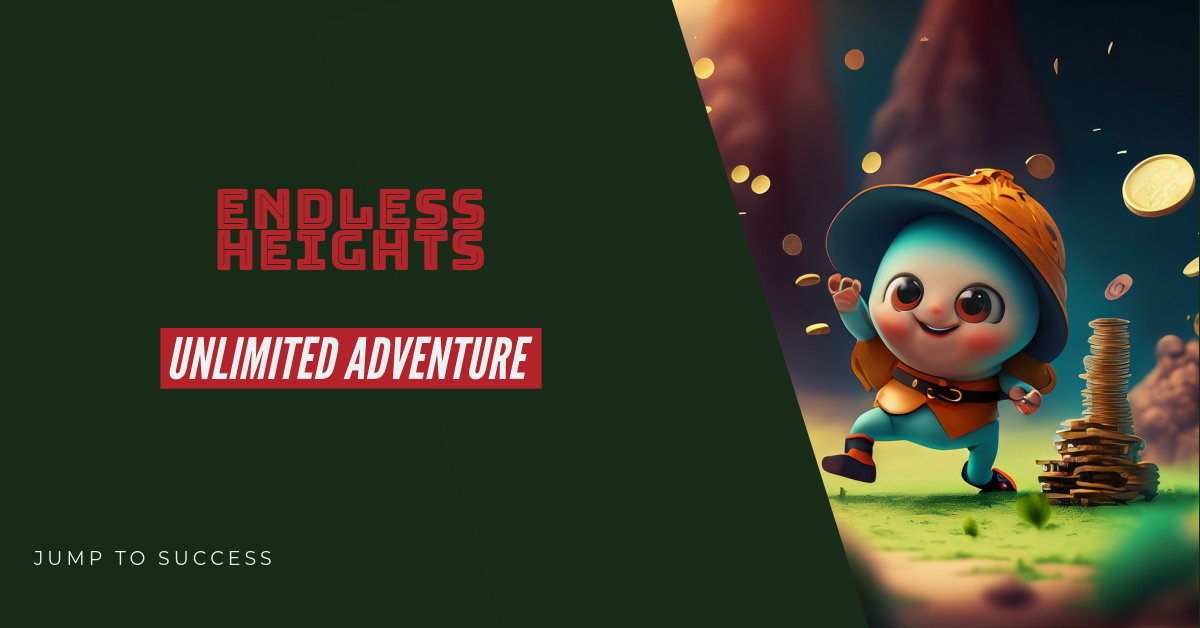
By creating a Doodle Jump clone, you will create a game where players control characters that jump continuously from platform to platform. This character aims to reach as high as possible without falling. Simplified HTML, CSS, and JavaScript mechanics are covered in this version.
Step 1: HTML Structure
Define the game area and the player’s character.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Doodle Jump Clone</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameArea">
<div id="player"></div>
</div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Make sure the player and the game area are styled. JavaScript will generate dynamic platforms.
#gameArea {
width: 400px;
height: 600px;
background-color: lightblue;
position: relative;
overflow: hidden;
margin: auto;
}
#player {
width: 30px;
height: 30px;
background-color: red;
position: absolute;
bottom: 50px;
left: 50%;
transform: translateX(-50%);
}
Step 3: JavaScript Logic
Make sure the game logic is implemented, such as platform generation, gravity, and jumping.
document.addEventListener('DOMContentLoaded', () => {
const gameArea = document.getElementById('gameArea');
const player = document.getElementById('player');
let playerLeft = 50; // Player's horizontal position
let playerBottom = 50; // Player's vertical position
let gravity = 3;
let isJumping = false;
let isGameOver = false;
let platformCount = 5;
let platforms = [];
function createPlatforms() {
for (let i = 0; i < platformCount; i++) {
let platformGap = 600 / platformCount;
let newPlatformBottom = 100 + i * platformGap;
let platform = document.createElement('div');
platform.classList.add('platform');
platform.style.left = Math.random() * 315 + 'px';
platform.style.bottom = newPlatformBottom + 'px';
gameArea.appendChild(platform);
platforms.push(platform);
}
}
function movePlatforms() {
if (playerBottom > 200) {
platforms.forEach(platform => {
let newBottom = parseInt(platform.style.bottom) - 4;
platform.style.bottom = newBottom + 'px';
if (newBottom < 10) {
let firstPlatform = platforms[0];
firstPlatform.remove();
platforms.shift();
createPlatform();
}
});
}
}
function createPlatform() {
let newPlatform = document.createElement('div');
newPlatform.classList.add('platform');
newPlatform.style.left = Math.random() * 315 + 'px';
newPlatform.style.bottom = 500 + 'px';
gameArea.appendChild(newPlatform);
platforms.push(newPlatform);
}
function jump() {
isJumping = true;
let upTimerId = setInterval(() => {
playerBottom += 20;
player.style.bottom = playerBottom + 'px';
if (playerBottom > 250) { // Peak of jump
clearInterval(upTimerId);
let downTimerId = setInterval(() => {
playerBottom -= 5;
player.style.bottom = playerBottom + 'px';
if (playerBottom <= 50) {
clearInterval(downTimerId);
isJumping = false;
}
}, 20);
}
}, 20);
}
function startGame() {
createPlatforms();
jump();
// Add more game mechanics as needed
}
startGame();
});
Game mechanics explained
In the game, platforms are generated randomly across the game field. Player character jumps between platforms.
Gravity and Jumping: The player character automatically jumps, and gravity pulls it back down. The game simulates a jump with intervals, increasing the player’s bottom position for the upward phase and decreasing it for the downward phase.
Moving Platforms: As the player reaches higher, the platforms shift down to simulate the camera following the player upward. Anoinous platforms are generated at the top as old ones move out of view at the bottom.
Enhancements
For a complete game, consider adding:
Scoring: Increase the score as the player reaches higher levels.
Player Controls: Allow the player to move the character left and right with keyboard or touch controls.
Game Over Condition: End the game if the player misses a platform and falls.
Audio/Visual Effects: Enhance the game with visual effects for jumping and landing, plus sound effects and background music.
Summary
A Doodle Jump-style game can be created using this setup, which involves vertical movement, platform generation, and simple jumping mechanics. Expanding and polishing this foundation can result in an engaging and challenging game.
Create an easy-to-understand game similar to Doodle Jump using these tips. This game involves helping a character jump from one platform to another without falling.
Setting Up the Game
Creating the Playground: Using HTML, we draw a box on the screen representing where our game happens. Inside this box, there’s a small square or character (the player) that we will move around.
Decorating CSS makes our game look nice. We color the game area (the box) and style our characters to stand out. We ensure the character appears at the bottom of the game area, ready to jump.
Making It Playable: JavaScript brings our game to life. We create invisible steps or platforms inside the game area. Our character will jump from one platform to another, moving upwards each time.
How to play?
Jumping: The character automatically jumps up from the bottom. Your goal is to move it so it lands on the next platform each time it comes down.
Moving higher: Each time the character lands on a platform, it jumps again. The platforms move downwards, so the character goes faster and higher.
Staying Alive: The game is over if the character misses a platform and falls. The challenge is to land on as many platforms as possible.
Making the game work
Platforms: We sprinkle several platforms randomly across the game area. The character needs to land on these to bounce back up.
Gravity and Jumping: We make the character constantly fall (like gravity pulls it). When it touches a platform, it gets a boost to jump back up.
Winning and Losing: You win by getting the highest score—how high you can get before missing a platform. In the game, you will lose if you miss a platform and fall.
What We’ve Learned
HTML draws the game area and characters.
CSS makes everything look pristine and defines character starting position.
JavaScript adds game rules and actions, like jumping and scoring.
Fun additions
As you get more comfortable with coding, you can add a few features like:
The character can move left and right to land on platforms as the player controls it.
Scoring: Keep track of how high the character gets scores points.
Challenges: Add moving or disappearing platforms for a more challenging game.
Summary for students
Building a game like Doodle Jump teaches you to use HTML, CSS, and JavaScript to create something fun and interactive. You learn to draw with HTML, decorate with CSS, and make things move and react with JavaScript. Assembling a puzzle is akin to building a puzzle, since every part is crucial to the game’s outcome.
For reference links and further reading, while I can’t provide live internet content, consider these types of resources:
- Unity Tutorials : Unity’s official learning resources are fantastic for beginners and advanced developers alike, covering everything from basic game mechanics to advanced platforming game development.
- Gamasutra : This site offers insights, postmortems, and articles by game developers and industry experts, which can be invaluable when looking into the nuances of game design and development.
- Game Development Stack Exchange : A Q&A site for professional and independent game developers. It’s a great place to ask specific questions about game development or search for issues others have faced and solved.
Leave a Reply