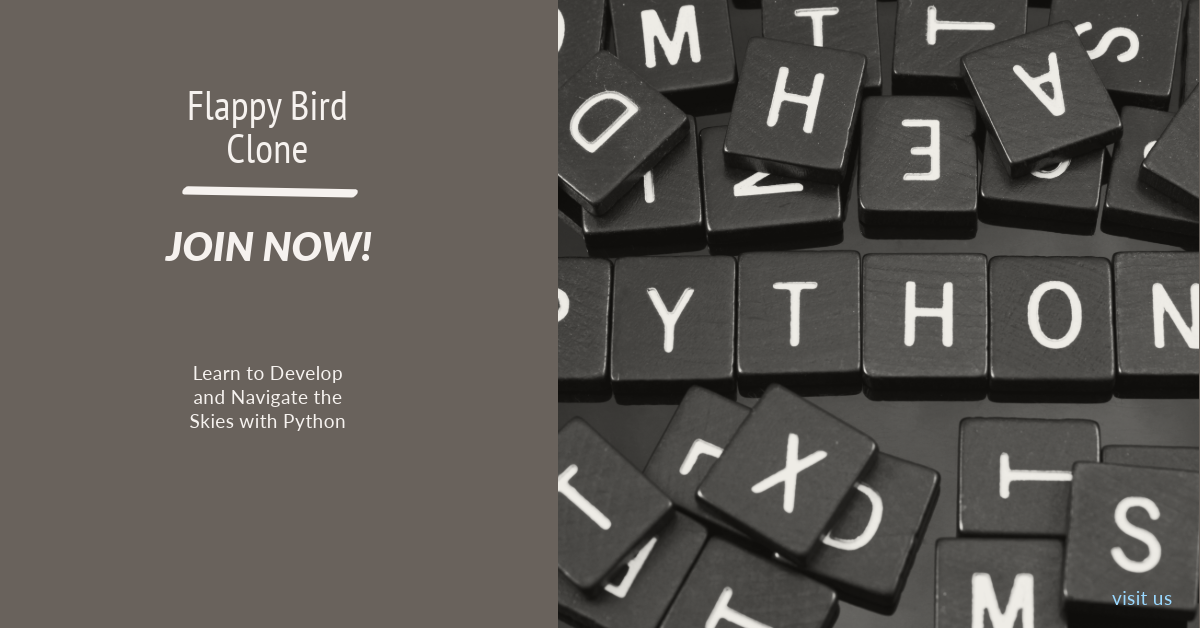
Flappy Bird Clone Game Plan:
Language: Python, suitable for demonstrating game mechanics and graphics handling.
Library: pygame, for creating the game window, handling events, and rendering graphics.
Game elements:
A bird controlled by the player.
Green pipes appear in rows, with gaps for birds to fly through.
Features:
The bird falls due to gravity and can be made to ‘flap’ upwards with a keypad.
Pipes move horizontally across the screen, and new pipes appear periodically.
Detecting collisions between the bird and the pipes.
Game over occurs when the bird hits a pipe or the ground.
User interface:
A window showing the bird, pipes, and the score.
Flappy Bird Clone Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 400, 600
BIRD_WIDTH, BIRD_HEIGHT = 30, 30
PIPE_WIDTH = 60
GAP_SIZE = 150
GRAVITY = 0.25
FLAP_STRENGTH = -5
GREEN = (0, 128, 0)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Flappy Bird Clone')
# Bird setup
bird = pygame.Rect(50, SCREEN_HEIGHT // 2, BIRD_WIDTH, BIRD_HEIGHT)
bird_movement = 0
# Pipes setup
pipes = []
pipe_frequency = 1500 # milliseconds
last_pipe = pygame.time.get_ticks()
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE:
bird_movement = FLAP_STRENGTH
# Bird movement
bird_movement += GRAVITY
bird.y += bird_movement
# Pipe creation
current_time = pygame.time.get_ticks()
if current_time - last_pipe > pipe_frequency:
pipe_height = random.randint(100, 400)
top_pipe = pygame.Rect(SCREEN_WIDTH, 0, PIPE_WIDTH, pipe_height)
bottom_pipe = pygame.Rect(SCREEN_WIDTH, pipe_height + GAP_SIZE, PIPE_WIDTH, SCREEN_HEIGHT - pipe_height - GAP_SIZE)
pipes.append((top_pipe, bottom_pipe))
last_pipe = current_time
# Pipe movement
for top_pipe, bottom_pipe in pipes[:]:
top_pipe.x -= 5
bottom_pipe.x -= 5
if top_pipe.right < 0:
pipes.remove((top_pipe, bottom_pipe))
# Collision detection
for top_pipe, bottom_pipe in pipes:
if bird.colliderect(top_pipe) or bird.colliderect(bottom_pipe) or bird.bottom >= SCREEN_HEIGHT:
running = False
# Drawing everything
screen.fill((0, 0, 0)) # Black background
pygame.draw.rect(screen, (255, 255, 0), bird) # Yellow bird
for top_pipe, bottom_pipe in pipes:
pygame.draw.rect(screen, GREEN, top_pipe)
pygame.draw.rect(screen, GREEN, bottom_pipe)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Flappy Bird Clone game will start.
This code provides a basic Flappy Bird Clone game where the player controls a bird, trying to fly between rows of green pipes. The bird falls due to gravity and can be made to ‘flap’ upwards with a key press (spacebar). Pipes move horizontally across the screen, and the game ends if the bird collides with a pipe or the ground.
Importing libraries
Import a pygame
Import random
Pygame creates a game window, handles events, and draws.
Random is used to generate pipe height.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 400, 600
# Other constants for bird size, pipe size, gravity, and colors
Initializes Pygame, which is necessary for its functionality.
Set constants like screen size, bird size, pipe size, and physical constants.
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Flappy Bird Clone’)
Creates a game window with the specified dimensions.
Sets the window title.
Setting up the birds and pipes
Bird = pygame.Rect(50, SCREEN_HEIGHT // 2, BIRD_WIDTH, BIRD_HEIGHT)
Bird_movement = 0
Pipe = []
Pipe_frequency = 1500
Last_pipe = pygame.time.get_ticks()
The bird is a rectangle representing the player’s bird.
Bird_movement controls bird vertical movement.
The pipe is a list of rectangles.
Pipe_frequency controls how often existing pipes are generated.
The Game Loop
While running:
# Event handling, bird and pipe movement, collision detection…
This loop runs continuously to update the game state and redraw the screen.
Handling player input
For the Python game events. Event.get():
If event.type == pygame.KEYDOWN:
If event.key == pygame.K_SPACE:
Bird_movement = FLAP_STRENGTH
Listens for key presses and makes the bird ‘flap’ if the spacebar is pressed.
Updating Bird and Pipe Positions
Bird_movement += GRAVITY
Bird. y += bird_movement
# Code for moving and creating pipes
It applies gravity to the bird, making it fall or rise based on flapping.
Moves the pipes across the screen and creates new pipes periodically.
Collision detection
For top_pipe and bottom_pipe in pipes:
If bird. Colliderect(top_pipe) or bird.collider(bottom_
Running = false.
Check if the bird collided with pipes or the ground.
Drawing the Game Elements
Screen.fill((0, 0, 0)) # Black background
Pygame. Draw.rect(screen, (255, 255, 0), bird) # Yellow bird
# Code for drawing pipes
He clears the screen and draws the bird and pipes.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(60)
Pygame. Display. Flip () updates the entire screen.
Clock. Tick (60) keeps the game running at 60 frames per second.
It is exciting to play the game.
Pygame.quit()
It closes the game window.
Learning Points for Students
Game Loop: The main loop handles events, updates the game state, and redraws the screen.
Physics Simulation: Basic gravity and flap mechanics to simulate bird movement.
Collision Detection: Checking for intersections between the bird and pipes.
Lists and Loops: Using lists to manage multiple objects (pipes) and loops to update/render them.
Reference Links to Include:
Python Official Documentation:
- Purpose: For foundational Python syntax and library information.
Pygame Documentation (for game development):
- Purpose: Essential for creating game graphics and handling user input, especially relevant for a Flappy Bird clone.
GitHub Repositories for Flappy Bird in Python:
- Purpose: To provide examples and inspiration from existing Flappy Bird clones developed in Python.
Stack Overflow for Python Game Development:
- Purpose: A great resource for solving programming challenges and getting advice on game development with Python.
Leave a Reply