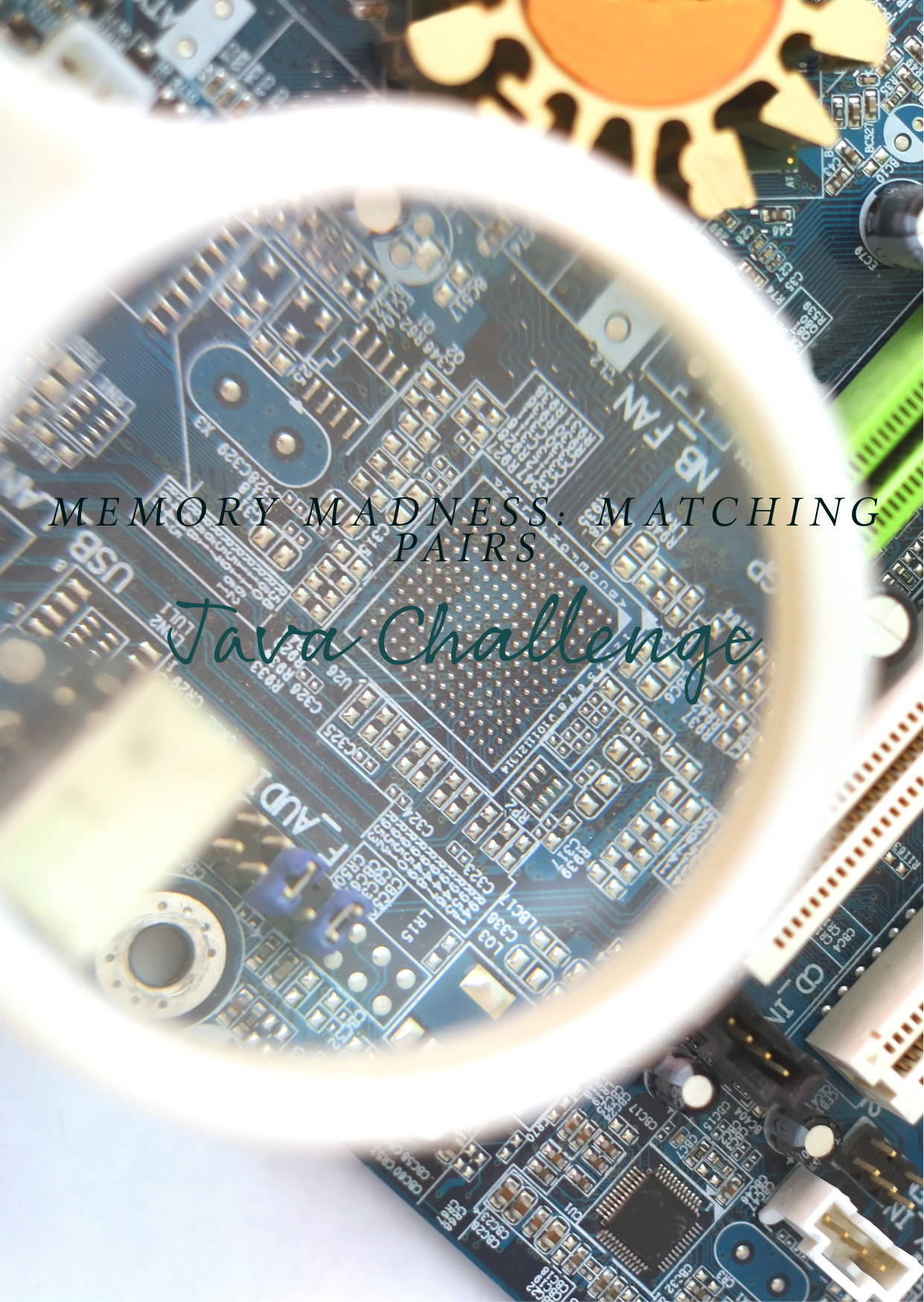
The goal of creating a Memory Card Game is for players to flip cards to find matching pairs. Practice JavaScript, HTML, and CSS through this website. The following version is designed for beginners.
Step 1: HTML Structure
Create a basic HTML file to define the game area and the cards. For simplicity, we’ll use emojis as card content.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Memory Game</title>
<link rel="stylesheet" href="style.css">
</head>
<body>
<div id="gameContainer"></div>
<script src="game.js"></script>
</body>
</html>
Step 2: CSS Styling
Add styles to give the game a basic visual structure. It includes styling the game area and the cards.
#gameContainer {
display: grid;
grid-template-columns: repeat(4, 100px);
grid-gap: 10px;
margin: 0 auto;
width: 420px;
}
.card {
width: 100px;
height: 100px;
background-color: #f0f0f0;
display: flex;
justify-content: center;
align-items: center;
font-size: 2em;
cursor: pointer;
}
Step 3: JavaScript Logic
JavaScript will handle creating the cards, flipping them, and checking for matches.
document.addEventListener('DOMContentLoaded', () => {
const gameContainer = document.getElementById('gameContainer');
const emojis = ['😀', '🎈', '🌻', '🚗', '🎉', '🏀', '🍉', '🐶'];
const cards = [...emojis, ...emojis]; // Duplicate emojis for pairs
let selectedCards = [];
let matchedPairs = 0;
// Shuffle cards
cards.sort(() => 0.5 - Math.random());
// Create cards and append to the game container
cards.forEach((emoji) => {
const cardElement = document.createElement('div');
cardElement.classList.add('card');
cardElement.dataset.emoji = emoji; // Store emoji in dataset for comparison
cardElement.addEventListener('click', () => flipCard(cardElement));
gameContainer.appendChild(cardElement);
});
function flipCard(card) {
if (selectedCards.length === 2 || card.classList.contains('matched')) {
return;
}
card.textContent = card.dataset.emoji; // Show emoji
selectedCards.push(card);
if (selectedCards.length === 2) {
checkForMatch();
}
}
function checkForMatch() {
const [firstCard, secondCard] = selectedCards;
if (firstCard.dataset.emoji === secondCard.dataset.emoji) {
firstCard.classList.add('matched');
secondCard.classList.add('matched');
matchedPairs++;
if (matchedPairs === emojis.length) {
alert('You won!');
}
} else {
setTimeout(() => {
firstCard.textContent = '';
secondCard.textContent = '';
}, 1000);
}
selectedCards = [];
}
});
Game mechanics explained
Initialization: The game starts by creating a set of cards with emojis. Each emoji appears twice to create pairs.
Shuffling: The cards are shuffled to randomize their positions on the grid.
Flipping Cards: When a player clicks on a card, it reveals the emoji. The game then waits for the player to select another card to see if it matches.
Checking for Matches: If the two selected cards match (they have the same emoji), they remain flipped. Otherwise, they flip back over after a short delay.
Winning the Game: The game is won when all pairs are correctly matched.
HTML structure
<div id="gameContainer"></div>
GameContainer: This is the play area where all cards are displayed. Our memory game board looks like this.
CSS Styling
#gameContainer {
display: grid;
}
.card {
background-color: #f0f0f0;
font-size: 2em;
}
The game container is a grid. This arrangement allows us to place cards neatly and organize them, making it easy for players to view all the cards.
Each card has a basic style, with a background color and a large font size to clearly display the card’s content (emojis) when flipped.
JavaScript Logic
Setting Up the Game
The game starts by creating a list of emojis, each appearing twice since the goal is to find matching pairs.
Cards are shuffled to ensure randomness, making it a test of memory each time you play.
Game flow
Flipping Cards: When you click on a card, the game reveals what’s on the other side (an emoji, in this case).
Matching Logic: After flipping two cards, the game checks if they match:
If they do, those cards remain flipped, signifying a successful match.
If they don’t match, they flip back over, and you’ll have to remember their positions to make a match in future turns.
Win the Game: Once all pairs are matched, you win.
Key functions
By flipping the card, you can discover the card’s emoji. For matching, it also records which cards are flipped.
CheckForMatch(): After two cards are flipped, this function checks if they match based on the emojis. If the cards match, they stay flipped. If not, they’re turned back over after a short pause.
Summary for students
In the Memory Card Game, you have a set of cards facing down. Each card has a matching pair.
Start the Game: All cards are laid out face-down in a grid.
Play the Game: You flip over two cards, trying to find two that match.
Cards that match stay face up.
If they don’t match, they flip back over, and you try again.
Goal: The aim is to remember where each card is and match all pairs as quickly as possible.
Reference Links:
- Java Game Development Resources:
- (consider LWJGL for direct control)
- (Java documentation)
- Game Design Inspiration (Memory Games)
Leave a Reply