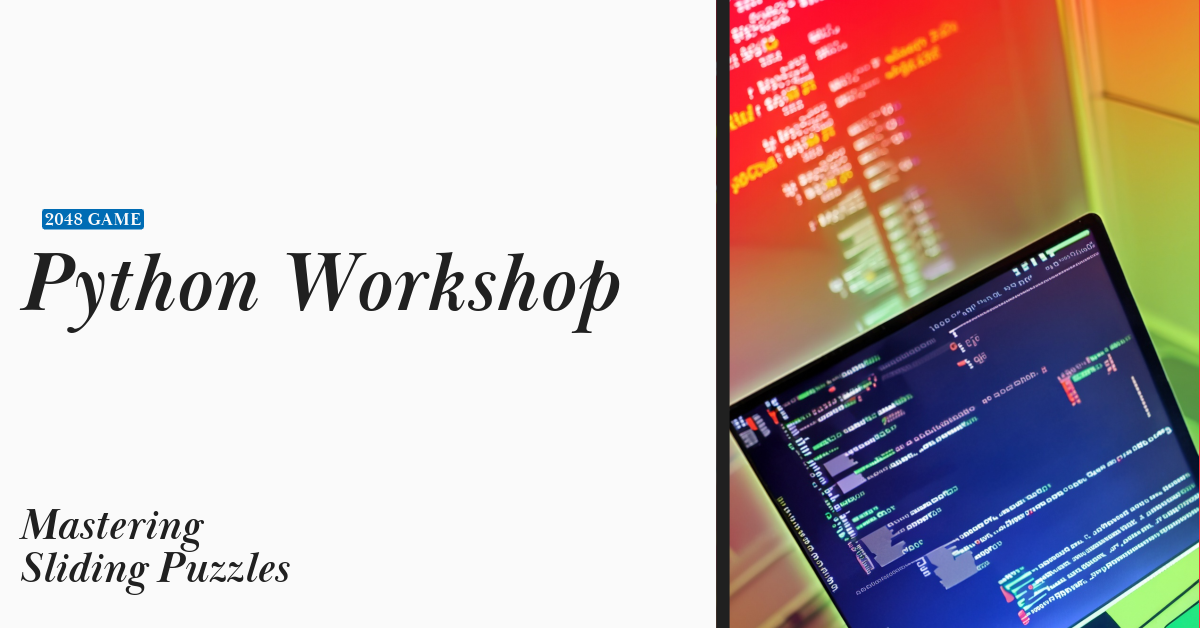
2048 Game Algorithm Plan:
Language: Python is ideal for creating grid-based logic and handling user input.
Game elements:
A 4×4 grid where each cell holds a number.
Numbers on tiles that can be combined when they are the same.
Features:
Ability to slide all tiles in four directions (up, down, left, right).
Combining equal numbers when they collide.
Randomly adding another number (typically 2 or 4) to the grid after each move.
Checking for game over conditions.
User interaction:
Text-based input for moving tiles.
Displaying the grid after each move.
2048 Game Code:
import random
def initialize_grid():
return [[0 for _ in range(4)] for _ in range(4)]
def add_new_tile(grid):
row = random.randint(0, 3)
col = random.randint(0, 3)
while grid[row][col] != 0:
row = random.randint(0, 3)
col = random.randint(0, 3)
grid[row][col] = random.choice([2, 4])
def compress_grid(grid):
new_grid = [[0 for _ in range(4)] for _ in range(4)]
for row in range(4):
position = 0
for col in range(4):
if grid[row][col] != 0:
new_grid[row][position] = grid[row][col]
position += 1
return new_grid
def merge_grid(grid):
for row in range(4):
for col in range(3):
if grid[row][col] == grid[row][col + 1]:
grid[row][col] *= 2
grid[row][col + 1] = 0
return grid
def reverse_grid(grid):
new_grid = []
for row in grid:
new_grid.append(row[::-1])
return new_grid
def transpose_grid(grid):
new_grid = [[grid[j][i] for j in range(4)] for i in range(4)]
return new_grid
def move_left(grid):
grid = compress_grid(grid)
grid = merge_grid(grid)
grid = compress_grid(grid)
return grid
def move_right(grid):
grid = reverse_grid(grid)
grid = move_left(grid)
grid = reverse_grid(grid)
return grid
def move_up(grid):
grid = transpose_grid(grid)
grid = move_left(grid)
grid = transpose_grid(grid)
return grid
def move_down(grid):
grid = transpose_grid(grid)
grid = move_right(grid)
grid = transpose_grid(grid)
return grid
# Initialize the game
grid = initialize_grid()
add_new_tile(grid)
add_new_tile(grid)
# Main game loop
running = True
while running:
for row in grid:
print(row)
move = input("Enter move (W, A, S, D): ")
if move.lower() == 'w':
grid = move_up(grid)
elif move.lower() == 'a':
grid = move_left(grid)
elif move.lower() == 's':
grid = move_down(grid)
elif move.lower() == 'd':
grid = move_right(grid)
else:
print("Invalid move. Try again.")
add_new_tile(grid)
# Check for game over condition
# ...
pygame.quit()
How to run:
Save this script as a .py file.
Run the script using Python. The game will start on the console.
Use the W, A, S, and D keys to navigate Left, Right, and Up.
This code creates a basic 2048 game where the player can move tiles in four directions. Each move compresses the grid, merges adjacent identical numbers, and adds another tile. The game is played on the console and continues until the player closes the window. The code for checking the game over condition is separate from this basic version.
Game Setup and Grid Initialization
Initialize the grid:
Def initialize_grid():
Return [0 for in range(4)] for in range(4)]
Create a 4×4 grid, filling it with zeros. Each zero represents a space in the game.
Add a New Tile:
Def add_new_tile(grid):
# Code to add tiles (2 or 4) to a random empty spot on the grid
Randomly selects an empty spot on the grid and places another tile, 2 or 4.
Grid manipulation functions
These functions manipulate the grid based on player input (W, A, S, D for up, left, down, right).
Compress the grid:
Def compress_grid(grid):
# Code to shift all numbers to the left
Shifts all non-zero numbers to the left side of the grid.
Merge numbers:
Def merge_grid(grid):
# Code to combine adjacent identical numbers
Combines adjacent identical numbers and doubles their value.
Reverse and Transpose:
Def reverse_grid(grid):
Def transpose_grid(grid):
Reverse_grid reverses each row of the grid. This is beneficial for right-to-left and down-to-right movements.
Transpose_grid swaps rows and columns, valid for up and down moves.
Move Functions:
Move_left, move_right, move_up, move_down:
These functions use the above manipulation functions to move in the specified direction.
Main Game Loop
While running:
# Display the grid and get the players’ input for the next move
It runs continuously, showing the grid’s current state and asking the player for the next move.
After each move, it compresses and merges the grid, then adds the next tile.
Handling player input
The move as input(“Enter the move (W, A, S, D): “)
It takes the player’s input to determine how to move the tiles.
Learning Points for Students
Multidimensional Lists: Understanding how to use and manipulate 2D lists to represent game grids.
Nested Loops and List Comprehensions: Using nested loops and list comprehensions for grid initialization and manipulation.
Functions and Modular Code: This section explains how to break down game logic into specific tasks for better code organization and readability.
Conditional Statements: Using if-else statements to control game logic based on user input.
Basic Game Mechanics: Learn how to code a puzzle game that moves and merges tiles.
Reference Links to Include:
Python Official Documentation:
- For comprehensive Python syntax and library references.
Pygame Library Documentation (if using Pygame):
- Essential for game development specifics, especially if your tutorial involves graphical elements.
GitHub Repositories for 2048 in Python:
- To provide examples of other 2048 games developed in Python, offering coding inspiration and insight.
Stack Overflow Python Game Development:
- A valuable resource for troubleshooting and community advice on game development with Python.
Leave a Reply