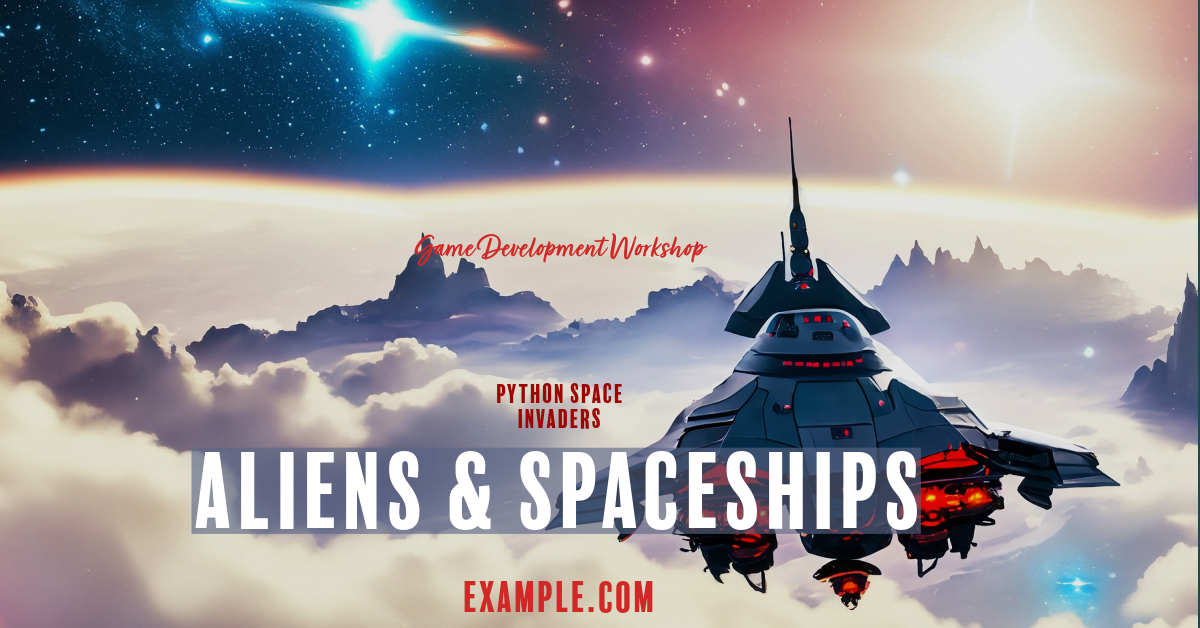
Space Invaders Clone Game Plan:
Language: Python is an excellent choice for demonstrating game development concepts.
Library: Pygame for rendering graphics, handling events, and managing game states.
Game elements:
The player at the bottom of the screen controls a spaceship.
Aliens move horizontally and ascend.
The player’s ability to shoot bullets to destroy aliens.
Features:
Horizontal movement of the spaceship using keyboard inputs (left and right arrows).
Spaceships fire bullets to destroy the aliens.
Aliens move in a pattern and descend toward the spaceship.
Game over conditions (e.g., aliens reaching the spacecraft or the player losing all lives).
User interface:
A window showing spacecraft, aliens, and bullets.
Score and live display.
Space Invaders Clone Game Code
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
SPACESHIP_WIDTH, SPACESHIP_HEIGHT = 50, 50
ALIEN_WIDTH, ALIEN_HEIGHT = 40, 40
BULLET_WIDTH, BULLET_HEIGHT = 5, 10
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Space Invaders Clone')
# Spaceship setup
spaceship = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT - 60, SPACESHIP_WIDTH, SPACESHIP_HEIGHT)
# Aliens setup
aliens = [pygame.Rect(x * 60, y * 60, ALIEN_WIDTH, ALIEN_HEIGHT) for x in range(10) for y in range(3)]
# Bullets setup
bullets = []
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bullets.append(pygame.Rect(spaceship.centerx, spaceship.top, BULLET_WIDTH, BULLET_HEIGHT))
# Spaceship movement
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and spaceship.left > 0:
spaceship.move_ip(-5, 0)
if keys[pygame.K_RIGHT] and spaceship.right < SCREEN_WIDTH:
spaceship.move_ip(5, 0)
# Bullet movement
for bullet in bullets[:]:
bullet.move_ip(0, -10)
if bullet.bottom < 0:
bullets.remove(bullet)
# Alien movement
for alien in aliens[:]:
alien.move_ip(0, 1)
if alien.bottom > SCREEN_HEIGHT:
running = False
# Bullet collision with aliens
for bullet in bullets[:]:
for alien in aliens[:]:
if bullet.colliderect(alien):
bullets.remove(bullet)
aliens.remove(alien)
break
# Drawing everything
screen.fill(BLACK)
for alien in aliens:
pygame.draw.rect(screen, GREEN, alien)
pygame.draw.rect(screen, WHITE, spaceship)
for bullet in bullets:
pygame.draw.rect(screen, RED, bullet)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Space Invaders Clone game will start.
In this code, the player controls a spaceship at the bottom of the screen to play Space Invaders Clone. As the spacecraft moves horizontally, bullets can be fired to destroy aliens descending. The game ends if an alien reaches the bottom of the screen.
Importing libraries
Import a pygame
Import random
Random is used for generating random values, though it’s not actively used in this basic version.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Other constants for spaceship, alien, bullet sizes, and colors
Pygame. Init () starts all the Pygame modules.
Constants are set for screen size, spaceship, alien, bullet sizes, and colors.
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Space Invaders Clone’)
This sets up the game window with the specified width and height.
The caption sets the window title.
Setting Up Game Elements
Spaceship = pygame.Rect(…)
Alien = [… for x in range(10) and for y in range(3)]
Bullets = []
The spaceship, aliens, and bullets are initialized.
Spaceship is a rectangle representing the player’s spaceship.
Alien is a list of rectangles representing enemies.
Bullets is a list to keep track of bullets fired by the player.
The Game Loop
While running:
# Event handling, spaceship movement, bullet movement…
The main game loop handles events, updates game state, and renders graphics.
Event Handling and Spaceship Movement
For an event in Pygame. Event.get():
# Event handling for stopping and shooting
Key = pygame. Key.get_pressed()
# Spaceship movement logic
Processes player inputs for quitting the game, moving the spaceship, and firing bullets.
Bullet and Alien Movement
For bullets in bullets[:]:
# Bullet movement logic
For aliens in aliens[:]:
# Alien movement logic
Updates bullet positions and moves them up the screen.
Move each alien down the screen.
Collision detection
For bullets in bullets[:]:
For aliens in aliens[:]:
# Check for collision between bullet and alien
Upon collision with an alien, remove the bullet and the alien.
Drawing on the screen
Screen.fill(BLACK)
# Drawing code for aliens, spaceships, and bullets
He clears the screen and draws aliens, spaceships, and bullets.
Update the display and frame rate
pygame. Display.flip()
Clock.tick(60)
Update the screen with drawn graphics.
It runs at 60 frames per second.
It is exciting to play the game.
Pygame.quit()
Closes the playgame window and exits the game.
Learning Points for Students
Loops and Conditionals: The game uses loops for the primary game cycle and conditionals for handling collisions and movements.
List Management: Managing lists of aliens and bullets, adding and removing elements based on game logic.
Collision Detection: This is the crucial part of the game where collisions between bullets and aliens are detected.
Basic Game Mechanics: Understanding how to control game entities, detect collisions, and render graphics.
Reference Links to Include:
Python Official Documentation:
- Purpose: For essential Python syntax and library information.
Pygame Documentation (for game development):
- Purpose: Crucial for creating the game’s graphical interface and handling user interactions.
GitHub Repositories for Space Invaders in Python:
- Purpose: To offer coding inspiration and practical examples from existing Space Invaders clones developed in Python.
Stack Overflow Python Game Development:
- Purpose: A valuable resource for troubleshooting common programming challenges and getting advice on game development with Python.
Leave a Reply