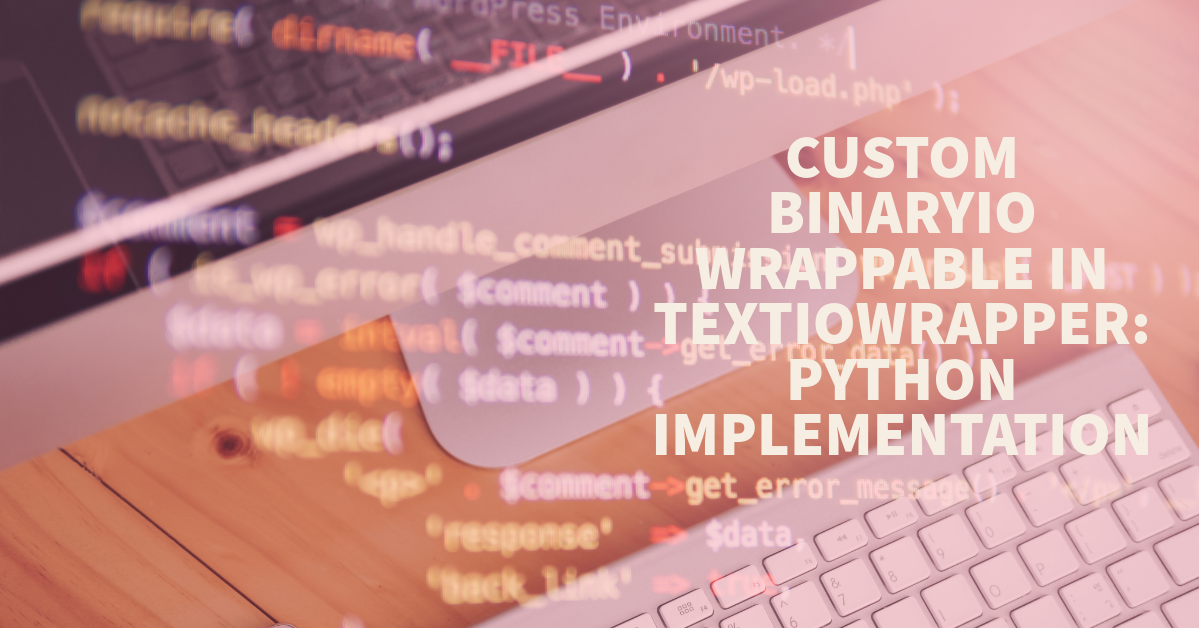
To implement a custom BinaryIO
that can be wrapped in a TextIOWrapper
, you need to ensure your custom class adheres to the BinaryIO
interface defined in the io
module. This interface includes methods for reading and writing bytes, seeking within the stream, and querying the stream’s current position, among others.
After creating a custom BinaryIO
class, you can then wrap it in a TextIOWrapper
to handle encoding and decoding of text, allowing you to read and write string data to a binary stream.
Here’s a step-by-step guide to implementing a simple custom BinaryIO
class and wrapping it in a TextIOWrapper
:
Step 1: Implement the Custom BinaryIO
Class
You must at least implement the methods read()
, write()
, seek()
, tell()
, and close()
for basic functionality. For simplicity, this example will focus on an in-memory bytes buffer, but you could adapt it to work with files, network streams, etc.
from io import BufferedIOBase
class CustomBinaryIO(BufferedIOBase):
def __init__(self):
self.buffer = bytearray()
self.position = 0
def read(self, size=-1):
if size == -1:
size = len(self.buffer) - self.position
data = self.buffer[self.position:self.position + size]
self.position += size
return bytes(data)
def write(self, b):
if isinstance(b, bytes):
end_position = self.position + len(b)
self.buffer[self.position:end_position] = b
self.position = end_position
return len(b)
raise TypeError("a bytes-like object is required, not '{}'".format(type(b).__name__))
def seek(self, offset, whence=0):
if whence == 0: # Absolute file positioning
self.position = offset
elif whence == 1: # Seek relative to current position
self.position += offset
elif whence == 2: # Seek relative to file's end
self.position = len(self.buffer) + offset
return self.position
def tell(self):
return self.position
def close(self):
pass # Implement resource release logic here if needed
def readable(self):
return True
def writable(self):
return True
def seekable(self):
return True
Step 2: Wrap the Custom BinaryIO
in a TextIOWrapper
Once you have a custom BinaryIO
implementation, you can wrap it in a TextIOWrapper
to work with text data. The TextIOWrapper
requires a binary stream (like the one your custom class provides) and handles encoding and decoding of text.
import io
# Initialize the custom binary IO
binary_stream = CustomBinaryIO()
# Wrap the binary stream in a TextIOWrapper for text handling
text_stream = io.TextIOWrapper(binary_stream, encoding='utf-8')
# Example usage
text_stream.write('Hello, world!')
text_stream.seek(0)
print(text_stream.read()) # Output: Hello, world!
text_stream.close() # Don't forget to close the stream when done
This example demonstrates a basic custom BinaryIO
implementation that can be used with TextIOWrapper
. Depending on your specific needs, you might need to implement additional methods or handle more complex scenarios, such as error handling, buffering, or concurrency.
Reference Links to Include:
Python Official Documentation:
- For foundational concepts on IO in Python, including BinaryIO and TextIOWrapper.
- Detailed guides or tutorials on creating custom IO classes in Python.
- Suggested Search: “Creating custom IO streams Python”
GitHub Repositories Featuring Custom IO Implementations:
- Examples of custom BinaryIO and TextIOWrapper implementations in Python projects.
Stack Overflow for Python IO Questions:
- A platform for troubleshooting and advice on Python IO, including custom implementations.
Leave a Reply