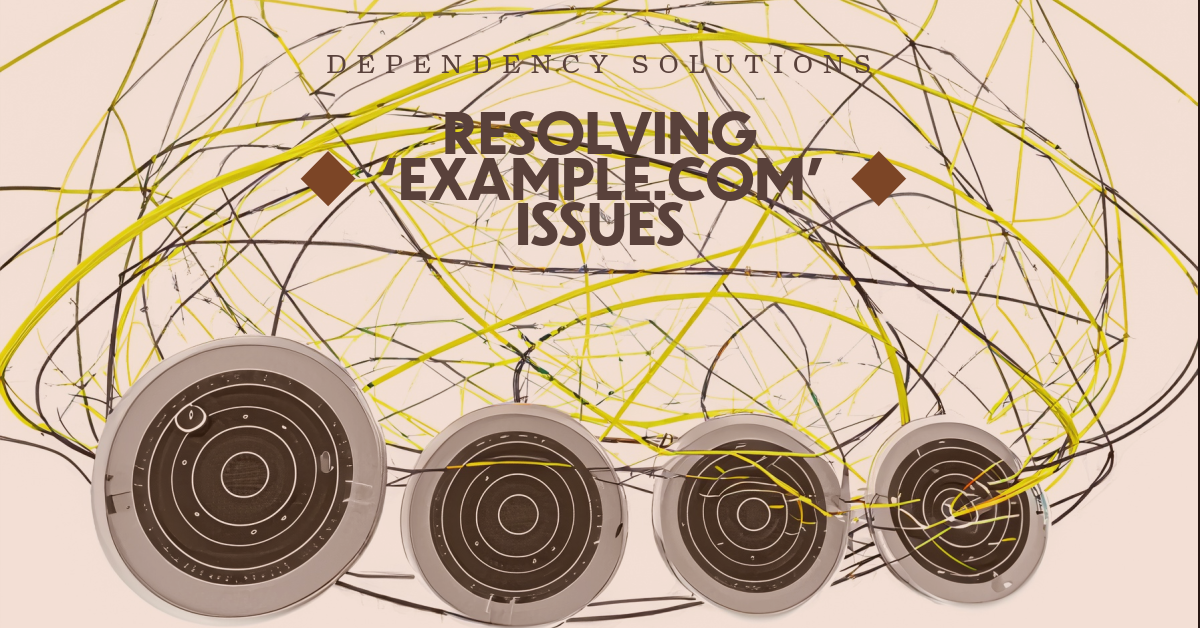
When pip
fails to install packages from a requirements.txt
file due to a supposedly missing dependency that you’ve already installed, it could be due to several reasons. Here are some steps to troubleshoot and resolve the issue, along with example code snippets for clarity.
1. Ensure the Dependency is Installed in the Same Environment
First, confirm that the dependency is installed in the same Python environment (e.g., virtual environment) as the one you’re trying to install your requirements.txt
in.
Check the currently active Python environment:
which python
or on Windows:
where python
List installed packages:
pip list
If the dependency is missing from the list, install it:
pip install <dependency-name>
2. Verify Dependency Version
Ensure that the version of the dependency you’ve installed matches or is compatible with what your requirements.txt
file or another package requires.
Example:
If requirements.txt
specifies some-package==1.2.3
, but you’ve installed some-package
version 2.0.0
, you might need to install the specific version required:
pip install some-package==1.2.3
3. Check for Environment Conflicts
If you’re using a virtual environment, make sure you’ve activated it before running pip install. If you’re not in the correct environment, you may inadvertently install packages in the wrong Python environment.
Activate your virtual environment:
On macOS/Linux:
source /path/to/venv/bin/activate
On Windows:
\path\to\venv\Scripts\activate
4. Use --force-reinstall
Option
Sometimes, forcing pip
to reinstall the packages can resolve strange dependency issues.
pip install --force-reinstall -r requirements.txt
5. Check for System-Level Dependencies
Some Python packages require system-level dependencies that cannot be installed by pip
. Ensure all such dependencies are installed. This is common with packages that have C extensions or are wrappers around C libraries.
For example, if you’re trying to install Pillow
(a Python Imaging Library), you might need system packages like libjpeg
and zlib
.
Install system dependencies (example for Ubuntu/Debian):
sudo apt-get install libjpeg-dev zlib1g-dev
Then, try installing your Python packages again:
pip install -r requirements.txt
6. Use Verbose Mode to Identify the Issue
Running pip install
in verbose mode (-v
) can help identify exactly why the installation is failing.
pip install -r requirements.txt -v
Look through the output for errors or warnings that might indicate the problem.
Example Troubleshooting Session:
# Activate virtual environment
source /path/to/venv/bin/activate
# Check if the dependency is installed
pip list | grep some-package
# If missing, install it
pip install some-package==1.2.3
# Try installing requirements again
pip install -r requirements.txt
# If it fails, use verbose mode to investigate
pip install -r requirements.txt -v
These steps should help diagnose and solve most issues related to pip
not installing packages due to missing dependencies. If you continue to have trouble, consider checking the package documentation for any known issues or specific installation instructions.
Reference Links to Include:
- For official guidelines and troubleshooting tips related to pip and dependency management.
Python Packaging Authority (PyPA) Troubleshooting:
- Offers insights into common issues and solutions regarding Python packages and dependencies.
- Suggested Search: “PyPA troubleshooting dependency issues”
GitHub Repositories with ‘requirements.txt’ Examples:
- For practical examples of well-structured ‘requirements.txt’ files in various Python projects.
Stack Overflow for pip and Dependency Questions:
- A platform for troubleshooting and advice on resolving pip dependency issues.
Leave a Reply