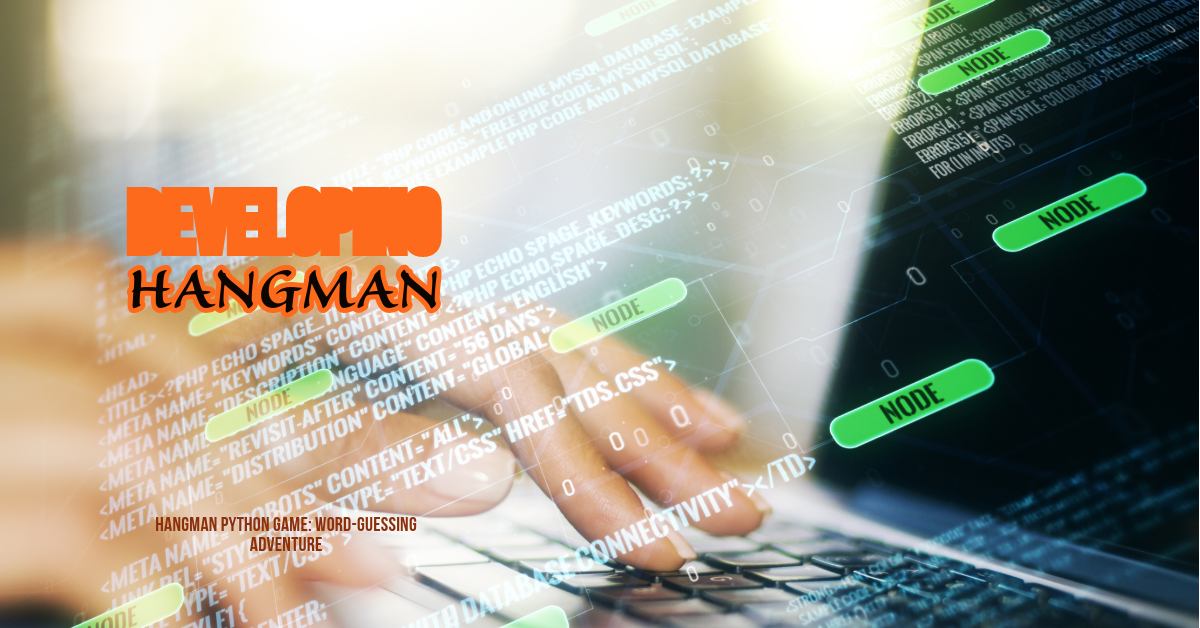
Hangman Game Plan:
Language: Python, suitable for text processing and simple graphics.
Library: Pygame, which creates graphical displays of letters and hangman puzzles.
Game elements:
A word or phrase that players will guess.
A display of blank spaces representing each letter in the word.
A graphical representation of the hangman is drawn for each incorrect guess.
Features:
Players guess letters one at a time.
Correct guesses reveal the word’s letters.
Incorrect guesses result in hangman parts being drawn.
The game ends when the word is guessed, or the hangman is fully drawn.
User interface:
A window showing the hangman, the word (as blank spaces and revealed letters), and guessing letters.
Hangman Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
# Word list
WORDS = ["python", "hangman", "programming", "game"]
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Hangman Game')
# Choose a word
word = random.choice(WORDS)
guessed = ["_" for _ in word]
wrong_guesses = 0
# Main game loop
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
guess = pygame.key.name(event.key)
if guess in word:
for i, letter in enumerate(word):
if letter == guess:
guessed[i] = letter
else:
wrong_guesses += 1
# Draw the screen
screen.fill(WHITE)
font = pygame.font.SysFont(None, 36)
display_word = " ".join(guessed)
text = font.render(display_word, True, BLACK)
screen.blit(text, (50, 50))
# Check for game over
if "_" not in guessed or wrong_guesses >= 6:
running = False
pygame.display.flip()
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Hangman game will start.
This code creates a basic Hangman game where the player guesses letters to reveal a hidden word. The game displays the word as a series of underscores (representing unguessed letters) and updates them as the player answers correctly. The game ends if the player makes too many wrong guesses or successfully guesses the word. This version does not include a graphical hangman, but the framework is there for adding this feature.
Importing libraries
Import a pygame
Import random
Pygame is used to create the game window and handle events.
Randomly selects a random word from a list.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
Initializes Pygame, which is necessary for game development.
Constants for screen size and color.
Setting Up the Game Window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Hangman Game’)
Sets up the game window with the specified width and height.
Set the window title to ‘Hangman Game.’
Choosing a Word and Setting Up Variables
Word = random.choice(WORDS)
Guess = [“_” for _ in words]
Wrong_guesses = 0
Choose a random word from a predefined list of WORDS.
Guess keeps track of the letters guessed by the player, initially filled with underscores.
Wrong_guesses counts the number of incorrect guesses.
Main Game Loop
While running:
# Event handling and game logic
The game’s main loop runs continuously to handle events and update the game state.
Handling events (Keyboard input)
For the game events. Event.get():
# Check for quit and key presses
Listens for the quit event to close the game and keyboard events for player guesses.
Update the Game State
# Code to update guessing letters and count wrong guesses
If the guess letter is in the word, it updates the guess to reveal it.
If the guessed letter is not in the word, it increments wrong_guesses.
Drawing the screen
# Drawing code for the guesswork and the hangman (if implemented)
Clears the screen to show the current state of the guessed word.
(Note: The graphical hangman is not implemented in this basic version, but this is where it would be drawn.)
Checking for Game Over
If “_” is not in guess or wrong_guess >= 6:
Running = false.
Check if the game is over because the player guesses the whole word or making too many wrong guesses.
We are updating the display.
Pygame. Display.flip()
Updates the entire screen with what has been drawn.
Playing the game is leaving.
Pygame.quit()
It closes the game window.
Learning Points for Students
Game Loop: Understanding the main loop that handles events and updates the game state.
Event Handling: Responding to user inputs like keyboard presses.
Lists and Strings: Using lists to compare letters and strings.
Conditional Logic: Implementing game rules with if-else statements.
Essential Graphics: How to use pygame to draw text on the screen.
Reference Links to Include:
Python Official Documentation:
- Purpose: For foundational Python knowledge and reference.
Tkinter Documentation for GUI (if using Tkinter):
- Purpose: Essential for readers interested in adding a graphical interface to their Hangman game.
GitHub Repositories for Hangman in Python:
- Purpose: To provide examples of Hangman games developed in Python, offering coding inspiration and insight.
Pygame Documentation (if using Pygame for graphics):
- Purpose: For readers who want to explore creating more advanced graphics for their Hangman game.
Leave a Reply