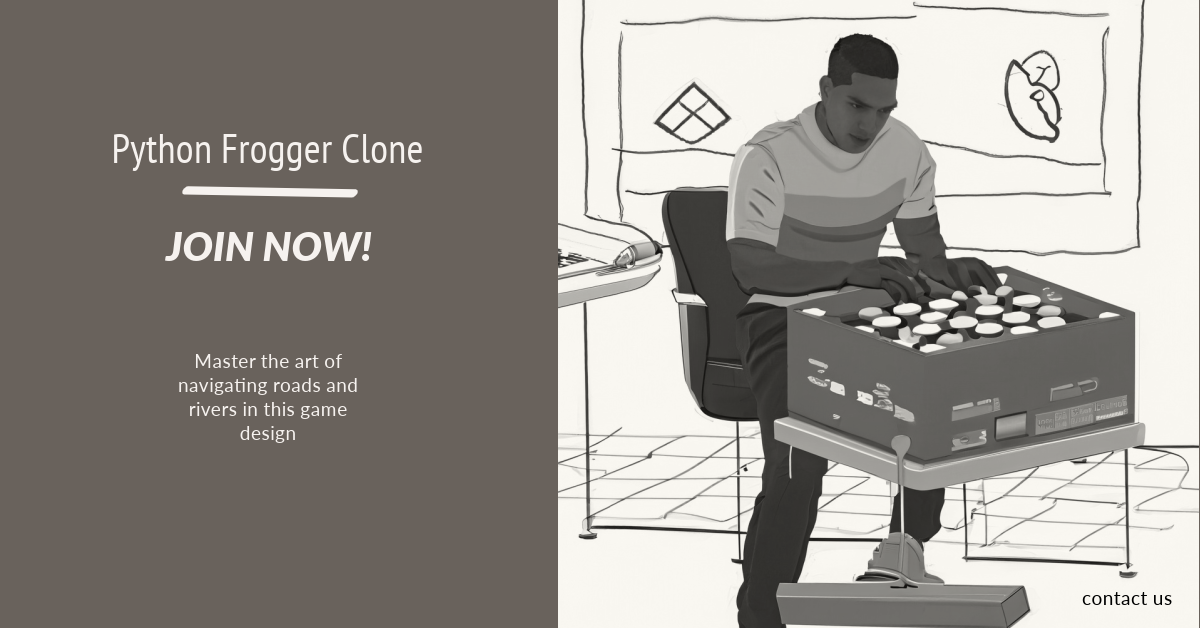
Frogger Clone Game Plan:
- Language: Python, suitable for simple game development and graphical representation.
- Library:
pygame
, for handling graphics and user inputs. - Game Elements:
- A frog character controlled by the player.
- A busy road with moving vehicles.
- A river with moving logs or lily pads.
- Safe spots or homes for the frog to reach.
- Features:
- Player can move the frog in four directions: up, down, left, and right.
- Collision detection with vehicles and river hazards.
- Safely navigating the frog to a home spot.
- Resetting the frog’s position after reaching a home or losing a life.
- User Interface:
- A window displaying the game with the frog, road, river, and homes.
- Optional: Display for lives or score.
Frogger Clone Game Code:
This code snippet is a conceptual framework. It outlines key parts of the game without implementing full collision detection, multiple lanes of traffic, or river hazards.
import pygame
import random
# Initialize Pygame
pygame.init()
# Screen dimensions
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Colors
GREEN = (0, 255, 0)
BLACK = (0, 0, 0)
# Setup the display
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Frogger Clone')
# Frog settings
frog_size = 50
frog_pos = [SCREEN_WIDTH // 2, SCREEN_HEIGHT - frog_size]
frog_speed = 20
# Game loop flag
running = True
# Clock to control game refresh rate
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
frog_pos[0] -= frog_speed
elif event.key == pygame.K_RIGHT:
frog_pos[0] += frog_speed
elif event.key == pygame.K_UP:
frog_pos[1] -= frog_speed
elif event.key == pygame.K_DOWN:
frog_pos[1] += frog_speed
# Game logic (e.g., move the frog, check for collisions)
# Drawing
screen.fill(BLACK) # Clear screen
pygame.draw.rect(screen, GREEN, (*frog_pos, frog_size, frog_size)) # Draw frog
# Update the display
pygame.display.flip()
# Cap the frame rate
clock.tick(30)
pygame.quit()
How to Run:
- Ensure you have Python and Pygame installed on your system.
- Save this script to a file, for example,
frogger_clone.py
. - Run the script from your terminal or command prompt:
python frogger_clone.py
. - Use the arrow keys to move the frog up, down, left, and right.
Game Explanation for Students:
- Initializing Pygame: Sets up the library for creating the game window and handling events.
- Screen Dimensions and Colors: Defines the size of the game window and colors used in the game.
- Display Setup: Creates the game window where the game will be drawn.
- Frog Settings: Defines the size, initial position, and speed of the frog.
- Game Loop: Continues running the game, handling events (like keyboard inputs for moving the frog), updating game logic, and redrawing the screen.
- Event Handling: Checks for the quit event to stop the game and arrow key presses to move the frog.
- Drawing: Clears the screen and draws the frog in its current position.
- Frame Rate Control: Uses a clock to ensure the game runs at a consistent speed.
Setting Up the Game
Initializing Pygame:
pygame.init()
- This line starts Pygame, which is necessary for making the game work. Pygame is a library that provides functions for creating games in Python.
Screen Dimensions and Colors:
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
GREEN = (0, 255, 0)
BLACK = (0, 0, 0)
- These lines set the width and height of the game window and define colors that will be used to draw objects.
GREEN
is for the frog, andBLACK
is for the background.
- These lines set the width and height of the game window and define colors that will be used to draw objects.
Creating the Game Window:
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Frogger Clone')
- This creates the game window with the specified dimensions and sets the title of the window.
The Frog
- Frog Settings:
frog_size = 50
frog_pos = [SCREEN_WIDTH // 2, SCREEN_HEIGHT - frog_size]
frog_speed = 20
- These lines define the frog’s size, initial position (starting in the middle at the bottom of the screen), and how fast it moves.
The Game Loop
- Running the Game:
while running:
# Handling events, updating game state, and drawing
- The
while
loop keeps the game running. Inside this loop, the game checks for player input (like pressing keys), updates the game’s state (like moving the frog), and draws the game objects on the screen.
- The
Handling Player Input
- Moving the Frog:
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_LEFT:
frog_pos[0] -= frog_speed
- This part checks if the player has pressed a key. If the left arrow key is pressed, it moves the frog to the left by decreasing its
x
position.
- This part checks if the player has pressed a key. If the left arrow key is pressed, it moves the frog to the left by decreasing its
Drawing on the Screen
- Drawing the Frog:
pygame.draw.rect(screen, GREEN, (*frog_pos, frog_size, frog_size))
- Clears the screen and then draws the frog as a green rectangle at its current position.
Updating the Display
- Updating the Game Window:
pygame.display.flip()
- This line updates the entire game window, showing the latest drawn frame. It’s necessary to see the changes made (like moving the frog).
Controlling the Game Speed
- Frame Rate Control:
clock.tick(30)
- This ensures that the game runs at 30 frames per second, making the game’s speed consistent across different computers.
Ending the Game
- Exiting Pygame:
pygame.quit()
- Stops Pygame and closes the game window when the game loop ends (e.g., if the player closes the game window).
Learning Points for Students
- Game Development Basics: Understanding how to set up a game window, process user input, and draw graphics.
- Event Loop: Learning about the game loop that continuously checks for events, updates the game state, and redraws the screen.
- Conditional Statements: Using
if
statements to react to specific keys being pressed to move the game character. - Coordinates and Movement: Manipulating the position of game objects to create movement.
Reference Links to Include:
Python Game Development with Pygame:
- For an introduction to game development in Python, particularly using Pygame for creating arcade-style games like Frogger.
- Suggested Search: “Python Pygame tutorial”
Game Design Principles:
- To offer insights into fundamental game design principles that can be applied to developing a Frogger clone.
- Suggested Search: “Game design fundamentals”
GitHub Repositories for Frogger in Python:
- For examples of Frogger clones developed in Python, providing coding inspiration and practical insights.
Stack Overflow for Python Game Development Queries:
- A platform for seeking advice and solutions to common challenges encountered in Python game development.
Leave a Reply