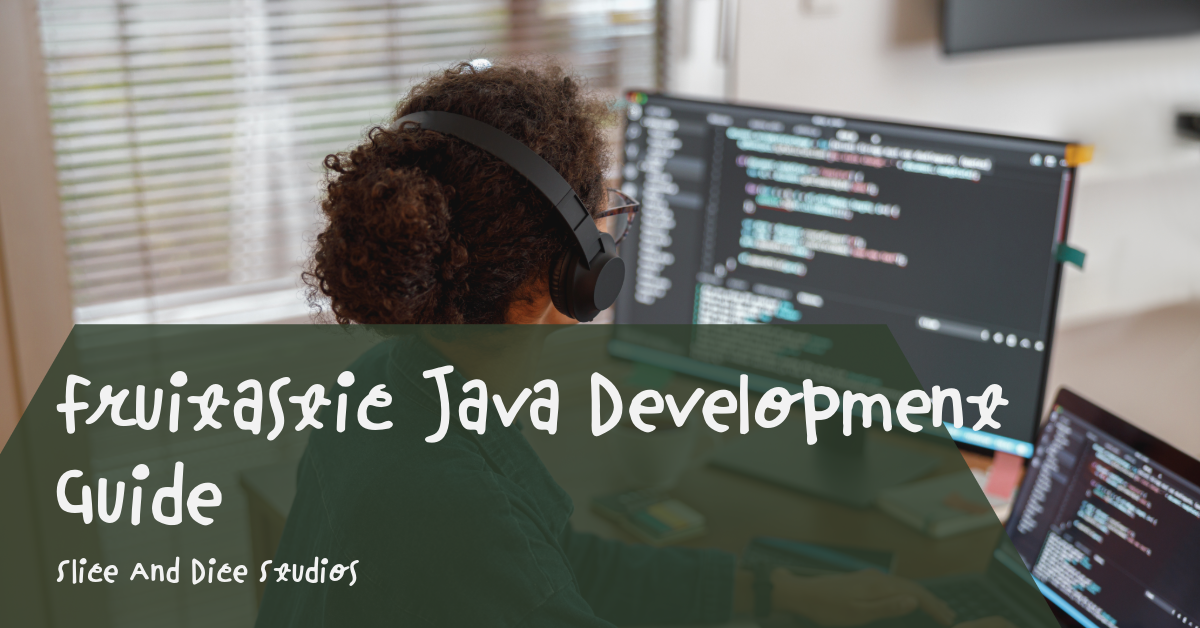
Creating a Fruit Ninja clone involves detecting swipe gestures to slice fruits and avoiding bombs. Given the complexity of swipe gestures, I’ll guide you through creating a simplified version of this game using mouse events for the web. This approach can later be adapted for touch events or a specific game development framework for mobile platforms.
Step 1: HTML Structure
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Fruit Ninja Clone</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameContainer"></div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
#gameContainer {
width: 800px;
height: 600px;
background-color: #ddf;
position: relative;
overflow: hidden;
cursor: crosshair;
}
.fruit, .bomb {
width: 50px;
height: 50px;
position: absolute;
background-size: cover;
}
.fruit { background-image: url('fruit.png'); } /* Placeholder for fruit image */
.bomb { background-image: url('bomb.png'); } /* Placeholder for bomb image */
Step 3: JavaScript Logic
In game.js
, we’ll create the basic mechanics. This includes spawning fruits and bombs, making them fall, and slicing them with mouse movements.
document.addEventListener('DOMContentLoaded', () => {
const gameContainer = document.getElementById('gameContainer');
let score = 0;
// Function to spawn fruits and bombs
function spawnObject(type) {
const object = document.createElement('div');
object.className = type;
object.style.left = `${Math.random() * (gameContainer.offsetWidth - 50)}px`; // Random position
object.style.top = `-50px`; // Start above the container
gameContainer.appendChild(object);
// Animate falling
let fallInterval = setInterval(() => {
let topPosition = parseInt(object.style.top);
if (topPosition > gameContainer.offsetHeight) clearInterval(fallInterval); // Remove if fallen
else object.style.top = `${topPosition + 4}px`;
}, 30);
// Add slicing event
object.addEventListener('mouseover', () => {
if (type === 'bomb') {
alert('Game Over!'); // Placeholder for game over
clearInterval(fallInterval);
gameContainer.innerHTML = ''; // Clear game area
} else {
score += 10;
console.log(`Score: ${score}`); // Placeholder for score keeping
}
object.remove(); // Remove fruit or bomb
});
}
// Spawn fruits and bombs at intervals
setInterval(() => spawnObject('fruit'), 2000);
setInterval(() => spawnObject('bomb'), 5000);
});
Game Mechanics Explained
- Spawning Objects: Fruits and bombs are created and fall from the top of the game container. Their
left
position is randomized, so they appear to come from different points. - Falling Animation: A simple animation effect is achieved by incrementally increasing the
top
style property, making the object move downwards. - Slicing and Game Over: When the player “slices” (hovers over) an object, an event listener triggers. If it’s a fruit, the player scores points. If it’s a bomb, the game ends.
- Scoring: Points are awarded for slicing fruits. You can extend this by updating a visible score display in the game.
Extending the Game
- Touch Events: For a more authentic experience on touch devices, replace mouse events (
mouseover
) with touch events (touchstart
,touchmove
). - Graphics: Use images or animations for fruits, bombs, and slicing effects. Adjust CSS
background-image
properties accordingly. - Gameplay Mechanics: Implement levels, time limits, or special fruits for bonus points to increase game complexity.
Summary
This basic implementation captures the essence of Fruit Ninja, focusing on object generation, basic animations, and interaction handling. It’s a foundational step towards creating a fully-featured game, with many opportunities for enhancements and customization.

Let’s break down the Fruit Ninja clone game code into simpler parts to make it easier for students to understand how each piece contributes to creating the game. We’ll focus on the game’s setup, spawning fruits and bombs, making them fall, and handling slices (interactions).
<div id="gameContainer"></div>
gameContainer
: This is where our game happens. Think of it as our game’s “arena” where fruits and bombs appear and fall down the screen.
CSS Styling
#gameContainer {
cursor: crosshair;
}
.fruit, .bomb {
width: 50px;
height: 50px;
background-size: cover;
}
cursor: crosshair;
: Changes the mouse cursor to a crosshair, making it feel like you’re aiming to slice..fruit
and.bomb
: These classes are for fruits and bombs, making them look like small squares (for now). In a real game, you’d replacebackground-image
with actual images of fruits and bombs.
JavaScript Logic
Setting Up The Game
document.addEventListener('DOMContentLoaded', () => {
const gameContainer = document.getElementById('gameContainer');
let score = 0;
...
});
- When the web page loads (
DOMContentLoaded
), we grab thegameContainer
and set up a variable to keep track of the player’s score.
Spawning Fruits and Bombs
function spawnObject(type) {
...
gameContainer.appendChild(object);
...
}
spawnObject(type)
: This function creates either a fruit or a bomb inside the game arena, depending on thetype
(‘fruit’ or ‘bomb’). It places the object at a random position at the top of the game area, ready to fall down.
Making Objects Fall
let fallInterval = setInterval(() => {
object.style.top = `${topPosition + 4}px`;
}, 30);
- Each fruit or bomb falls down the screen because we repeatedly move it a little bit lower (
topPosition + 4
) every 30 milliseconds.
Slicing Fruits and Avoiding Bombs
object.addEventListener('mouseover', () => {
if (type === 'bomb') {
alert('Game Over!');
gameContainer.innerHTML = ''; // Clear game area
} else {
score += 10;
console.log(`Score: ${score}`);
}
object.remove(); // Remove fruit or bomb
});
- When you move your mouse over (or “slice”) a fruit, you gain points. If it’s a bomb, the game shows “Game Over!” and resets.
object.remove();
: Removes the fruit or bomb from the screen after it’s sliced or triggers a game over.
Continuously Spawning Objects
setInterval(() => spawnObject('fruit'), 2000);
setInterval(() => spawnObject('bomb'), 5000);
Summary for Students
In this simple version of Fruit Ninja:
- The game area (
gameContainer
) is where all the action happens. - Fruits and bombs “spawn” at random positions at the top and then fall down the screen.
- You “slice” fruits for points by moving your cursor over them. If you hit a bomb, it’s game over.
- The game keeps spawning fruits and bombs, making you dodge and slice to increase your score.
This explanation helps break down how the game works, showing how to create interactive web games with HTML, CSS, and JavaScript. It’s a basic foundation, and there are many ways to expand and improve the game, like adding touch events for mobile devices or improving the graphics and gameplay.
Reference Links to Include:
Java Game Development Frameworks:
- Introduction to frameworks suitable for developing interactive games in Java, such as LibGDX.
- Suggested Search: “Java game development LibGDX
Implementing Touch and Swipe Mechanics in Java:
- Guides or tutorials on creating responsive touch mechanics for a game similar to Fruit Ninja.
- Suggested Search: “Java swipe mechanics for games”
Game Design Principles for Action Games:
- Insights into designing engaging and responsive action games, focusing on elements like timing and player feedback.
- Suggested Search: “Action game design principles”
GitHub Repositories for Fruit Ninja in Java:
- Examples of Fruit Ninja clones or similar projects developed in Java, offering coding inspiration and practical insights.
Stack Overflow for Java Game Development Questions:
- A platform for troubleshooting and advice on developing interactive games in Java.
Leave a Reply