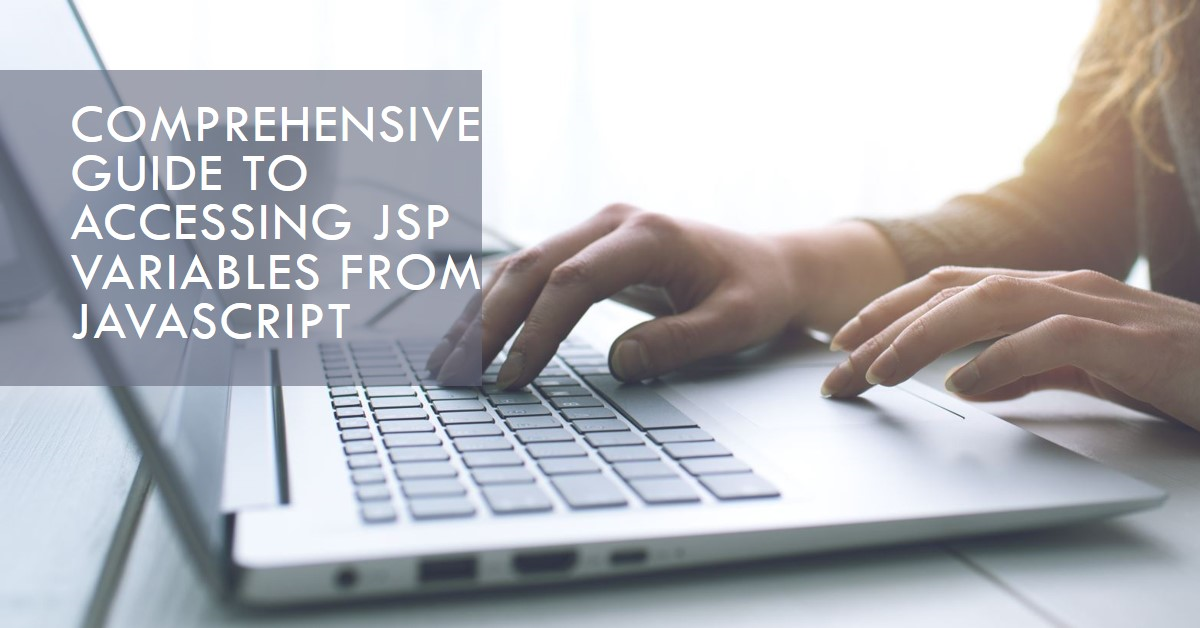
A JSP (JavaServer Pages) variable is read using JavaScript, which passes data from the server-side JSP environment to the client-side JavaScript. Since JSP is executed on the server and JavaScript runs on the client, you need to embed the JSP variable into HTML so that JavaScript can access it. Here’s how to achieve this:
Embedding JSP Variables into JavaScript
<script>
var myJsVar = "<%= jspVariable %>";
console.log(myJsVar); // Outputs the value of jspVariable
</script>
Here, JSPVariable is a variable defined in your JSP file. The <%= %> syntax outputs the value of a JSP expression directly into HTML output. Ensure that jspVariable is escaped correctly to prevent XSS (Cross-Site Scripting) vulnerabilities, especially if it includes user input.
Using HTML Data Attributes: If you prefer not to mix JavaScript and JSP code directly, you can use HTML data attributes to store the JSP variable’s value, which JavaScript can then read.
<div id="myData" data-jsp-variable="<%= jspVariable %>"></div>
In JavaScript:
var myJsVar = document.getElementById("myData").getAttribute("data-jsp-variable");
console.log(myJsVar);
This method keeps your JavaScript code separate from your JSP code. This makes it cleaner and easier to maintain, especially for larger scripts or external JavaScript files.
Considerations for Complex Data
For complex data types (e.g., objects or arrays), you can use JSON to pass data from JSP to JavaScript:
Convert to JSON in JSP: First, convert your complex data into a JSON string in your JSP file. Use a library like Jackson or Gson in a servlet to prepare this JSON string.
<%
String jsonData = "{\"key\":\"value\"}"; // This could be dynamically generated
%>
Embed the JSON String: Next, embed the JSON string similarly to the direct embedding method.
<script>
var myJsObject = JSON.parse('<%= jsonData %>');
console.log(myJsObject.key); // Outputs: value
</script>
Ensure you handle JSON strings carefully to avoid syntax errors in your JavaScript code, especially with quotation marks and special characters. Using escape functions where necessary can mitigate these issues.
Security considerations
Escaping Data: Always properly escape data passed from JSP to JavaScript to prevent XSS attacks. It is essential if the data contains user input or other untrusted content.
Content Security Policy (CSP): Implementing a CSP can help mitigate the risk of XSS and other vulnerabilities by controlling the sources from which scripts can be executed.
By following these methods and considerations, you can read JSP variables in JavaScript securely and efficiently.
For reference links and further reading, while I can’t browse the internet to provide live links, I recommend looking into:
- Official Oracle JavaServer Pages Documentation for foundational knowledge on JSP.
- Mozilla Developer Network (MDN) Web Docs on JavaScript for best practices and advanced techniques in JavaScript.
- Stack Overflow for community-driven discussions, solutions, and examples of accessing JSP variables from JavaScript.
Leave a Reply