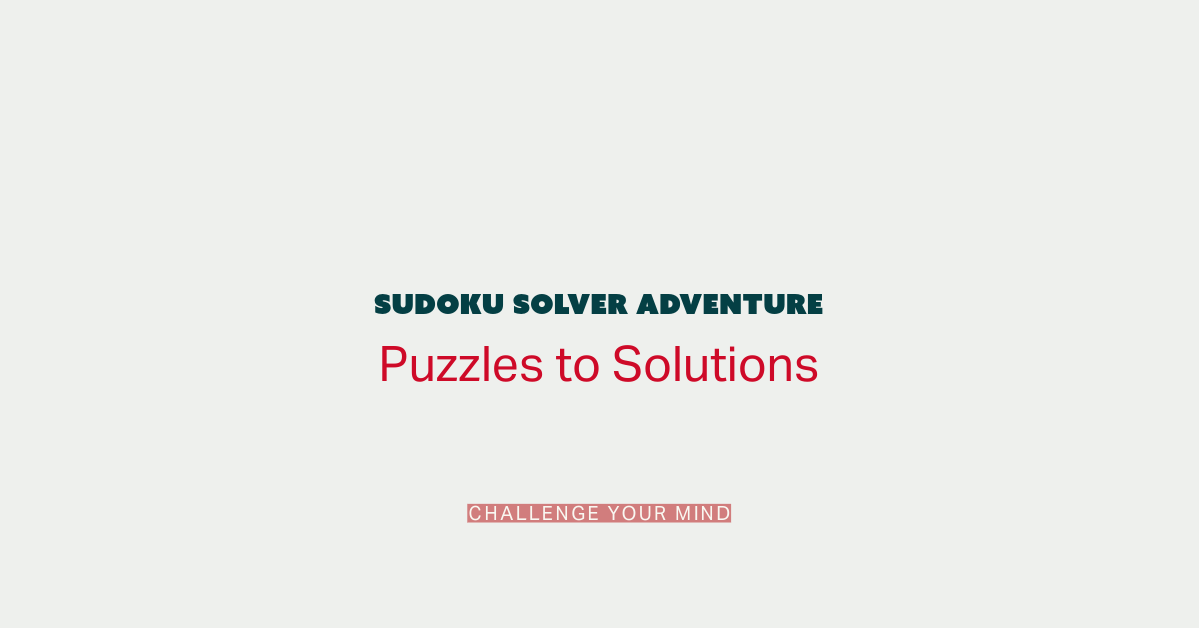
Sudoku Solver Algorithm Plan:
Language: Python is ideal for creating algorithms and handling data structures like grids.
Concept: Create a function that inputs a Sudoku puzzle and returns the solved puzzle.
Algorithm:
Use backtracking, a form of recursion, to fill in the Sudoku grid.
Check each row, column, and 3×3 square for valid numbers.
Place a number and move to the next cell if it is valid.
If no number is valid, proceed to the previous cell and try a different number.
Validation: Ensure that the numbers are placed according to Sudoku rules.
Sudoku Solver Algorithm Code:
def is_valid(board, row, col, num):
# Check if a number can be placed in a specific position
for x in range(9):
if board[row][x] == num or board[x][col] == num:
return False
start_row, start_col = 3 * (row // 3), 3 * (col // 3)
for i in range(3):
for j in range(3):
if board[start_row + i][start_col + j] == num:
return False
return True
def solve_sudoku(board):
for row in range(9):
for col in range(9):
if board[row][col] == 0:
for num in range(1, 10):
if is_valid(board, row, col, num):
board[row][col] = num
if solve_sudoku(board):
return True
board[row][col] = 0
return False
return True
# Example Sudoku Puzzle
board = [
[5, 3, 0, 0, 7, 0, 0, 0, 0],
[6, 0, 0, 1, 9, 5, 0, 0, 0],
[0, 9, 8, 0, 0, 0, 0, 6, 0],
[8, 0, 0, 0, 6, 0, 0, 0, 3],
[4, 0, 0, 8, 0, 3, 0, 0, 1],
[7, 0, 0, 0, 2, 0, 0, 0, 6],
[0, 6, 0, 0, 0, 0, 2, 8, 0],
[0, 0, 0, 4, 1, 9, 0, 0, 5],
[0, 0, 0, 0, 8, 0, 0, 7, 9]
]
if solve_sudoku(board):
for row in board:
print(row)
else:
print("No solution exists")
How to run:
Save this script as a .py file.
Run the script using Python. The script will solve the provided Sudoku puzzle and print the solution.
This code solves a Sudoku puzzle using a back-tracking algorithm. It iteratively fills the board with numbers, checks if the current board state is valid, and backtracks when it reaches a dead end. If the puzzle can be solved, it prints the solvable board; otherwise, it states that no solution exists.
Function for validating numbers
Def is_valid(board, row, col, num):
# Code to check if a number can be placed in a specific position
This function checks whether a number (num) can be placed in a given row and column on the Sudoku board.
It checks three conditions:
The number is already in a different row.
The number is already in a different column.
The number is already in a different 3×3 grid.
The number can be placed if all conditions are met (returns True). Otherwise, it cannot be (returns False).
Function to Solve Sudoku
Def solve_sudoku(board):
# Code to solve the Sudoku puzzle using backtracking
This function solves the entire Sudoku puzzle.
It passes through each grid cell:
If a cell is empty (marked as 0), it tries to fill it with a valid number (1-9).
If a valid number is found, the function is called recursively to solve the rest of the board.
If no valid number can be found for a cell, it resets the cell to 0 and backtracks (tries a different number in the previous cell).
Trying to Solve a Sample Puzzle
Board = […]
The board is a 2D list representing a Sudoku puzzle. Empty cells are represented by 0.
Running the Solver
If solve_sudoku(board):
# Print the solved board
Else:
# Print that no solution exists
This part of the code attempts to solve the provided puzzle.
If solve_sudoku returns True, the puzzle is solved, and the solved board is printed.
If it returns False, the puzzle can’t be solved, and a message is printed.
Learning Points for Students
Recursion and Backtracking: The algorithm solves the puzzle using recursion (a function calling itself) and backtracking (undoing previous steps).
Nested Loops: The code uses loops within loops to go through the rows, columns, and 3×3 grids of the Sudoku puzzle.
Problem-Solving: Demonstrates a method to approach and solve a complex problem in steps.
Boolean Logic: Uses True/False values to control program flow.
Reference Links to Include:
Python Official Documentation:
- Purpose: Essential for readers to consult Python’s syntax and libraries.
Pygame for Python Game Development:
- Purpose: If your tutorial includes building a Sudoku game interface, Pygame documentation can help readers with graphical elements.
GitHub Repositories for Sudoku Solver:
- Purpose: Provides examples of Sudoku solvers coded in Python, which can offer inspiration and practical coding insights.
Stack Overflow for Python Programming:
- Purpose: A resource for troubleshooting and exploring community solutions to programming challenges encountered while developing the Sudoku solver and game.
Leave a Reply