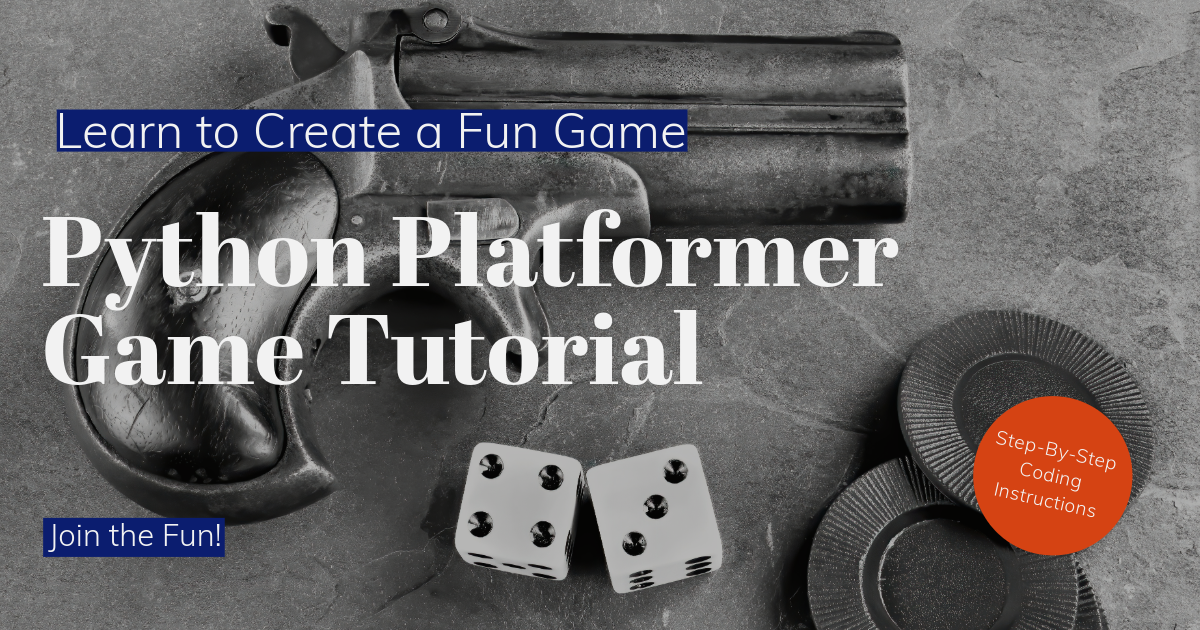
Platformer Game Plan:
Language: Python, which is excellent for game development.
Library: pygame, for creating the game window, handling events, and rendering graphics.
Game elements:
A character that the player controls.
Platforms for the character to jump on.
Enemies the character must avoid or defeat.
Collectibles that the character can gather for points or power-ups.
Features:
Character movement, including walking and jumping.
Collision detection for platforms, enemies, and collectibles.
The game world is navigated through side-scrolling mechanics.
Simple enemy behavior and interactions.
Score or point system based on collectibles and enemies.
User interface:
A window showing the game world, characters, platforms, enemies, and collectibles.
Display for score or points.
Platformer Game Code:
import pygame
import random
# Initialize Pygame
pygame.init()
# Constants
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
PLAYER_WIDTH, PLAYER_HEIGHT = 50, 50
PLATFORM_WIDTH, PLATFORM_HEIGHT = 100, 20
ENEMY_WIDTH, ENEMY_HEIGHT = 50, 50
COLLECTIBLE_SIZE = 20
GRAVITY = 0.5
JUMP_STRENGTH = -10
BLACK = (0, 0, 0)
BLUE = (0, 0, 255)
RED = (255, 0, 0)
YELLOW = (255, 255, 0)
# Screen setup
screen = pygame.display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
pygame.display.set_caption('Platformer Game')
# Player setup
player = pygame.Rect(100, SCREEN_HEIGHT - 60, PLAYER_WIDTH, PLAYER_HEIGHT)
player_velocity_y = 0
# Platforms setup
platforms = [pygame.Rect(x * 150, random.randint(100, 500), PLATFORM_WIDTH, PLATFORM_HEIGHT) for x in range(5)]
# Enemies setup
enemies = [pygame.Rect(x * 300, random.randint(100, 500), ENEMY_WIDTH, ENEMY_HEIGHT) for x in range(3)]
# Collectibles setup
collectibles = [pygame.Rect(x * 200, random.randint(100, 500), COLLECTIBLE_SIZE, COLLECTIBLE_SIZE) for x in range(5)]
# Game loop
running = True
clock = pygame.time.Clock()
while running:
# Event handling
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
if event.type == pygame.KEYDOWN:
if event.key == pygame.K_SPACE and player.bottom >= SCREEN_HEIGHT - 60:
player_velocity_y = JUMP_STRENGTH
# Player movement
player_velocity_y += GRAVITY
player.y += player_velocity_y
# Collision with platforms
for platform in platforms:
if player.colliderect(platform) and player_velocity_y > 0:
player.y = platform.top - PLAYER_HEIGHT
player_velocity_y = 0
# Collision with enemies
for enemy in enemies:
if player.colliderect(enemy):
running = False
# Collision with collectibles
for collectible in collectibles[:]:
if player.colliderect(collectible):
collectibles.remove(collectible)
# Drawing everything
screen.fill(BLACK)
pygame.draw.rect(screen, BLUE, player)
for platform in platforms:
pygame.draw.rect(screen, RED, platform)
for enemy in enemies:
pygame.draw.rect(screen, YELLOW, enemy)
for collectible in collectibles:
pygame.draw.rect(screen, (0, 255, 0), collectible)
# Update the display
pygame.display.flip()
clock.tick(60)
pygame.quit()
How to run:
Install pygame if not already installed: pip install pygame.
Save this script as a .py file.
Run the script, and the Platformer game will start.
This code provides a basic side-scrolling platformer game where the player controls a character that can jump on platforms, avoid enemies, and collect items. The game ends if the player collides with an enemy.
Importing libraries
Import a pygame
Import random
Pygame creates the game window, handles events, and draws graphics.
Randomly generated positions for platforms, enemies, and collectibles.
Initializing Pygame and Setting Constants
Pygame.init()
SCREEN_WIDTH, SCREEN_HEIGHT = 800, 600
# Other constants for player, platform, enemy, collectible sizes, and colors
Pygame. Init () starts all Pygame modules.
Constants define the game screen size, the player, platforms, enemies, collectibles, and colors.
Creating the game window
Screen = Pygame. Display.set_mode((SCREEN_WIDTH, SCREEN_HEIGHT))
Pygame. Display.set_caption(‘Platform Game’)
Sets up the game window with the specified width and height.
The caption sets the window title.
Setting Up the Player, Platforms, Enemies, and Collectibles
Player = pygame.Rect(…)
Platform = [… for x in range(5)]
Enemies = [… for x in range(3)]
Collectibles = [… for x in range(5)]
The player, platforms, enemies, and collectibles are initialized.
The player is a rectangle representing the player’s character.
Platforms, enemies, and collectibles are lists of rectangles representing game objects.
The Game Loop
While running:
# Event handling, player movement, collision detection…
The game loop is where the game logic happens. It continues as long as running is taking place.
Handling player input and movement
For Pygame events. Event.get():
# Event handling for quitting and jumping
Player_velocity_y += GRAVITY
Player. y += player_velocity_y
Processes player inputs for quitting the game and jumping.
It applies gravity to the player and updates his vertical position.
Collision detection
# Collision logic for platforms, enemies, and collectibles
Check if the player has collided with platforms (to stop falling), enemies (game over), or collectibles (to collect them).
Drawing the Game Elements
Screen.fill(BLACK)
# Drawing code for players, platforms, enemies, and collectibles
It clears the screen and draws players, platforms, enemies, and collectibles.
Update the display and frame rate.
Pygame. Display.flip()
Clock.tick(60)
Update the screen with drawn graphics.
The game runs at 60 frames per second to ensure smooth gameplay.
I find it an leave game.
Pygame.quit()
Closes the playgame window and exits the game.
Learning Points for Students
Game Loop: The central loop handles events, updates the game state, and redraws the screen.
Physics and Mechanics: Implementing gravity and jumping mechanics.
Collision Detection: Essential for interacting with platforms, enemies, and collectibles.
Lists and Loops: Using lists to manage game objects and loops to update and render them.
Reference Links to Include:
Python Official Documentation:
- Purpose: To provide foundational Python knowledge necessary for game development.
Pygame Documentation (for game mechanics and graphics):
- Purpose: Essential for implementing the game’s interactive and visual elements.
GitHub Repositories for Platformer Games in Python:
- Purpose: To offer coding inspiration and practical examples from existing platformer games developed in Python.
Stack Overflow Python Game Development:
- Purpose: A valuable resource for troubleshooting common issues and getting advice on game development with Python.
Leave a Reply