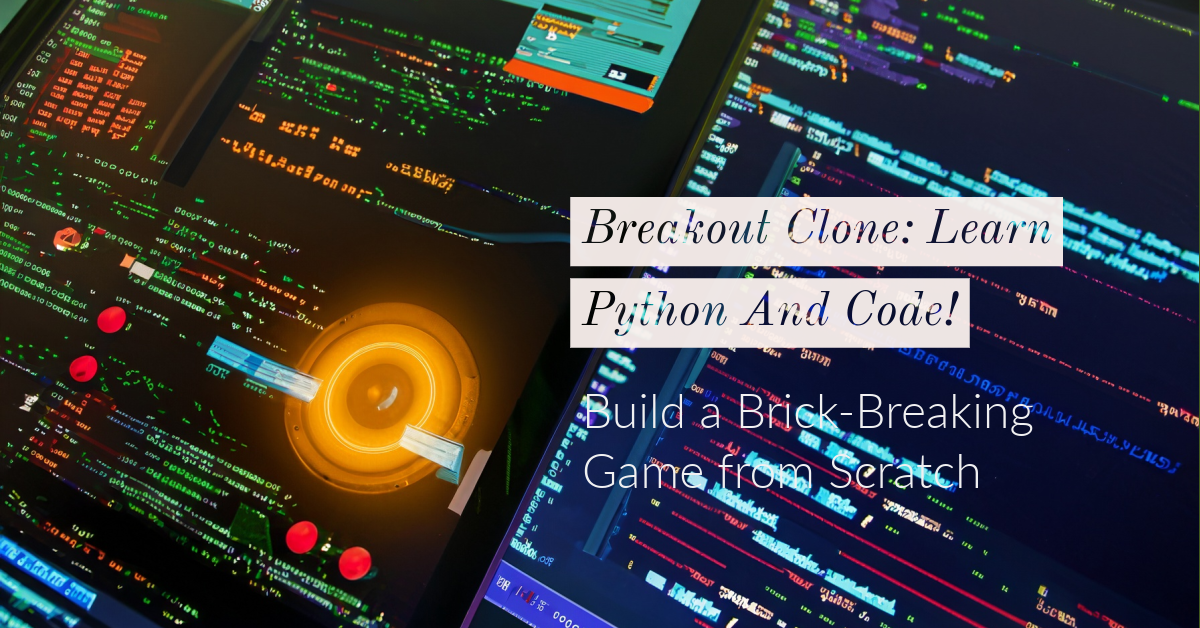
Breakout Clone Game Plan:
Language: Python, excellent for game development basics.
Library: pygame, for creating the game window, handling events, and rendering graphics.
Game elements:
In this game, the player uses a paddle at the bottom of the screen to control movement.
A ball that bounces off the paddle and onto the wall.
Bricks are arranged at the top of the screen so the ball can break.
Features:
Movement of the paddle using keyboard inputs (left and right arrows).
Ball movement that bounces off walls, paddles, and bricks.
Removing bricks when hit by a ball.
Game over conditions (e.g., when the ball misses the paddle).
User interface:
An image of the game with a paddle, ball, and bricks displayed in a window.
The score displayed is based on the number of bricks broken.
Breakout Clone Game Code
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
PADDLE_WIDTH, PADDLE_HEIGHT = 100, 20
BALL_RADIUS = 8
BRICK_WIDTH, BRICK_HEIGHT = 60, 30
WHITE = (255, 255, 255)
RED = (255, 0, 0)
BLUE = (0, 0, 255)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Breakout Clone')
# Paddle setup
paddle = pygame.Rect(SCREEN_WIDTH // 2 - PADDLE_WIDTH // 2, SCREEN_HEIGHT - 40, PADDLE_WIDTH, PADDLE_HEIGHT)
# Ball setup
ball = pygame.Rect(SCREEN_WIDTH // 2, SCREEN_HEIGHT // 2, BALL_RADIUS, BALL_RADIUS)
ball_speed_x = 6 * random.choice((1, -1))
ball_speed_y = 6 * random.choice((1, -1))
# Bricks setup
bricks = [pygame.Rect(x * (BRICK_WIDTH + 10) + 10, y * (BRICK_HEIGHT + 5) + 5, BRICK_WIDTH, BRICK_HEIGHT) for x in range(10) for y in range(5)]
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
# Paddle movement
keys = pygame.key.get_pressed()
if keys[pygame.K_LEFT] and paddle.left > 0:
paddle.move_ip(-10, 0)
if keys[pygame.K_RIGHT] and paddle.right < SCREEN_WIDTH:
paddle.move_ip(10, 0)
# Ball movement
ball.move_ip(ball_speed_x, ball_speed_y)
# Ball collision with walls
if ball.left <= 0 or ball.right >= SCREEN_WIDTH:
ball_speed_x *= -1
if ball.top <= 0:
ball_speed_y *= -1
# Ball collision with paddle
if ball.colliderect(paddle):
ball_speed_y *= -1
# Ball collision with bricks
for brick in bricks[:]:
if ball.colliderect(brick):
ball_speed_y *= -1
bricks.remove(brick)
break
# Game over condition
if ball.bottom >= SCREEN_HEIGHT:
running = False
# Drawing everything
screen.fill(BLACK)
for brick in bricks:
pygame.draw.rect(screen, RED, brick)
pygame.draw.rect(screen, WHITE, paddle)
pygame.draw.ellipse(screen, BLUE, ball)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Breakout Clone game will start.
This code provides a simple Breakout Clone game where the player controls a paddle at the bottom of the screen. This paddle bounces a ball and breaks bricks at the top. The game ends when the ball misses the paddle. The ball bounces off the walls, the paddle, and the bricks, with the bricks removed upon collision.
Importing libraries
Import a pygame
Import random
Pygame creates a game window, manages events, and draws.
Random sets the ball’s initial direction.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Other constants for paddle, ball, bricks, and colors
Initialize the Pygame module.
Set up constants like screen dimensions, paddle sizes, balls, bricks, and color values.
Creating the game window
Screen = pygame. Display.set_mode(SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Breakout Clone’)
Creates a game window with the specified dimensions.
Sets the window title.
Setting up the paddle, ball, and bricks
Paddle = pygame.Rect(…)
Ball = pygame.Rect(…)
Bricks = [… for x in range(10) and for y in range(5)]
Initialize the paddle, ball, and brick list.
The paddle and ball are represented as rectangles (Pygame. Rect).
Bricks are created using list comprehension to make a grid.
Ball movement initialization
Ball_speed_x = 6 * random.choice((1, -1))
Ball_speed_y = 6 * random.choice((1, -1))
Sets the ball’s initial speed and direction.
The Game Loop
While running:
# Event handling, paddle movement, ball movement…
The main loop is where game logic occurs.
Paddle movement
Key = pygame. Key.get_pressed()
If keys[pygame.K_LEFT] and paddle.left > 0:
Paddle.move_ip(-10, 0)
# Similar code for moving right
Moves the paddle left and right based on keyboard input.
Ball Movement and Collision
Ball.move_ip(ball_speed_x, ball_speed_y)
# Code to handle collisions with walls, paddles, and bricks
Updates the ball’s position.
The direction changes when the ball collides with a wall, paddle, or brick.
Ball and Brick Collision
For bricks in bricks[:]:
If ball.collider(brick):
Ball_speed_y * = -1
Bricks.remove(brick)
Break
Make sure there are no collisions between the bricks and the ball.
Removes bricks upon collision.
Game Over Condition
If ball. Bottom >= SCREEN_HEIGHT:
Running = false.
End the game if the ball misses the paddle.
Drawing on the screen
Screen.fill(BLACK)
The drawing code for bricks, paddles, and ball
Clears the screen and draws the bricks, paddle, and ball.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(60)
Update the screen with drawn graphics.
There should be 60 frames per second in the game.
Playing the game is leaving.
Pygame.quit()
It closes the game window and ends the game.
Learning Points for Students
Loops and Conditionals: The game uses loops for the primary game cycle and conditionals for game logic.
Collision detection: involves determining how the ball will move when it collides with another object.
List Comprehension: Used to create brick grids efficiently.
Game Mechanics: Having a good understanding of how game mechanics, such as movement and collision, are implemented.
Reference Links to Include:
Python Official Documentation:
- Purpose: To provide foundational Python syntax and library information.
Pygame Documentation (for game development):
- Purpose: Essential for creating the game’s graphical interface and handling user interactions, especially relevant for a Breakout clone.
GitHub Repositories for Breakout in Python:
- Purpose: To offer coding inspiration and practical examples from existing Breakout clones developed in Python.
Stack Overflow Python Game Development:
- Purpose: A valuable resource for troubleshooting common programming challenges and getting advice on game development with Python.
Leave a Reply