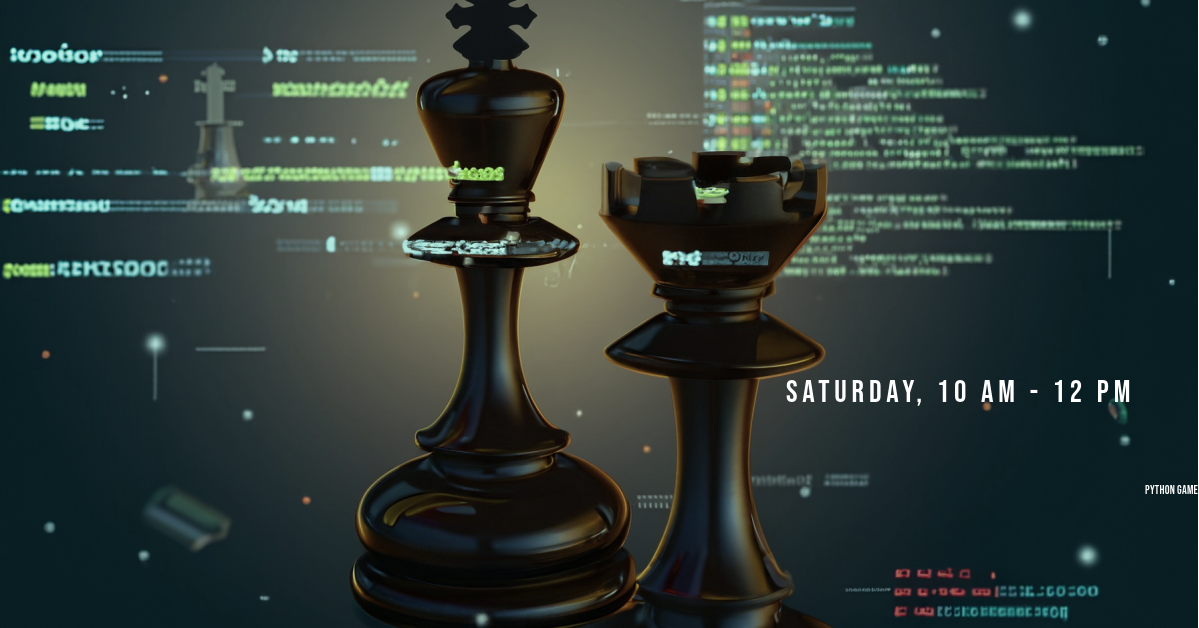
Checkers Game with Basic AI Plan:
- Language: Python, suitable for creating board games and implementing basic AI.
- Game Elements:
- A 8×8 game board with black and red pieces.
- Basic movement and capturing rules of Checkers.
- Features:
- Two-player mode: Two players take turns to play.
- Single-player mode with basic AI: Player vs. a simple AI that makes random valid moves.
- Display of the game board in the console.
- Basic rules implementation for moving and capturing pieces.
- AI Implementation:
- The AI should randomly choose a valid move from available moves.
- User Interface:
- Text-based interaction: Players input their moves via the console.
Checkers Game with Basic AI Code:
import random
def initialize_board():
board = []
for row in range(8):
board_row = []
for col in range(8):
if row % 2 != col % 2:
if row < 3:
board_row.append('R') # Red pieces
elif row > 4:
board_row.append('B') # Black pieces
else:
board_row.append('.')
else:
board_row.append(' ')
board.append(board_row)
return board
def print_board(board):
for row in board:
print(' '.join(row))
print()
def get_valid_moves(board, player):
moves = []
# Add logic to calculate valid moves for the player
return moves
def ai_move(board):
valid_moves = get_valid_moves(board, 'B')
return random.choice(valid_moves) if valid_moves else None
def player_move(board, player):
valid_moves = get_valid_moves(board, player)
# Add logic for player to make a move
return chosen_move
def is_game_over(board):
# Add logic to determine if the game is over
return False
# Initialize the game
board = initialize_board()
current_player = 'R' # Red starts
# Main game loop
while not is_game_over(board):
print_board(board)
if current_player == 'R':
player_move(board, 'R')
else:
ai_move(board)
current_player = 'B' if current_player == 'R' else 'R'
print("Game over!")
How to Run:
- Save this script in a
.py
file. - Run the script using Python, and the Checkers game will start.
- The game is played in the console. Players input their moves, and the AI makes random valid moves.
This code sets up a basic framework for a Checkers game. It initializes the board, prints it, and alternates turns between a human player and an AI. The AI randomly selects from valid moves. The code for calculating valid moves, player input, and game over conditions needs to be filled in for a complete game. This script provides a structure that can be expanded upon to create a more advanced Checkers game.
Initializing the Game Board
initialize_board
Function:def initialize_board():
# Code to create an 8x8 board with initial checkers placement
- This function creates an 8×8 game board.
- “R” represents red checkers, “B” for black checkers, “.” for empty playable squares, and ” ” for non-playable squares.
print_board
Function:def print_board(board):
# Code to print the game board
- Prints the current state of the game board to the console.
Handling Moves
get_valid_moves
Function:def get_valid_moves(board, player):
# Code to calculate valid moves for the given player
- This function would calculate all valid moves for the current player (“R” or “B”). It’s where the rules of checkers would be implemented, but this logic is not detailed in the provided code.
AI Move Function:
def ai_move(board):
# Code for AI to make a random valid move
- The AI selects a random move from the list of valid moves. This function represents a very basic AI behavior.
Player Move Function:
def player_move(board, player):
# Code for player to input and make a move
- This function would allow the human player to input their move. The actual implementation would involve checking if the move is valid and then updating the board.
Checking Game Status
is_game_over
Function:def is_game_over(board):
# Code to check if the game is over
- Determines whether the game has ended, either if a player has no more moves or checkers left.
Running the Game
- The game initializes the board and sets the current player.
- In the main game loop, the board is printed, and the current player makes a move. The player alternates each turn.
- The game continues until
is_game_over
returnsTrue
.
Learning Points for Students
- Grid Representation: Understanding how a 2D list can represent a game board.
- Loops and Conditions: Using loops to create the board and conditions to place the initial checkers.
- Function Implementation: The importance of breaking down the game logic into specific functions.
- AI Basics: Introduction to AI in games, even as simple as making random moves.
- User Input and Game Logic: The code structure suggests how players might input their moves and how the game would process them.
This code provides a basic framework for creating a text-based Checkers game. It demonstrates fundamental programming concepts like working with lists, implementing game logic, and handling user input. The AI part is simplified to random move selection, which serves as an introduction to how computer opponents can be integrated into games.
Reference Links to Include:
Python Game Development Libraries:
- For tutorials on using libraries like Pygame for game development.
- Suggested Search: “Python Pygame tutorials”
Artificial Intelligence in Games:
- To provide insights into integrating AI into Python games, enhancing the challenge and engagement of chess and checkers.
- Suggested Search: “AI in Python games”
GitHub Repositories for Chess and Checkers in Python:
- For practical examples and inspiration from existing Python projects.
Stack Overflow for Python Game Development:
- A resource for troubleshooting and advice related to game development challenges in Python.
Leave a Reply