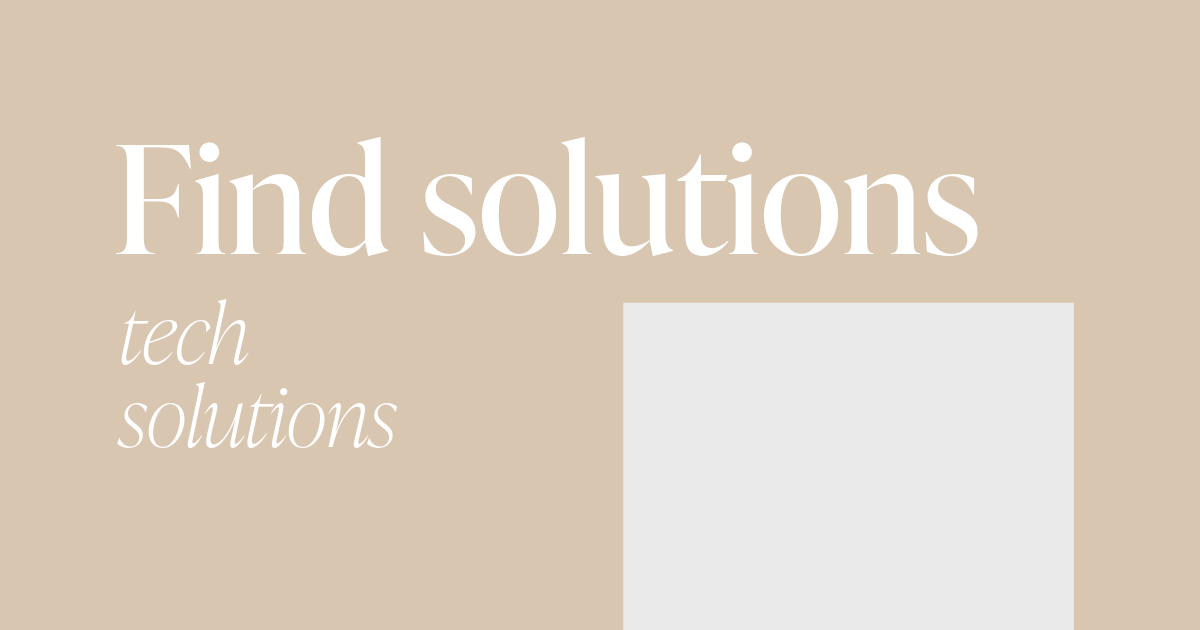
A 406 Not Acceptable response status code in a Spring-based application typically occurs when the server cannot produce a response matching the acceptable values defined in the request’s Accept headers. This often happens in JSON requests when the client expects a specific content type that the server is not configured to return. Here are some common reasons and solutions for troubleshooting a 406 response in Spring when dealing with JSON requests:
Missing or Incorrect Content Negotiation Configuration
Ensure that Spring is configured to handle and produce the content type your client expects. Spring MVC needs to be configured for JSON responses to use a MappingJackson2HttpMessageConverter that converts response objects to JSON.
Solution: Make sure your project, such as Jackson, includes the necessary dependencies for JSON serialization and that Spring is configured to use it.
<!-- If using Maven, ensure you have the Jackson dependency -->
<dependency>
<groupId>com.fasterxml.jackson.core</groupId>
<artifactId>jackson-databind</artifactId>
<version>YourJacksonVersion</version>
</dependency>
Incorrect @RequestMapping or @GetMapping configuration.
The @RequestMapping or @GetMapping annotation in your controller method should be configured to produce the content type your client accepts (application/JSON in most cases).
Solution: Use the produce attribute of the @RequestMapping or @GetMapping annotation to specify the content type.
@GetMapping(path = "/yourEndpoint", produces = MediaType.APPLICATION_JSON_VALUE)
public ResponseEntity<YourObject> getYourObject() {
...
}
Accept header mismatch
The client’s Accept header does not match any content type the server will produce. This can happen if the client specifies a particular content type or if there’s a typo.
Solution: Check the Accept header sent by the client and ensure it aligns with what the server can produce, typically application/JSON.
Configuration Issue or the absence of Message Converter
Spring might need to be correctly configured to convert the response to JSON format due to a missing message converter or misconfiguration.
Solution: Ensure you have correctly configured message converters in your Spring application context. If you use Spring Boot, most of this configuration is auto-configured for you. Otherwise, you might need to add MappingJackson2HttpMessageConverter to your computer’s configuration.
@Override
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) {
converters.add(new MappingJackson2HttpMessageConverter());
}
Content negotiation and configuration
Spring MVC’s content negotiation could exclude application/JSON as an acceptable response format.
Solution: Verify and adjust your content negotiation configuration. Much of Spring Boot is auto-configured, but you can still customize it in application.properties or application.yml or through a WebMVCConfigurer implementation.
Debugging steps
Check Dependencies: Ensure that all necessary dependencies are included in your project.
Review Configuration: Look over your Spring MVC configuration for message converters or content negotiation issues.
Inspect Client Requests: Verify that the client’s request headers, especially the Accept header, are correctly set to accept the content types your server is configured to produce.
Examine Annotations: Ensure your controller methods are correctly annotated to produce the expected content type.
By systematically checking these areas, you can identify and resolve the cause of the 406 Not Acceptable status code in your Spring application.
For reference links and additional insights, while I can’t provide current live internet content, consider exploring:
- Spring Official Documentation for in-depth understanding and official solutions related to Spring framework issues, including error 406.
- Stack Overflow for community-driven discussions and solutions on encountering and resolving the JSON request error 406 in Spring applications.
- Baeldung or similar educational sites for Spring and Java that often contain detailed articles and tutorials addressing common issues like content negotiation problems.
Leave a Reply